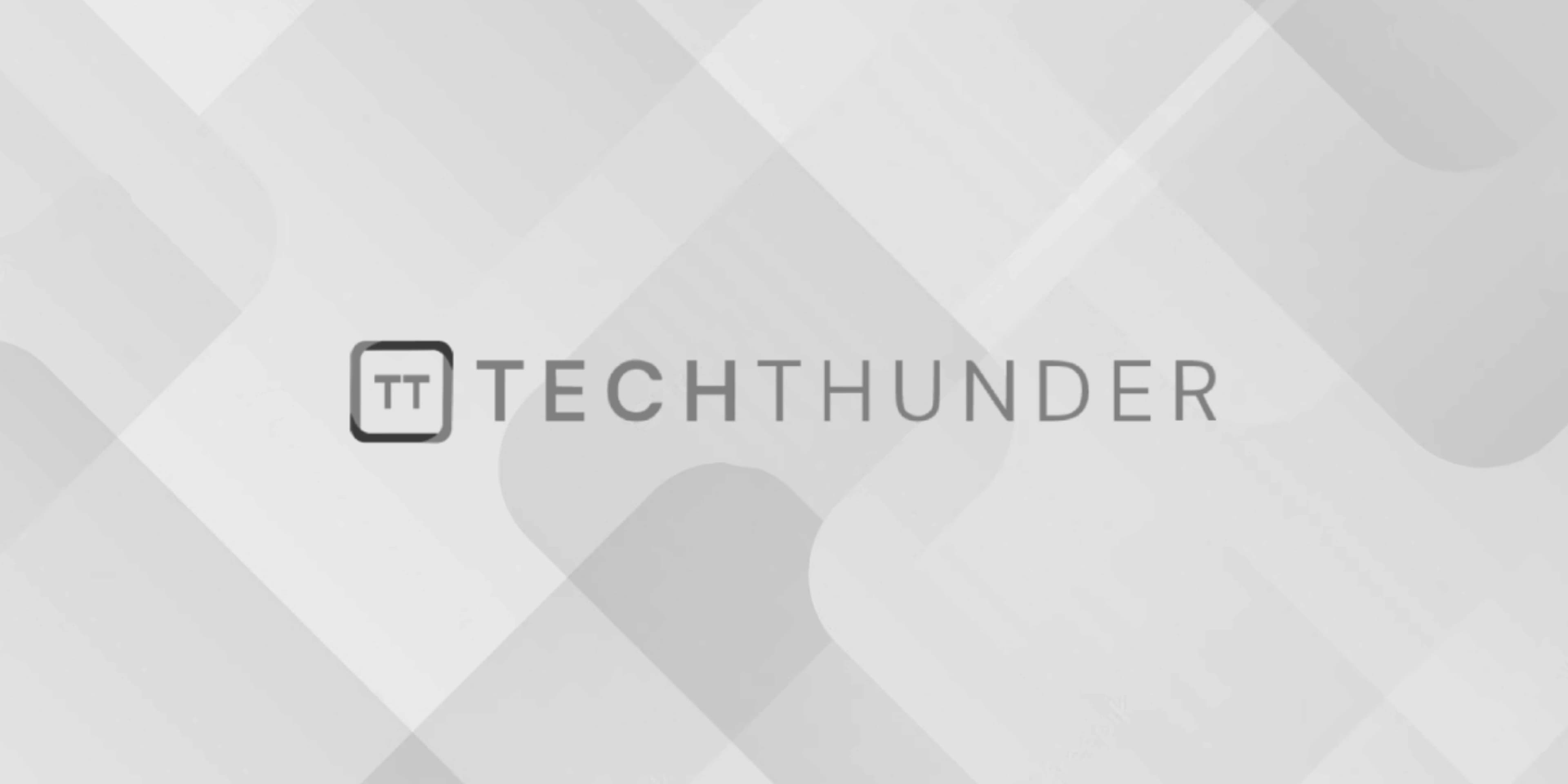
242 views
C String Functions
The string functions are part of the standard C library and are declared in the <string.h>
header. These functions provide various operations for working with strings, such as copying, concatenating, comparing, and searching within strings. Below are some commonly used C string functions:
strlen()
:
- Function: Calculates the length (number of characters) of a string.
- Prototype:
size_t strlen(const char *str);
strcpy()
:
- Function: Copies one string to another.
- Prototype:
char *strcpy(char *dest, const char *src);
strncpy()
:
- Function: Copies a specified number of characters from one string to another.
- Prototype:
char *strncpy(char *dest, const char *src, size_t n);
strcat()
:
- Function: Concatenates (appends) one string to the end of another.
- Prototype:
char *strcat(char *dest, const char *src);
strncat()
:
- Function: Concatenates a specified number of characters from one string to the end of another.
- Prototype:
char *strncat(char *dest, const char *src, size_t n);
strcmp()
:
- Function: Compares two strings lexicographically.
- Prototype:
int strcmp(const char *str1, const char *str2);
strncmp()
:
- Function: Compares a specified number of characters of two strings.
- Prototype:
int strncmp(const char *str1, const char *str2, size_t n);
strchr()
:
- Function: Finds the first occurrence of a specified character in a string.
- Prototype:
char *strchr(const char *str, int c);
strrchr()
:
- Function: Finds the last occurrence of a specified character in a string.
- Prototype:
char *strrchr(const char *str, int c);
strstr()
:- Function: Finds the first occurrence of a substring in a string.
- Prototype:
char *strstr(const char *haystack, const char *needle);
strtok()
:- Function: Breaks a string into tokens based on a delimiter.
- Prototype:
char *strtok(char *str, const char *delimiters);
strspn()
:- Function: Calculates the length of the initial substring of a string that consists of characters from a specified set.
- Prototype:
size_t strspn(const char *str, const char *accept);
strcspn()
:- Function: Calculates the length of the initial substring of a string that does not contain any characters from a specified set.
- Prototype:
size_t strcspn(const char *str, const char *reject);
strcpy_s()
andstrncpy_s()
:- These are safer versions of
strcpy()
andstrncpy()
that include buffer size checks to avoid buffer overflows. They are part of the C11 standard, not the C standard library.
- These are safer versions of
strlwr()
andstrupr()
:- These functions are not part of the standard C library but are sometimes available in some implementations to convert strings to lowercase and uppercase, respectively. However, they are not portable and may not be safe to use.
When using these functions, be careful to ensure that your strings are null-terminated (i.e., they have a ‘\0’ character at the end), and that you handle the memory and buffer sizes correctly to avoid buffer overflows and memory corruption.