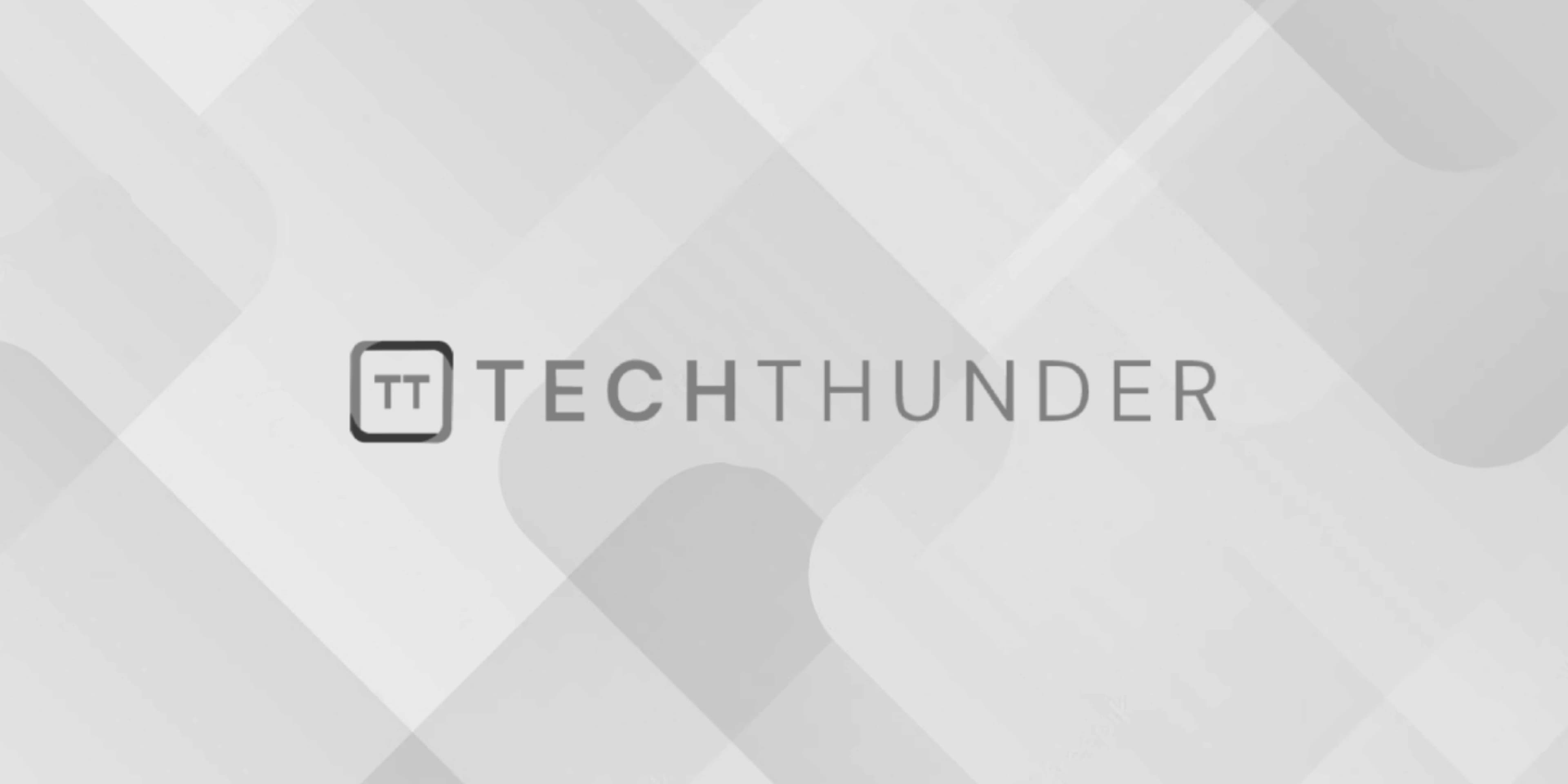
ASCII value in C
You can obtain the ASCII (American Standard Code for Information Interchange) value of a character using the int
data type. The ASCII value represents the integer value that corresponds to a specific character in the ASCII character set. Here’s how you can obtain the ASCII value of a character in C:
#include <stdio.h>
int main() {
char character = 'A'; // Define a character
int asciiValue = (int)character; // Cast the character to an integer
printf("The ASCII value of '%c' is %d\n", character, asciiValue);
return 0;
}
In this example, the character 'A'
is assigned to the variable character
. By casting it to an int
using (int)
, you obtain the ASCII value of that character. The printf
statement then prints both the character and its ASCII value.
When you run this program, it will output:
The ASCII value of 'A' is 65
Each character in the ASCII character set is associated with a unique integer value, allowing you to convert between characters and their corresponding ASCII values using this method.