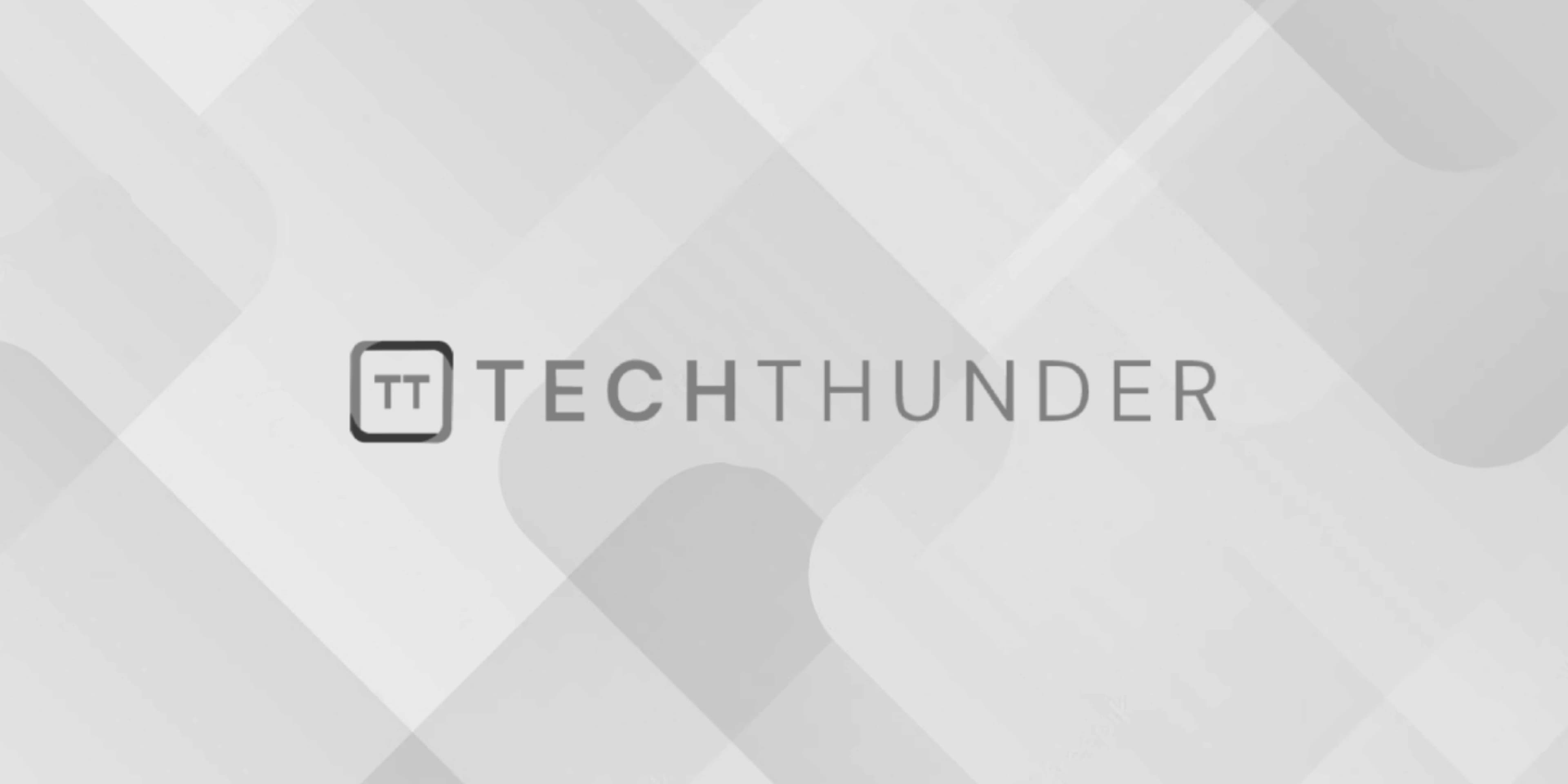
C if-else
The if-else
statement is a fundamental control flow construct that allows you to make decisions and execute different blocks of code based on the evaluation of a condition. It’s used to control the flow of your program by specifying what actions should be taken depending on whether a condition is true or false.
Here’s the basic syntax of the if-else
statement:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
condition
: An expression that is evaluated as either true (non-zero) or false (zero).- The code block inside the first set of curly braces
{}
is executed if the condition is true. - The code block inside the second set of curly braces
{}
(after theelse
keyword) is executed if the condition is false.
You can also use multiple if-else
statements together to create more complex decision structures:
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition2 is true (and condition1 is false)
} else {
// Code to execute if neither condition1 nor condition2 is true
}
Here’s an example that uses if-else
to check if a number is positive, negative, or zero:
#include <stdio.h>
int main() {
int num = 5;
if (num > 0) {
printf("The number is positive.\n");
} else if (num < 0) {
printf("The number is negative.\n");
} else {
printf("The number is zero.\n");
}
return 0;
}
In this example:
- The condition
num > 0
is evaluated first. If it’s true, the message “The number is positive.” is printed. - If the first condition is false, the next condition
num < 0
is evaluated. If it’s true (and the first condition is false), the message “The number is negative.” is printed. - If neither of the conditions is true, the code inside the
else
block is executed, and the message “The number is zero.” is printed.
Some key points to remember when using if-else
statements:
- You can nest
if-else
statements within each other to create more complex decision structures. - The code block inside each
if
orelse
statement can contain single statements or compound statements (blocks of code enclosed in curly braces). - The
else
part is optional. You can have anif
statement without anelse
if you only want to take action when a condition is true.
The if-else
statement is a fundamental building block for creating conditional logic in C programs and is used extensively for making decisions based on the evaluation of conditions.