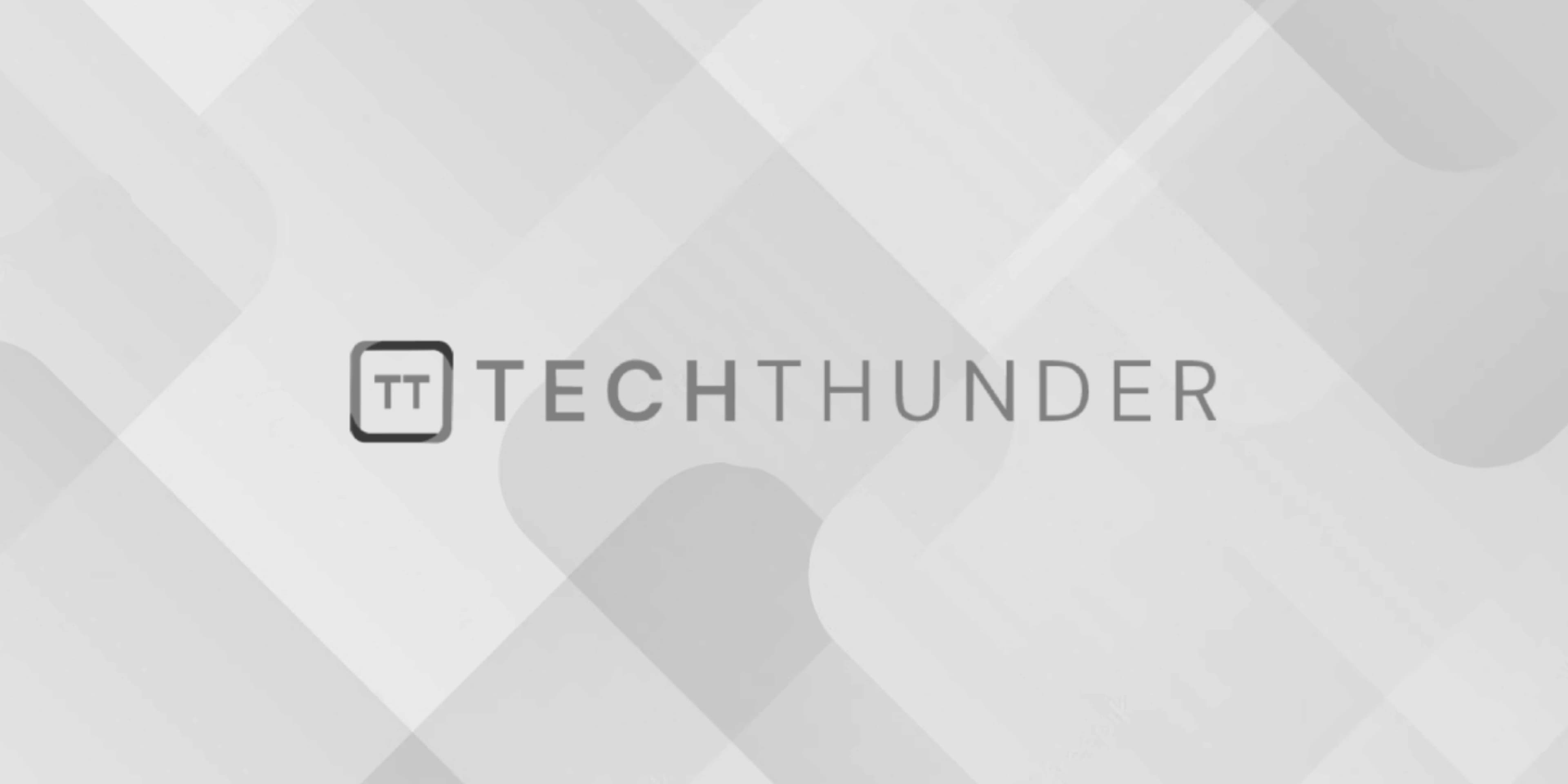
isalnum() function in C
The isalnum()
function is a library function declared in the <ctype.h>
header. It is used to determine whether a given character is alphanumeric, which means it is either a letter (a-z or A-Z) or a digit (0-9). Here’s the function signature:
int isalnum(int c);
c
: The integer representation of the character to be tested.
The function returns a non-zero (true) value if the character is alphanumeric and 0 (false) if it’s not.
Here’s an example of how to use isalnum()
:
#include <stdio.h>
#include <ctype.h>
int main() {
char ch1 = 'A';
char ch2 = '7';
char ch3 = '$';
if (isalnum(ch1)) {
printf("%c is alphanumeric.\n", ch1);
} else {
printf("%c is not alphanumeric.\n", ch1);
}
if (isalnum(ch2)) {
printf("%c is alphanumeric.\n", ch2);
} else {
printf("%c is not alphanumeric.\n", ch2);
}
if (isalnum(ch3)) {
printf("%c is alphanumeric.\n", ch3);
} else {
printf("%c is not alphanumeric.\n", ch3);
}
return 0;
}
In this example, isalnum()
is used to check whether three characters (ch1
, ch2
, and ch3
) are alphanumeric. The function returns true for 'A'
and '7'
since they are alphanumeric characters, and it returns false for '$'
since it’s not alphanumeric.
Output:
A is alphanumeric.
7 is alphanumeric.
$ is not alphanumeric.
You can use isalnum()
to validate and process input where you expect alphanumeric characters or to filter out characters that don’t meet this criterion.