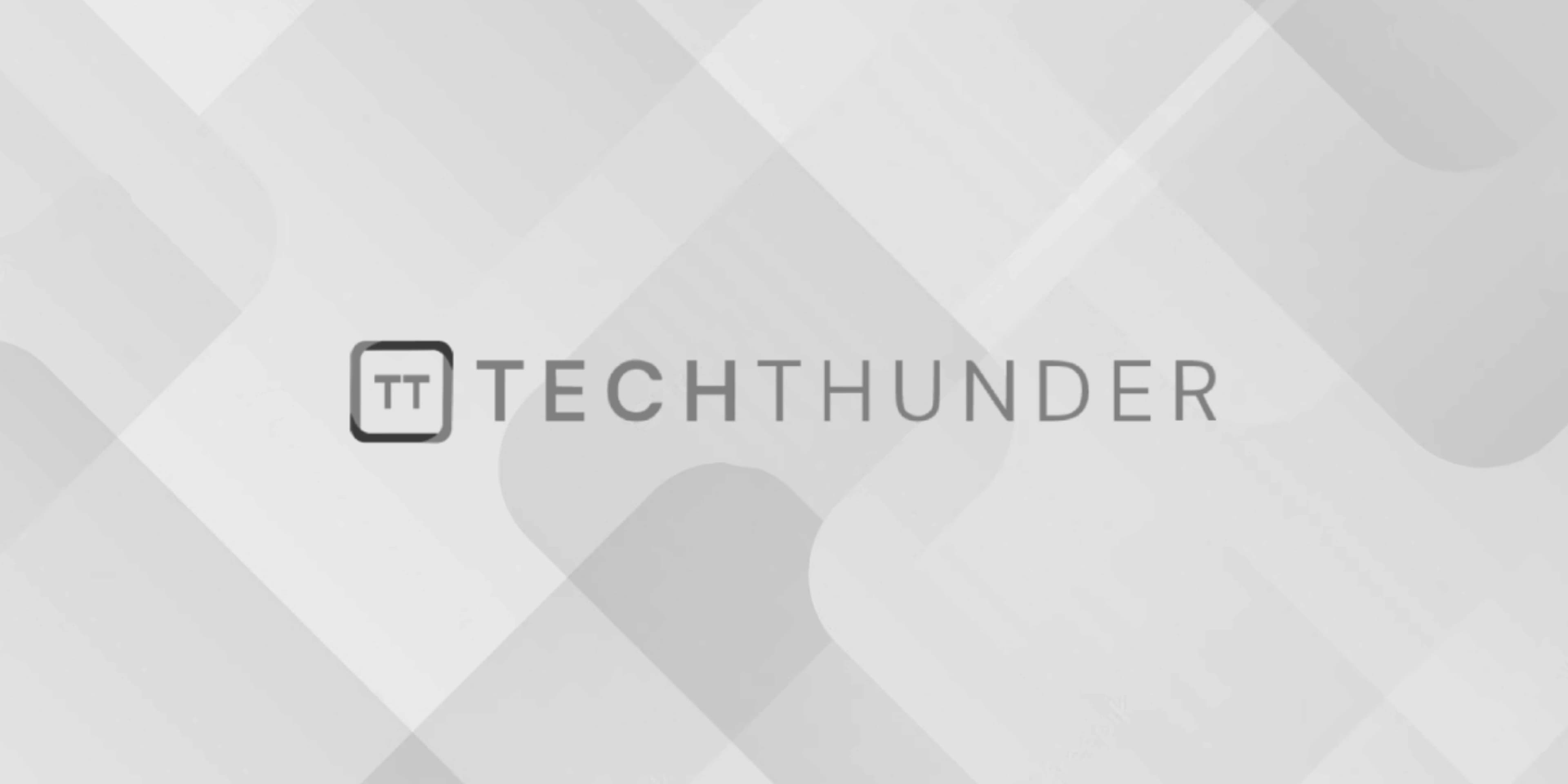
83 views
Reverse a String in C
To reverse a string in C, you can write a simple program that swaps characters from the beginning and end of the string until you reach the middle of the string. Here’s an example of how to reverse a string in C:
C
#include <stdio.h>
#include <string.h>
// Function to reverse a string
void reverseString(char *str) {
int length = strlen(str);
int start = 0;
int end = length - 1;
while (start < end) {
// Swap characters at start and end positions
char temp = str[start];
str[start] = str[end];
str[end] = temp;
// Move the start and end pointers inward
start++;
end--;
}
}
int main() {
char str[] = "Hello, World!";
printf("Original string: %s\n", str);
// Reverse the string
reverseString(str);
printf("Reversed string: %s\n", str);
return 0;
}
In this program:
- We include the
<stdio.h>
and<string.h>
headers for input/output and string manipulation functions. - We define a function
reverseString
that takes a character array (char*
) as its argument. - Inside the
reverseString
function, we use awhile
loop to swap characters from the beginning and end of the string until thestart
pointer is less than theend
pointer. - The
strlen()
function is used to find the length of the input string. - We use a temporary variable
temp
to perform the character swap. - In the
main
function, we declare an input stringstr
, print it, call thereverseString
function to reverse it, and then print the reversed string.
When you run this program, it will output:
Plaintext
Original string: Hello, World!
Reversed string: !dlroW ,olleH
The reverseString
function modifies the input string in place, reversing it without requiring additional memory for a new string.