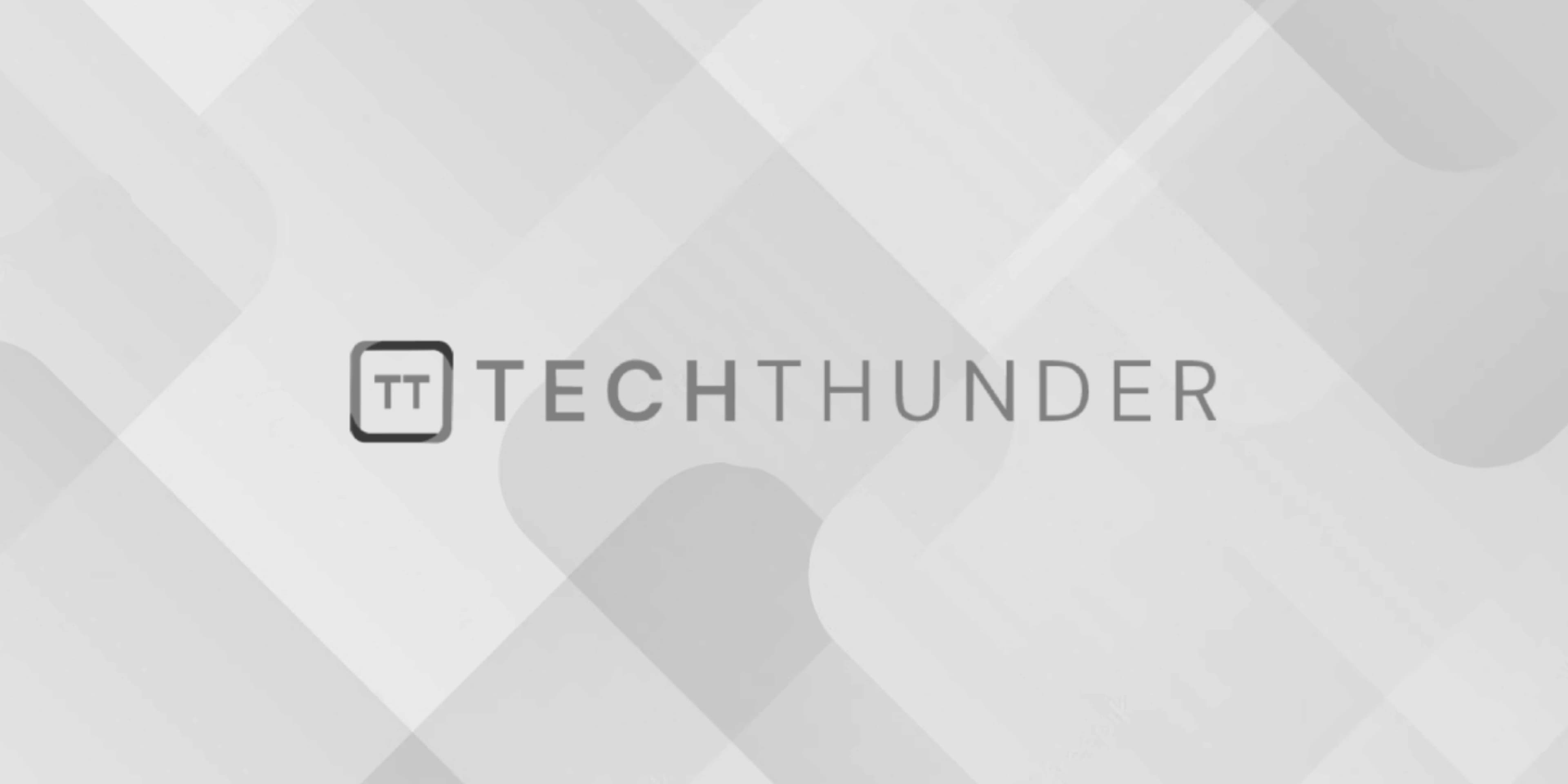
135 views
Prime Numbers List in C
The simple C program that generates a list of prime numbers within a specified range:
C
#include <stdio.h>
// Function to check if a number is prime
int isPrime(int num) {
if (num <= 1) {
return 0; // 0 and 1 are not prime
}
for (int i = 2; i * i <= num; ++i) {
if (num % i == 0) {
return 0; // Found a divisor
}
}
return 1; // No divisors found
}
int main() {
int lower, upper;
printf("Enter the lower and upper bounds: ");
scanf("%d %d", &lower, &upper);
printf("Prime numbers between %d and %d:\n", lower, upper);
for (int num = lower; num <= upper; ++num) {
if (isPrime(num)) {
printf("%d ", num);
}
}
printf("\n");
return 0;
}
In this program, the isPrime
function checks if a given number is prime by iterating from 2 up to the square root of the number. If the number is divisible by any smaller number, it’s not prime. The main program takes the lower and upper bounds from the user and generates a list of prime numbers within that range.
Remember that this is a basic implementation and might not be the most efficient for generating prime numbers for very large ranges. More advanced algorithms like the Sieve of Eratosthenes can be used for better performance. Always validate user input to ensure it’s within an appropriate range.