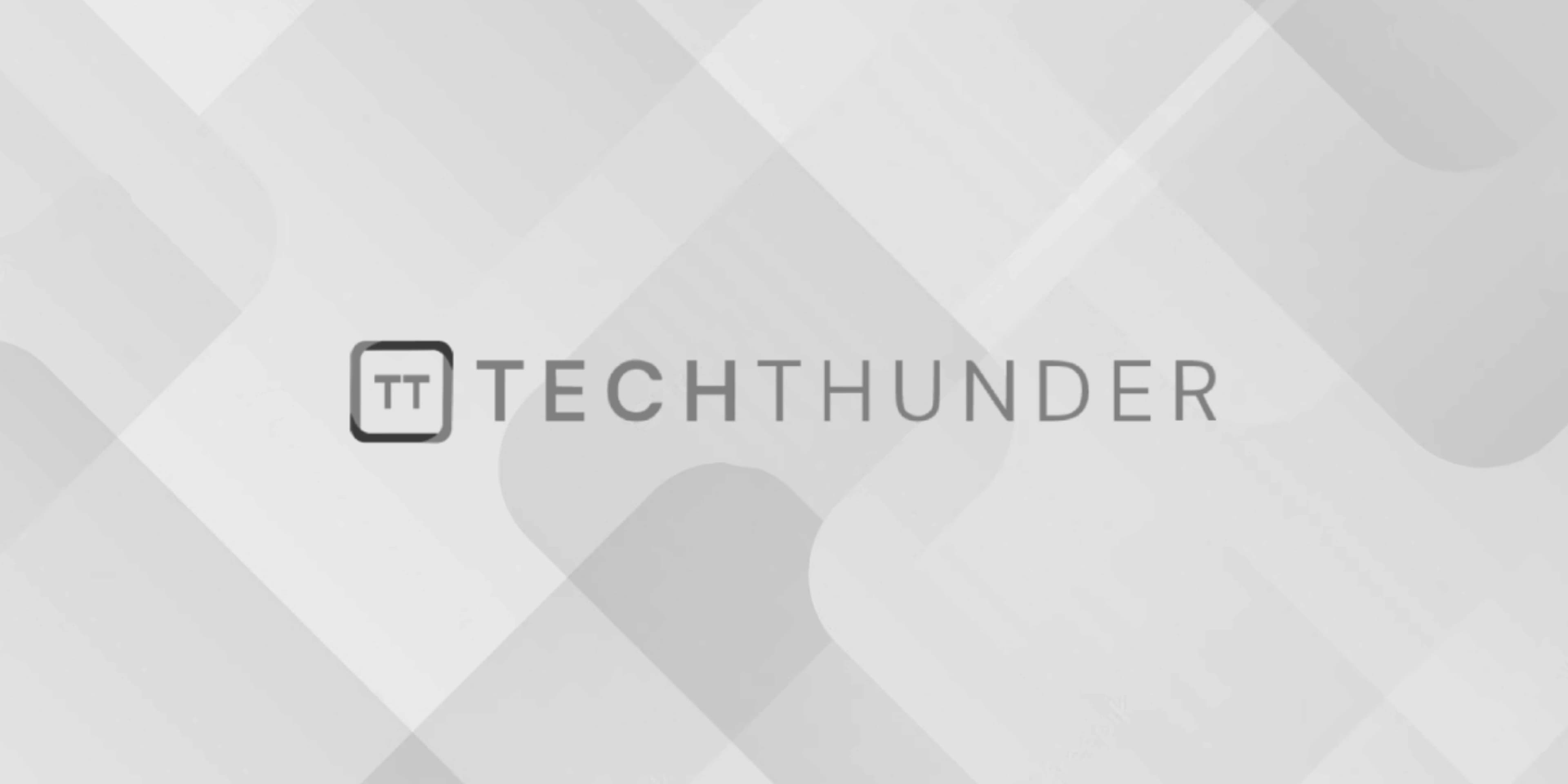
snprintf() function in C
The snprintf()
function in C is used for formatted string output to a character array while limiting the number of characters written to prevent buffer overflow. It is similar to the printf()
function for formatting, but instead of printing the formatted output to the standard output (usually the console), snprintf()
writes the output to a character array.
The prototype of snprintf()
is as follows:
int snprintf(char *str, size_t size, const char *format, ...);
str
: A pointer to the character array where the formatted output will be stored.size
: The maximum number of characters to be written tostr
, including the null-terminator. This parameter prevents buffer overflow.format
: A format string that specifies the formatting of the output, similar to the format string used inprintf()
....
: Additional arguments corresponding to the format specifiers in the format string.
The snprintf()
function returns the number of characters that would have been written to the str
if there were no size constraints (excluding the null-terminator). It does not write more than size
characters, and if the output exceeds this limit, it is truncated to fit within size - 1
characters, leaving room for the null-terminator.
Here’s a simple example of how to use snprintf()
:
#include <stdio.h>
#include <stdlib.h>
int main() {
char buffer[20]; // Character array to store the formatted output
int number = 42;
// Format and write the output to the buffer
int chars_written = snprintf(buffer, sizeof(buffer), "The answer is: %d", number);
if (chars_written < 0) {
// Handle error
perror("snprintf");
exit(EXIT_FAILURE);
}
// Check if the output fit within the buffer
if (chars_written >= sizeof(buffer)) {
printf("Output was truncated.\n");
}
// Print the formatted output
printf("Formatted string: %s\n", buffer);
return 0;
}
In this example:
- We use
snprintf()
to format the string “The answer is: 42” and store it in thebuffer
. - The
sizeof(buffer)
parameter ensures that we limit the output to fit within the size of the buffer. - We check if the output was truncated by comparing
chars_written
to the size of the buffer. - Finally, we print the formatted output stored in the
buffer
.
snprintf()
is a safer alternative to sprintf()
because it prevents buffer overflows by specifying the maximum size of the output buffer.