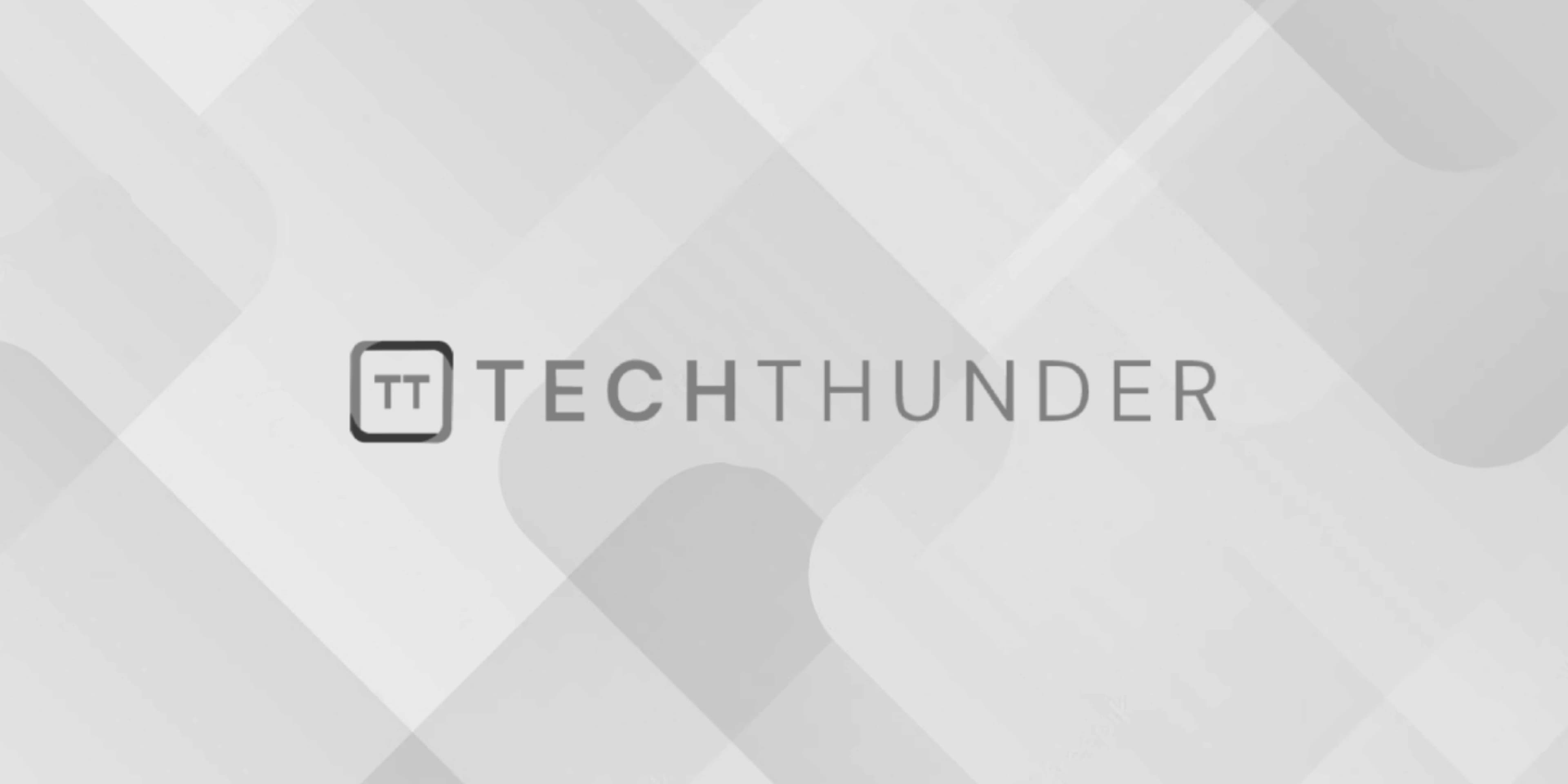
59 views
C fprintf() fscanf()
The fprintf()
and fscanf()
are functions used for formatted input and output to and from files, respectively. These functions are similar to printf()
and scanf()
, which are used for formatted input and output to and from the standard input and output (usually the console).
Here’s a brief overview of fprintf()
and fscanf()
:
fprintf()
Function:
- The
fprintf()
function is used to write formatted data to a file. - It takes three main arguments:
- A file pointer (
FILE*
) to the file where you want to write the data. - A format string, similar to the format string used in
printf()
, that specifies the format of the data to be written. - Additional arguments corresponding to the format specifiers in the format string.
- A file pointer (
- Example of
fprintf()
:#include <stdio.h> int main() { FILE *file = fopen("output.txt", "w"); // Open a file for writing if (file != NULL) { int x = 42; fprintf(file, "The answer is: %d", x); fclose(file); // Close the file } return 0; }
fscanf()
Function:
- The
fscanf()
function is used to read formatted data from a file. - It takes three main arguments:
- A file pointer (
FILE*
) to the file from which you want to read the data. - A format string, similar to the format string used in
scanf()
, that specifies the format of the data to be read. - Additional arguments (pointers) where the data will be stored based on the format specifiers in the format string.
- A file pointer (
- Example of
fscanf()
:#include <stdio.h> int main() { FILE *file = fopen("input.txt", "r"); // Open a file for reading if (file != NULL) { int x; fscanf(file, "The answer is: %d", &x); printf("Read from file: %d\n", x); fclose(file); // Close the file } return 0; }
In both examples, we open a file using fopen()
, perform either writing or reading operations using fprintf()
or fscanf()
, and close the file using fclose()
when we’re done. These functions allow you to work with files in a formatted manner, making it easier to read and write structured data.