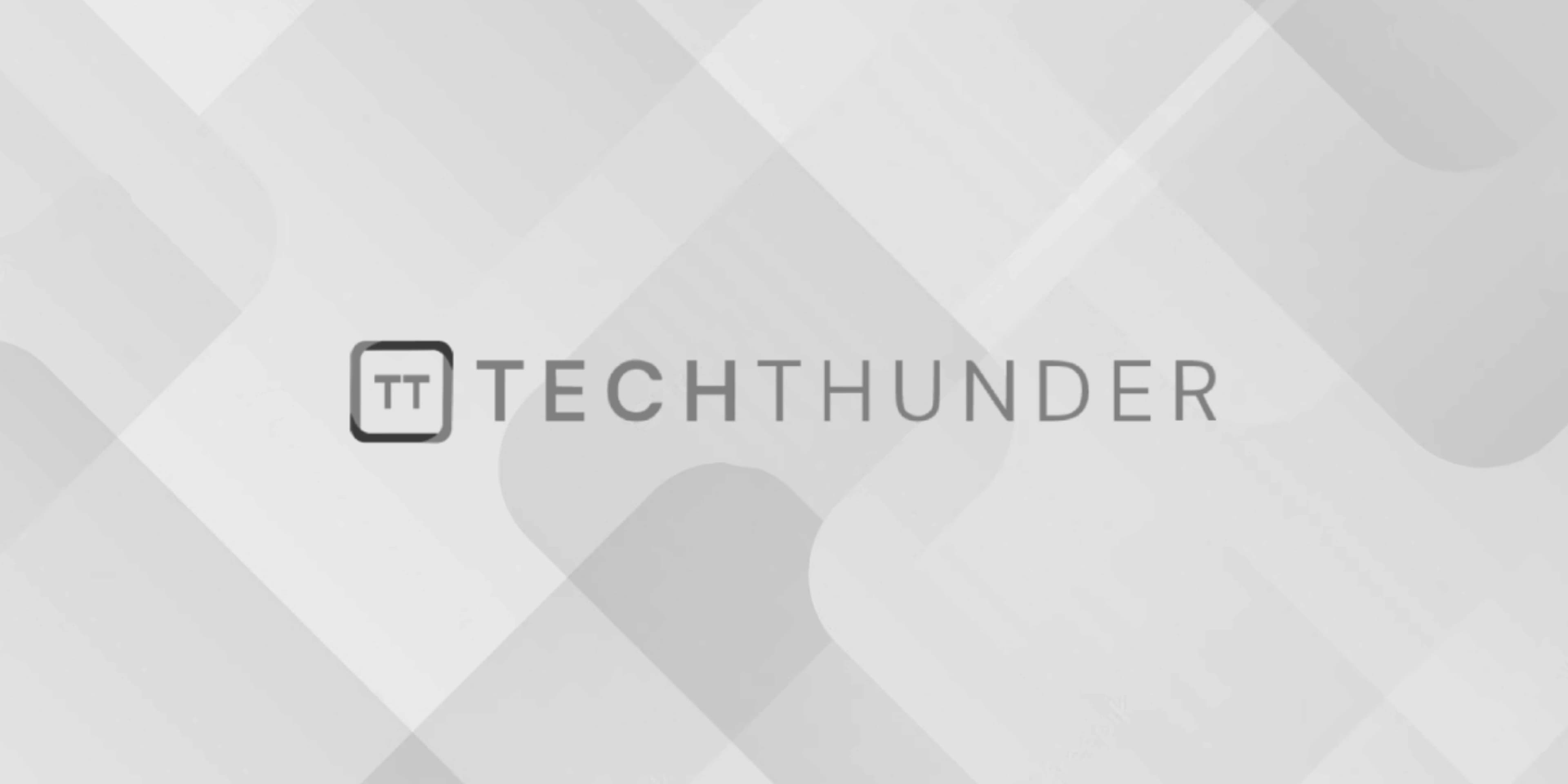
91 views
C Expressions
The expression is a combination of values, variables, operators, and function calls that can be evaluated to produce a result. Expressions can be simple, consisting of a single value or variable, or complex, involving multiple components and operations.
Here are some common elements of C expressions:
- Constants and Variables: Expressions can contain constants (literal values) and variables. For example:
C
int x = 5; // Constant and variable
float pi = 3.1415; // Constant
- Operators: Operators are used to perform operations on values and variables within an expression. C provides various types of operators, including arithmetic operators (+, -, *, /), relational operators (==, !=, <, >), logical operators (&&, ||, !), and assignment operators (=, +=, -=, *=, /=), among others.
- Function Calls: You can include function calls within expressions to obtain their return values. For example:
C
int result = add(2, 3); // Function call within an expression
- Parentheses: Parentheses are used to group expressions and control the order of evaluation. For example:
C
int result = (2 + 3) * 4; // Using parentheses to control order
- Conditional Expressions (Ternary Operator): The ternary operator (
? :
) allows you to create conditional expressions. It has the formcondition ? true_expression : false_expression
. For example:
C
int x = 5;
int y = (x > 0) ? 10 : -10; // Ternary conditional expression
- Bitwise Operators: C provides bitwise operators for performing operations at the bit level. These operators include
&
(bitwise AND),|
(bitwise OR),^
(bitwise XOR), and<<
(left shift), among others. - Compound Expressions: Expressions can be composed of multiple operators and operands. The order of evaluation follows operator precedence and associativity rules.
- Function Pointers: C allows you to create expressions involving function pointers, which can be used to call different functions dynamically based on conditions.
Examples of C expressions:
C
int sum = 5 + 3; // Simple arithmetic expression
int isGreater = (x > y); // Relational expression
int isTrue = (a && b); // Logical expression
int result = factorial(5); // Function call within an expression
int max = (x > y) ? x : y; // Ternary conditional expression
int shiftedValue = value << 2; // Bitwise left shift expression
C expressions are the building blocks of C programs and are used extensively for calculations, decision-making, and data manipulation. Understanding how expressions work and how they are evaluated is fundamental to programming in C.