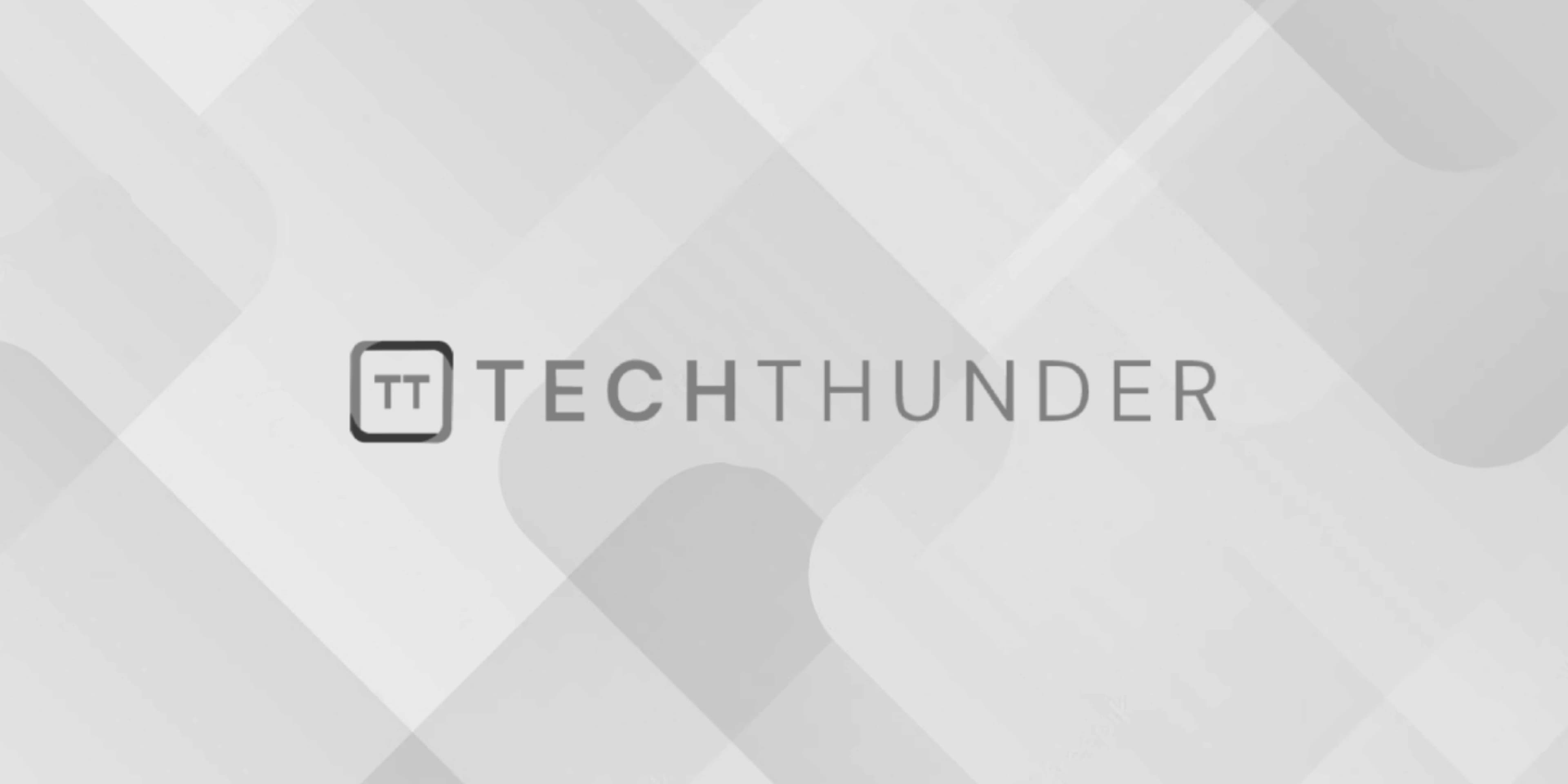
Type Casting vs Type Conversion in C
The type casting and type conversion are related concepts, but they serve different purposes.
Type Conversion:
- Type conversion refers to the automatic or implicit conversion of one data type to another by the C compiler when required.
- It occurs when you perform operations involving different data types, and the compiler automatically converts one or both operands to a common data type.
- Type conversion can occur during arithmetic operations, assignments, and function calls.
- For example, when you add an
int
and afloat
, theint
is automatically converted to afloat
before the addition takes place. - Type conversion can lead to data loss or unexpected results if you’re not careful.
Type Casting:
- Type casting, on the other hand, is an explicit operation that allows you to convert a value from one data type to another manually.
- It’s performed by using casting operators like
(type)
ortype(value)
to specify the desired data type. - Type casting is explicit, meaning that you, as the programmer, explicitly specify the conversion.
- It’s often used when you want to ensure that a specific type of conversion occurs, even if it might result in data loss or a change in representation.
- For example, you can explicitly cast a
float
to anint
using(int)
to truncate the fractional part and obtain an integer result.
Here’s an example illustrating the difference between type conversion and type casting:
#include <stdio.h>
int main() {
int integerResult;
float floatValue = 10.5;
// Type conversion (implicit)
integerResult = floatValue; // Automatic conversion from float to int
printf("Type Conversion: Integer Result = %d\n", integerResult);
// Type casting (explicit)
integerResult = (int)floatValue; // Explicitly cast float to int
printf("Type Casting: Integer Result = %d\n", integerResult);
return 0;
}
In this example, the first assignment involves type conversion, where the float
value is automatically converted to an int
when assigning it to integerResult
. In the second assignment, type casting is explicitly used to convert the float
to an int
. Both methods result in data loss (truncating the fractional part), but the second method makes the conversion explicit.
In summary, type conversion happens automatically by the C compiler when it deems necessary for operations involving different data types, while type casting is an explicit operation where you manually specify the desired conversion. Type casting gives you more control over how data is converted and can be used when you want to ensure a specific type of conversion occurs.