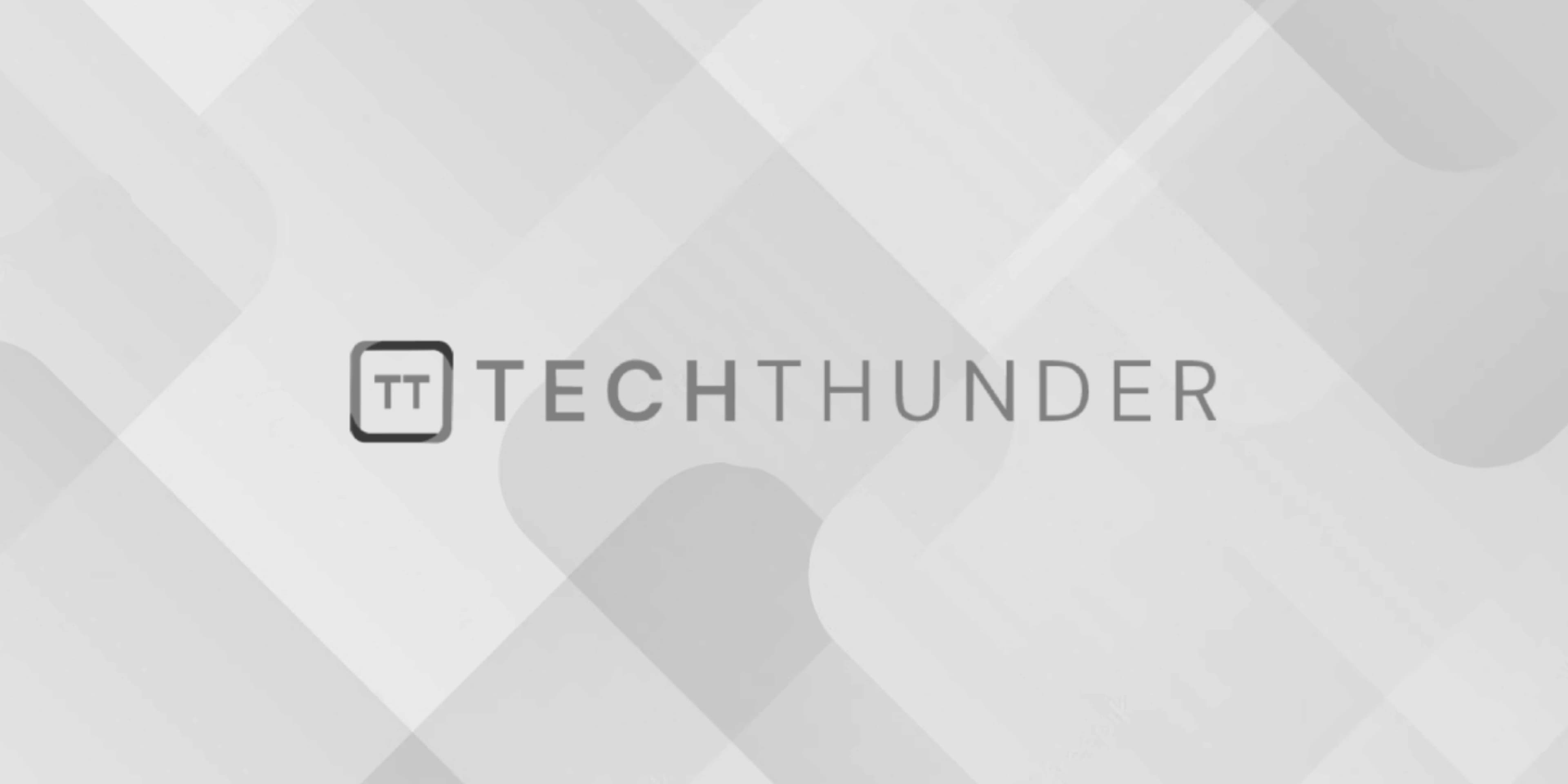
237 views
Reverse Number in C
To reverse a number in C, you can repeatedly extract and print the last digit of the number by taking its remainder when divided by 10. After printing the last digit, you can remove it from the number by dividing it by 10. Repeat this process until the number becomes zero. Here’s a C program to reverse a number:
C
#include <stdio.h>
int main() {
int num, reversedNum = 0;
printf("Enter an integer: ");
scanf("%d", &num);
// Reverse the number
while (num != 0) {
int digit = num % 10; // Extract the last digit
reversedNum = reversedNum * 10 + digit; // Append the digit to the reversed number
num /= 10; // Remove the last digit
}
printf("Reversed number: %d\n", reversedNum);
return 0;
}
In this program:
- We input an integer from the user.
- We initialize
reversedNum
to 0, which will store the reversed number. - Inside a
while
loop, we repeatedly extract the last digit ofnum
using the modulo operator (%
). This digit is stored in thedigit
variable. - We append the
digit
toreversedNum
by multiplyingreversedNum
by 10 and adding thedigit
to it. This effectively adds the digit to the reversed number. - We remove the last digit from
num
by dividing it by 10. - We repeat this process until
num
becomes zero. - Finally, we print the
reversedNum
, which contains the reversed digits of the input number.
Compile and run the program, and it will reverse the entered integer and display the reversed number.