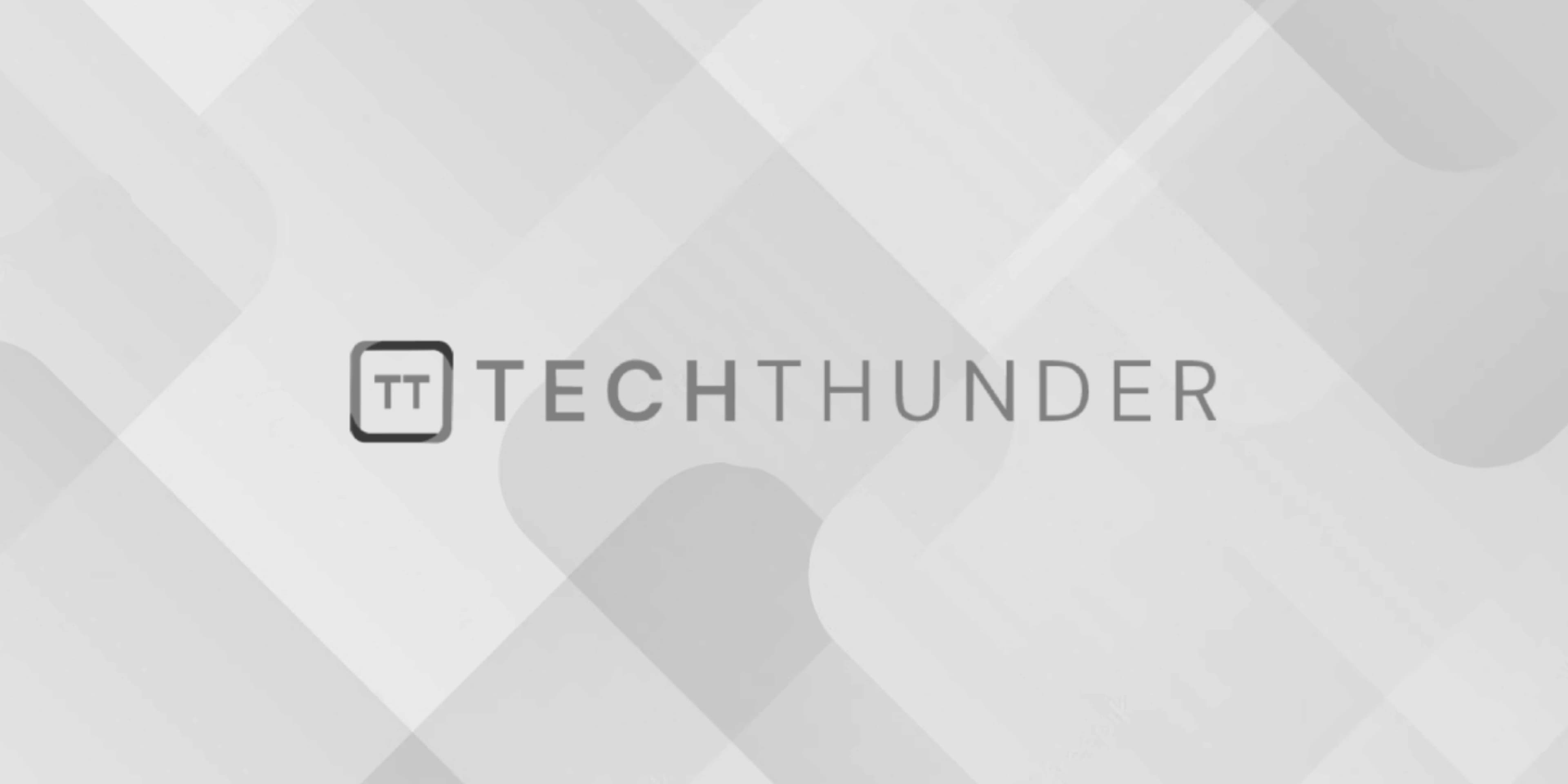
Float in C
The float
data type is used to represent single-precision floating-point numbers. It is one of the basic data types in C for representing real numbers with decimal points. The float
data type typically occupies 4 bytes (32 bits) in memory, and it provides a reasonable range of values with decimal precision.
Here’s the basic syntax for declaring a float
variable:
float variable_name;
Here’s an example of declaring and using a float
variable:
#include <stdio.h>
int main() {
float price = 12.99; // Declare and initialize a float variable
float discount = 0.2;
float discounted_price = price * (1 - discount); // Perform arithmetic operations
printf("Original Price: $%.2f\n", price); // Print the original price with 2 decimal places
printf("Discounted Price: $%.2f\n", discounted_price);
return 0;
}
In this example:
- We declare a
float
variableprice
and initialize it with a value of12.99
. - We also declare a
float
variablediscount
and set it to0.2
. - We perform arithmetic operations using
float
variables to calculate the discounted price. - We use the
%f
format specifier in theprintf
function to displayfloat
values. The.2
in%.2f
specifies that we want to display two decimal places.
It’s important to note that float
variables have limitations in terms of precision and range. If you need higher precision, you can use the double
data type, which provides double-precision floating-point numbers and occupies 8 bytes (64 bits) in memory. Additionally, for even higher precision, you can use the long double
data type, which offers extended precision, although its size and behavior may vary across different systems.