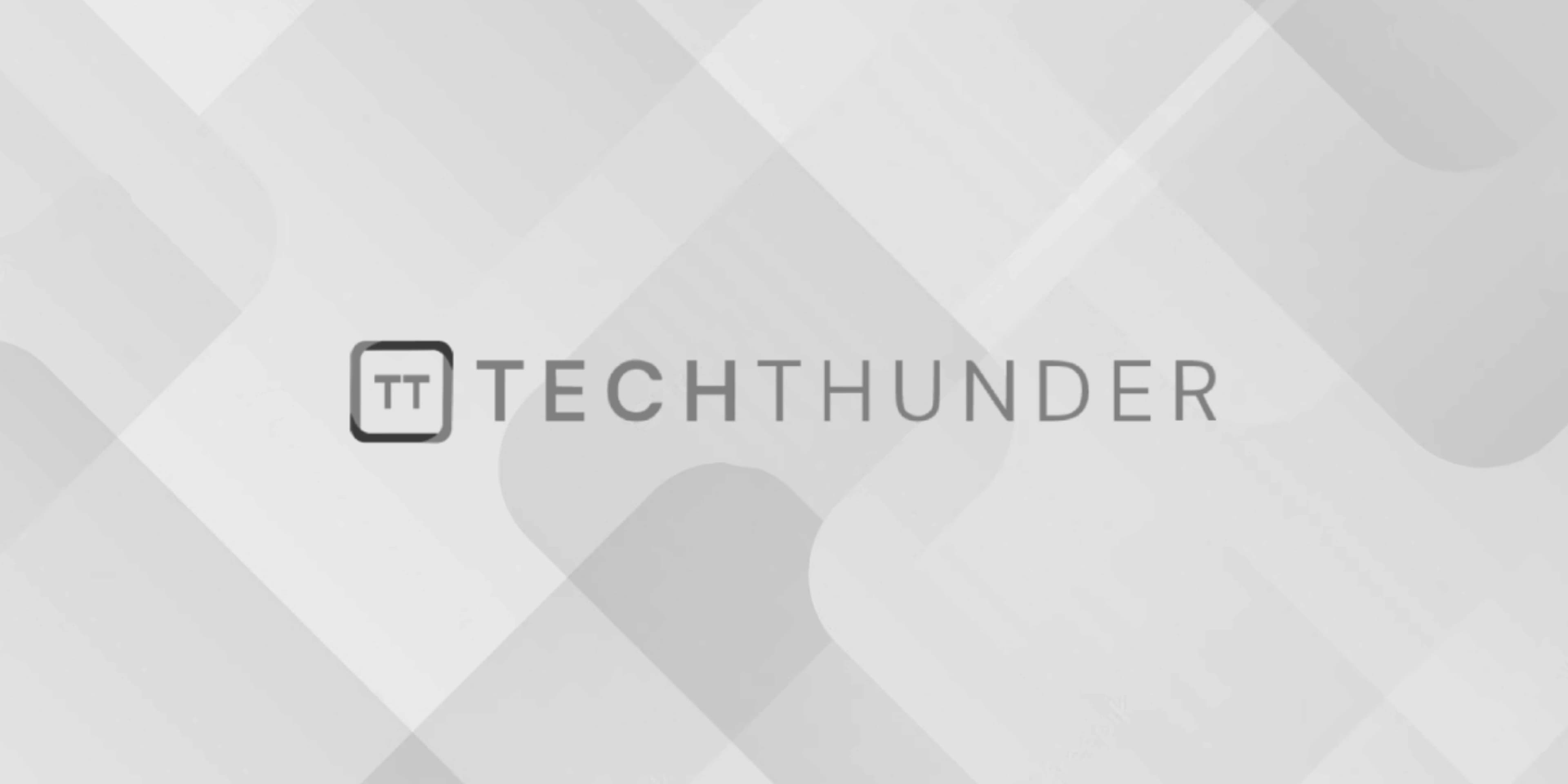
229 views
C Identifiers
The C programming, identifiers are names given to various program elements, such as variables, functions, arrays, structures, and labels. Identifiers are used to uniquely identify these elements within a program. Here are some rules and conventions for naming identifiers in C:
Rules for Identifiers:
- Character Set:
- Identifiers must consist of letters (both uppercase and lowercase), digits, and underscore
_
characters. - The first character of an identifier must be a letter (uppercase or lowercase) or an underscore.
- Length:
- Identifiers can be of any length, but only the first 31 characters are significant. Most C compilers treat only the first 31 characters of an identifier as distinct.
- Case Sensitivity:
- C is case-sensitive, meaning that uppercase and lowercase letters are considered distinct.
- For example,
variableName
andvariablename
are treated as two different identifiers.
Conventions for Identifiers:
- Descriptive Names:
- Use meaningful and descriptive names for your identifiers to improve code readability.
- Choose names that reflect the purpose or usage of the element.
- Camel Case:
- For variable names and function names, it is common to use camel case.
- Camel case involves starting with a lowercase letter and capitalizing the first letter of each subsequent word without spaces.
- Example:
myVariableName
,calculateInterestRate
.
- Underscores:
- Some programmers prefer using underscores to separate words in identifiers, especially when camel case may not be suitable.
- Example:
user_name
,maximum_value
.
- Uppercase for Constants:
- By convention, constants (variables whose values do not change) are often written in all uppercase letters.
- Example:
PI
,MAX_SIZE
.
- Avoid Reserved Words:
- Do not use C keywords or reserved words (e.g.,
int
,while
,return
) as identifiers.
- Consistency:
- Maintain consistency in your naming conventions throughout your codebase.
Examples of Valid Identifiers:
myVariable
totalSum
is_valid
MAX_ELEMENTS
calculateArea
_privateVariable
Examples of Invalid Identifiers:
123abc
(starts with a digit)my-variable
(contains a hyphen, which is not allowed)if
(a reserved word)thisIsAReallyLongIdentifierThatExceedsThe31CharacterLimit
(exceeds the significant character limit)
It’s important to follow these rules and conventions when naming identifiers in C to ensure code clarity and maintainability. Well-chosen identifiers make your code more readable and understandable by both you and other programmers who may work on the code in the future.