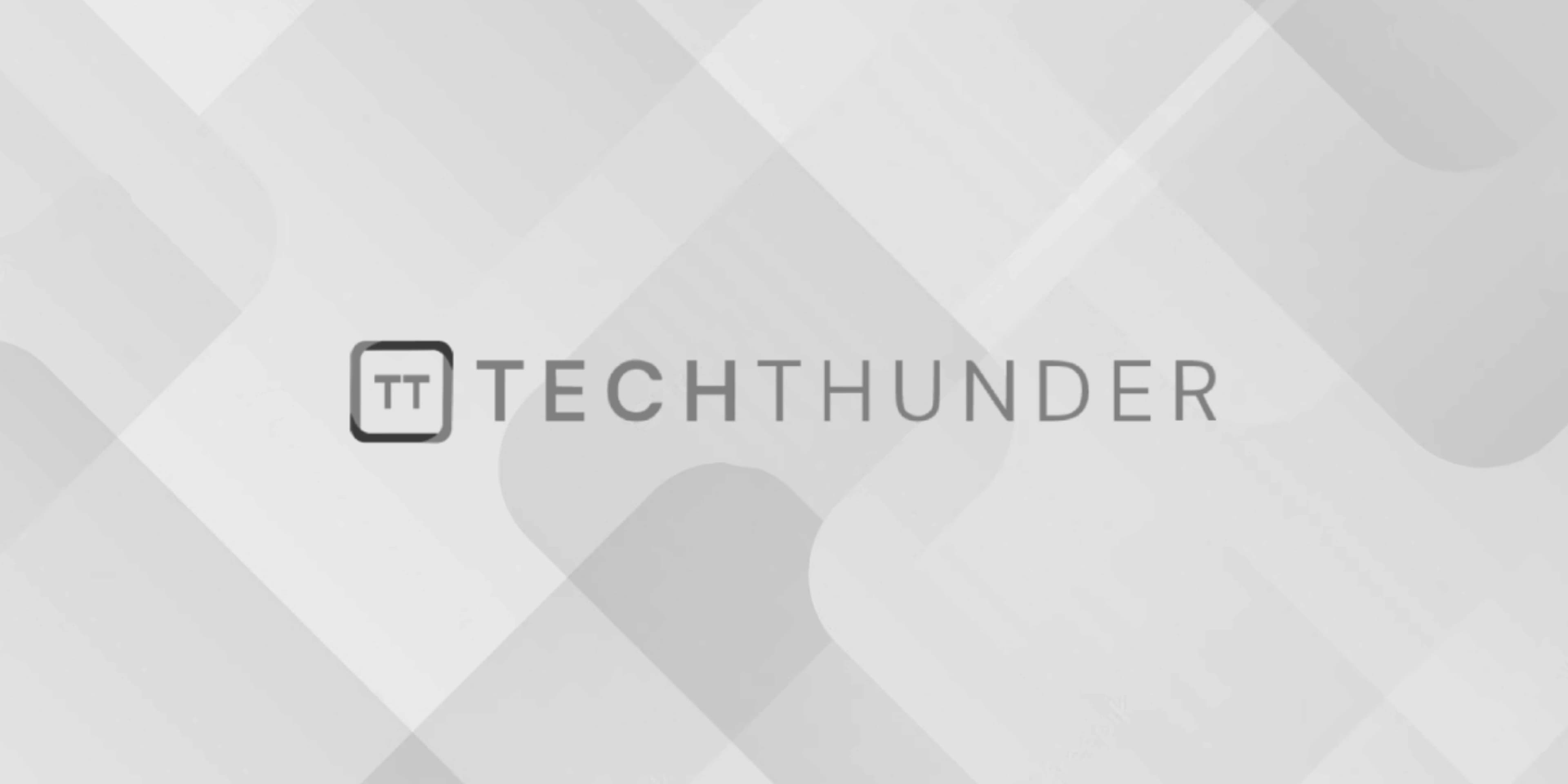
C Pointer to Pointer
The pointer to a pointer, often referred to as a “pointer of pointer” or “double pointer,” is a pointer variable that holds the address of another pointer variable. Pointer to pointer variables are useful in situations where you need to work with pointers indirectly or when you want to modify the value of a pointer itself. To declare and use pointer to pointer variables, you typically use multiple asterisks (**
) to indicate the levels of indirection.
Here’s an example to illustrate pointer to pointer usage:
#include <stdio.h>
int main() {
int value = 42;
int *ptr1 = &value; // Pointer to an integer
int **ptr2 = &ptr1; // Pointer to a pointer to an integer
printf("Value: %d\n", **ptr2); // Access value indirectly through ptr2
// Modifying the value through ptr1
*ptr1 = 100;
printf("Modified Value: %d\n", **ptr2); // Access modified value through ptr2
return 0;
}
In this example:
- We declare an integer variable
value
and a pointerptr1
that points to it. - We declare a pointer to a pointer
ptr2
and initialize it with the address ofptr1
. This meansptr2
holds the address ofptr1
. - We use
**ptr2
to indirectly access and print the value ofvalue
. This demonstrates how you can use a pointer to pointer to access the value of an integer indirectly. - We modify the value of
value
throughptr1
, and the change is reflected when we print the value through**ptr2
.
Pointer to pointer variables are commonly used in scenarios like dynamic memory allocation (e.g., when managing arrays of pointers or creating multi-dimensional arrays), modifying pointer variables within functions, and handling data structures like linked lists, trees, or graphs. They allow for greater flexibility in managing memory and data structures in C programs.