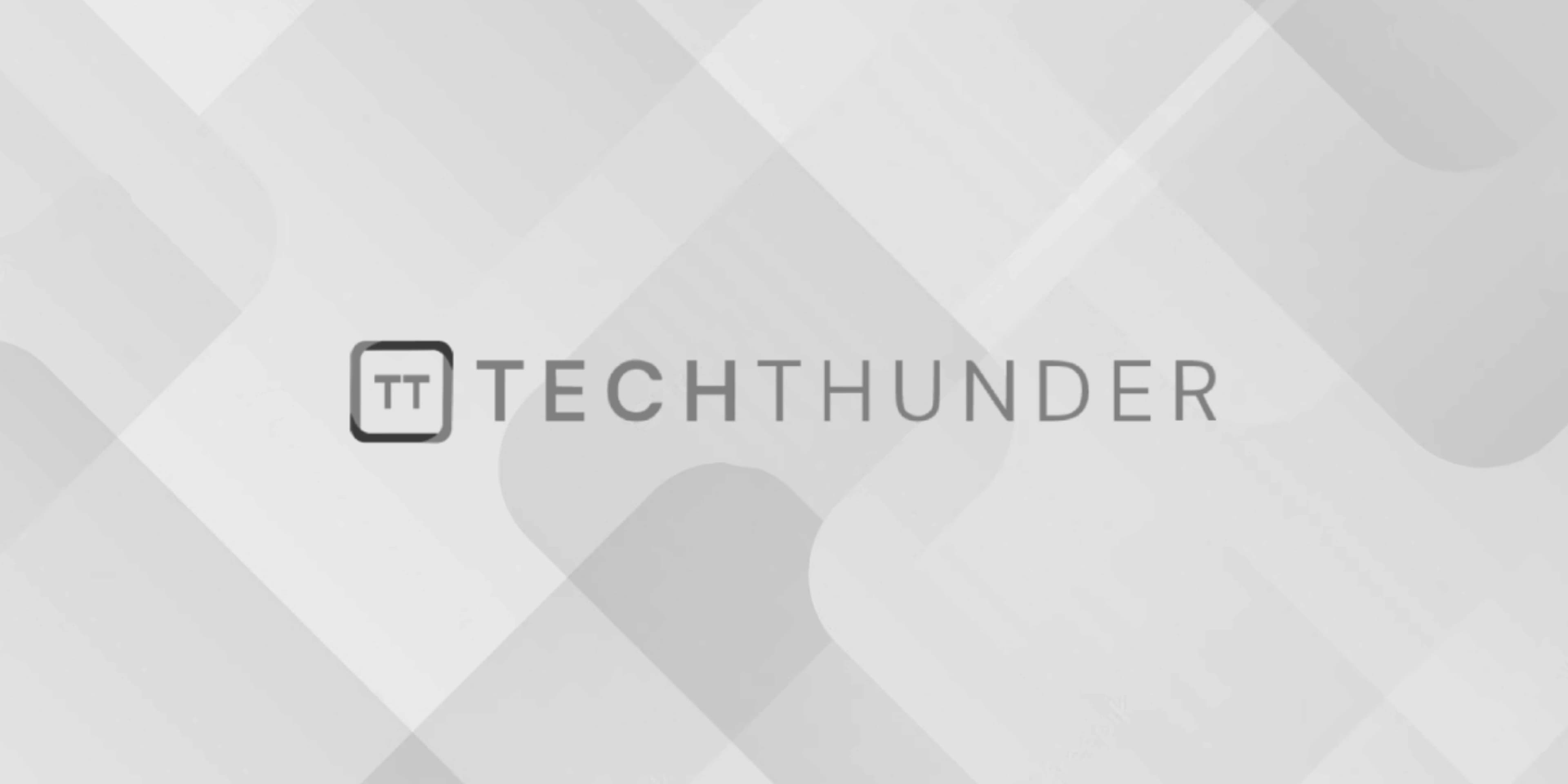
Binary Numbers List in C
Generating a list of binary numbers is a straightforward task in C. However, you need to define the range of decimal numbers for which you want to generate their binary representations. Here’s an example program that generates the binary representation of decimal numbers within a specified range:
#include <stdio.h>
// Function to convert decimal to binary
void decimalToBinary(int decimal) {
if (decimal > 0) {
decimalToBinary(decimal / 2);
printf("%d", decimal % 2);
}
}
int main() {
int lower, upper;
printf("Enter the lower and upper bounds: ");
scanf("%d %d", &lower, &upper);
printf("Binary representations of numbers between %d and %d:\n", lower, upper);
for (int num = lower; num <= upper; ++num) {
printf("%d: ", num);
decimalToBinary(num);
printf("\n");
}
return 0;
}
In this program, the decimalToBinary
function recursively converts a decimal number to its binary representation. It does so by dividing the decimal number by 2 and printing the remainders in reverse order.
Please keep in mind that this example generates the binary representation for each decimal number individually. If you’re looking to generate a list of binary numbers within a range, you would need to specify whether you want the binary numbers to be represented as strings or as actual binary values. The above program generates binary representations as strings.
Also, make sure to validate user input to ensure it’s within an appropriate range.