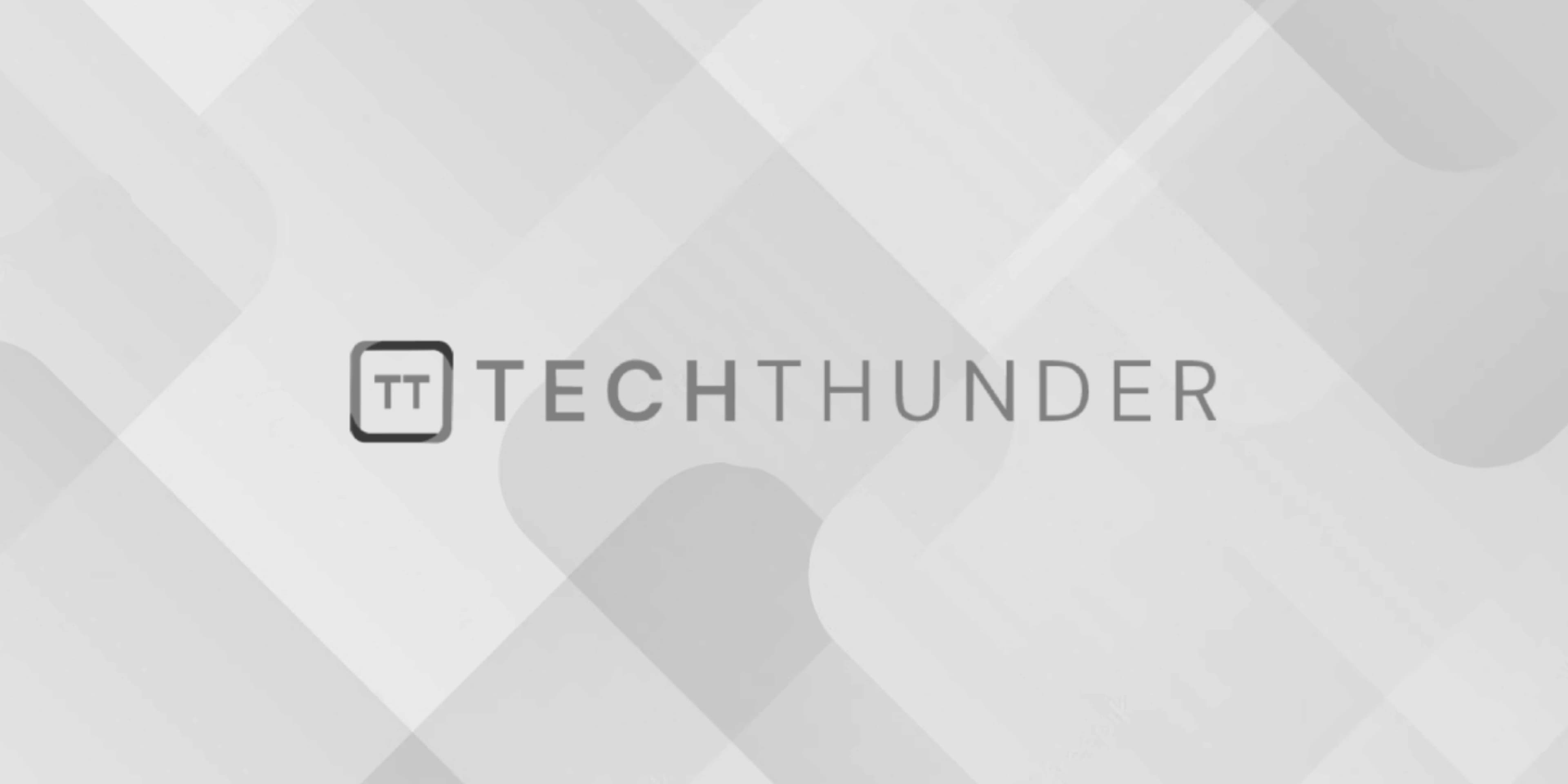
283 views
sizeof() operator in C
The sizeof
operator is used to determine the size in bytes of a data type or a variable. It is a unary operator that returns an integer value representing the size of the specified object or data type in memory. The result of the sizeof
operator is of type size_t
, which is an unsigned integer type.
Here are some common uses of the sizeof
operator in C:
- Size of Data Types:
You can usesizeof
to determine the size of fundamental data types such asint
,char
,float
,double
, and user-defined data types, including structures and unions. For example:
C
printf("Size of int: %zu bytes\n", sizeof(int));
printf("Size of char: %zu bytes\n", sizeof(char));
- Size of Variables:
You can usesizeof
to find the size of variables. For example:
C
int x = 10;
printf("Size of x: %zu bytes\n", sizeof(x));
- Size of Arrays:
sizeof
can also be used to find the size of arrays. The size of an array is the product of the size of its elements and the number of elements. For example:
C
int arr[] = {1, 2, 3, 4, 5};
size_t arrSize = sizeof(arr) / sizeof(arr[0]);
printf("Size of arr: %zu bytes\n", sizeof(arr));
printf("Number of elements in arr: %zu\n", arrSize);
- Size of Structures and Unions:
sizeof
can help you determine the memory footprint of structures and unions. For example:
C
struct Point {
int x;
int y;
};
printf("Size of Point struct: %zu bytes\n", sizeof(struct Point));
- Dynamic Memory Allocation:
sizeof
is commonly used when dynamically allocating memory using functions likemalloc
,calloc
, orrealloc
. It ensures that you allocate the correct amount of memory:
C
int *arr = (int *)malloc(sizeof(int) * 10);
- Checking Sizes at Compile Time:
sizeof
can be used in preprocessor directives to conditionally include code based on the size of data types. For example:
C
#if sizeof(int) == 4
// Code for 32-bit systems
#elif sizeof(int) == 8
// Code for 64-bit systems
#endif
The sizeof
operator is a valuable tool for writing portable and efficient C code, as it allows you to work with data types and memory sizes in a platform-independent manner. Keep in mind that the size of a data type may vary depending on the compiler and the target architecture, so it’s essential to use sizeof
to ensure correctness and portability in your programs.