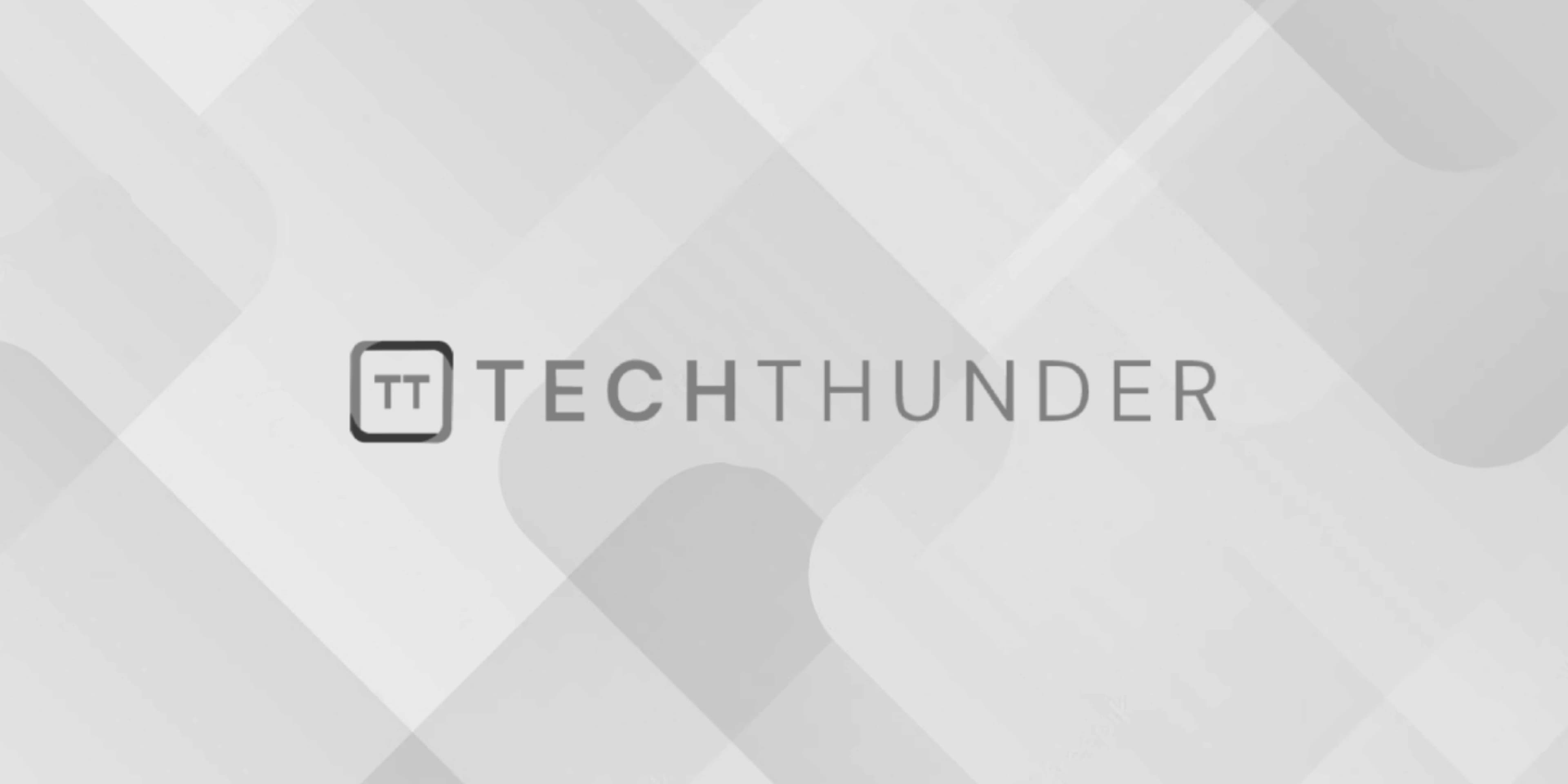
204 views
isdigit() function in C
The isdigit()
function is part of the C Standard Library and is used to determine if a given character is a decimal digit or not. It checks whether a character represents one of the decimal digits from ‘0’ to ‘9’. The function returns a non-zero value (true) if the character is a digit, and it returns 0
(false) otherwise. The isdigit()
function is defined in the ctype.h
header.
Here’s the prototype of the isdigit()
function:
C
#include <ctype.h>
int isdigit(int c);
c
: An integer that represents a character (or the result ofgetc()
orfgetc()
).
The isdigit()
function is useful for checking whether a character is a numeric digit before attempting to perform numeric operations on it. Here’s an example of how to use it:
C
#include <stdio.h>
#include <ctype.h>
int main() {
char ch;
printf("Enter a character: ");
scanf(" %c", &ch); // Note the space before %c to skip leading whitespace
if (isdigit(ch)) {
printf("'%c' is a decimal digit.\n", ch);
} else {
printf("'%c' is not a decimal digit.\n", ch);
}
return 0;
}
In this example:
- We use
scanf
to read a character from the user, and the character is stored in the variablech
. - We then use the
isdigit()
function to check whether the entered character is a decimal digit. - Depending on the result, we print a message indicating whether the character is a decimal digit or not.
This function is particularly helpful when you need to validate or filter input to ensure that it consists of numeric digits.