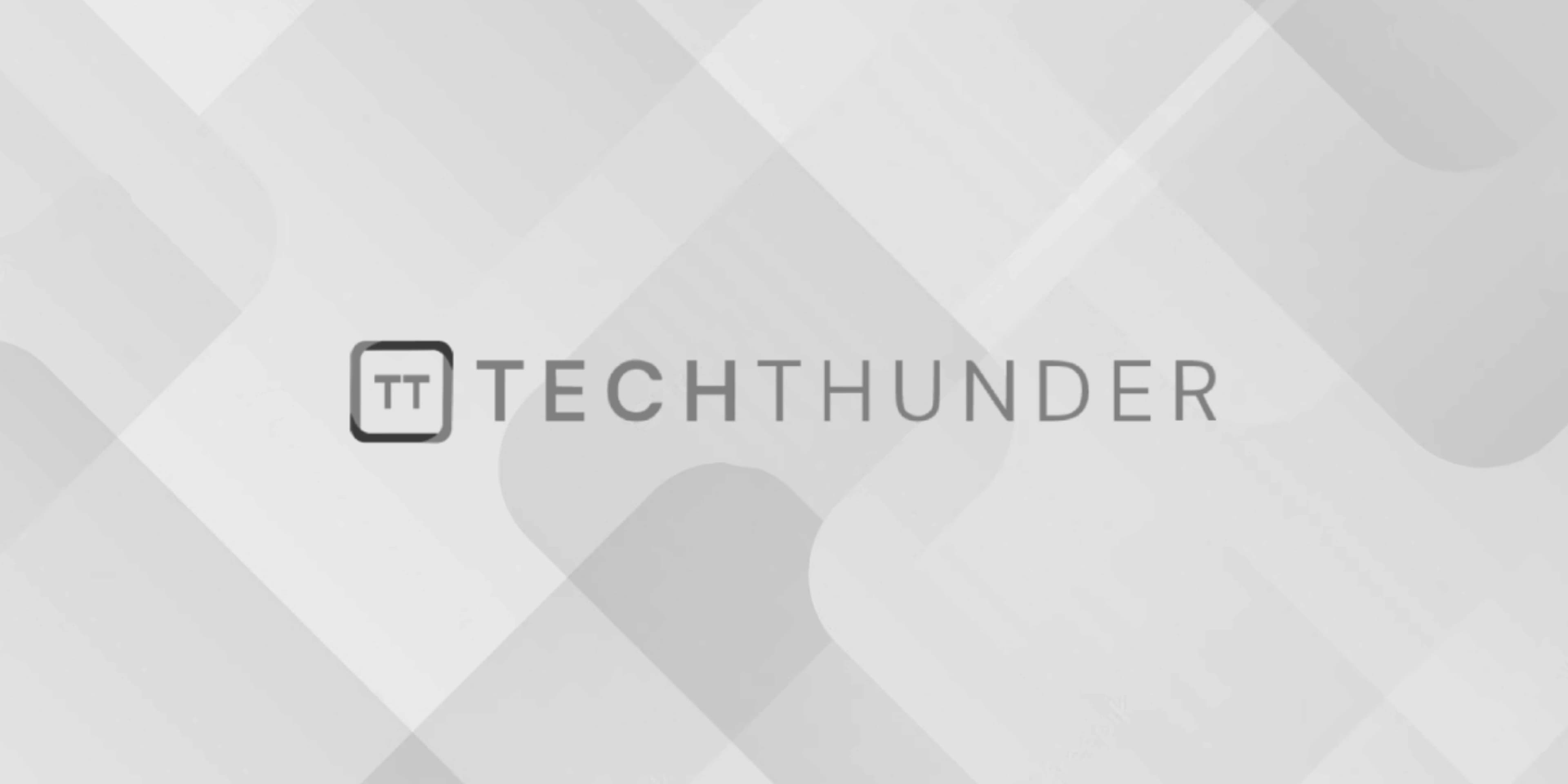
405 views
memmove() in C
The memmove()
function in C is used to copy a block of memory from a source location to a destination location. It is similar to memcpy()
, but it can handle overlapping source and destination regions of memory. The memmove()
function is part of the C Standard Library, and its prototype is as follows:
C
void *memmove(void *dest, const void *src, size_t n);
dest
: A pointer to the destination memory location where the data will be copied.src
: A pointer to the source memory location from which the data will be copied.n
: The number of bytes to copy.
Here’s an example of how to use memmove()
:
C
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char buffer[20];
// Copy the first 5 characters from 'str' to 'buffer'
memmove(buffer, str, 5);
buffer[5] = '\0'; // Null-terminate 'buffer'
printf("Original string: %s\n", str);
printf("Copied string: %s\n", buffer);
return 0;
}
In this example:
- We have a source string
str
containing the text “Hello, World!” and a destination character arraybuffer
. - We use
memmove()
to copy the first 5 characters fromstr
tobuffer
. - We manually null-terminate
buffer
to ensure it’s a valid C string. - After copying,
buffer
contains the copied substring “Hello.”
Important points to note about memmove()
:
- It is safer to use
memmove()
when dealing with overlapping memory regions because it handles the copying correctly, ensuring that data is not corrupted. memmove()
performs byte-by-byte copying, making it suitable for both text and binary data.- Like
memcpy()
,memmove()
returns a pointer to the destination, but this return value is typically not used. - Be cautious when using
memmove()
with overlapping memory regions to avoid unintended consequences. In cases where overlapping occurs,memmove()
is the safer choice, whilememcpy()
may lead to unexpected results. - Just like other memory operations, ensure that the destination buffer has enough space to accommodate the copied data to prevent buffer overflow.