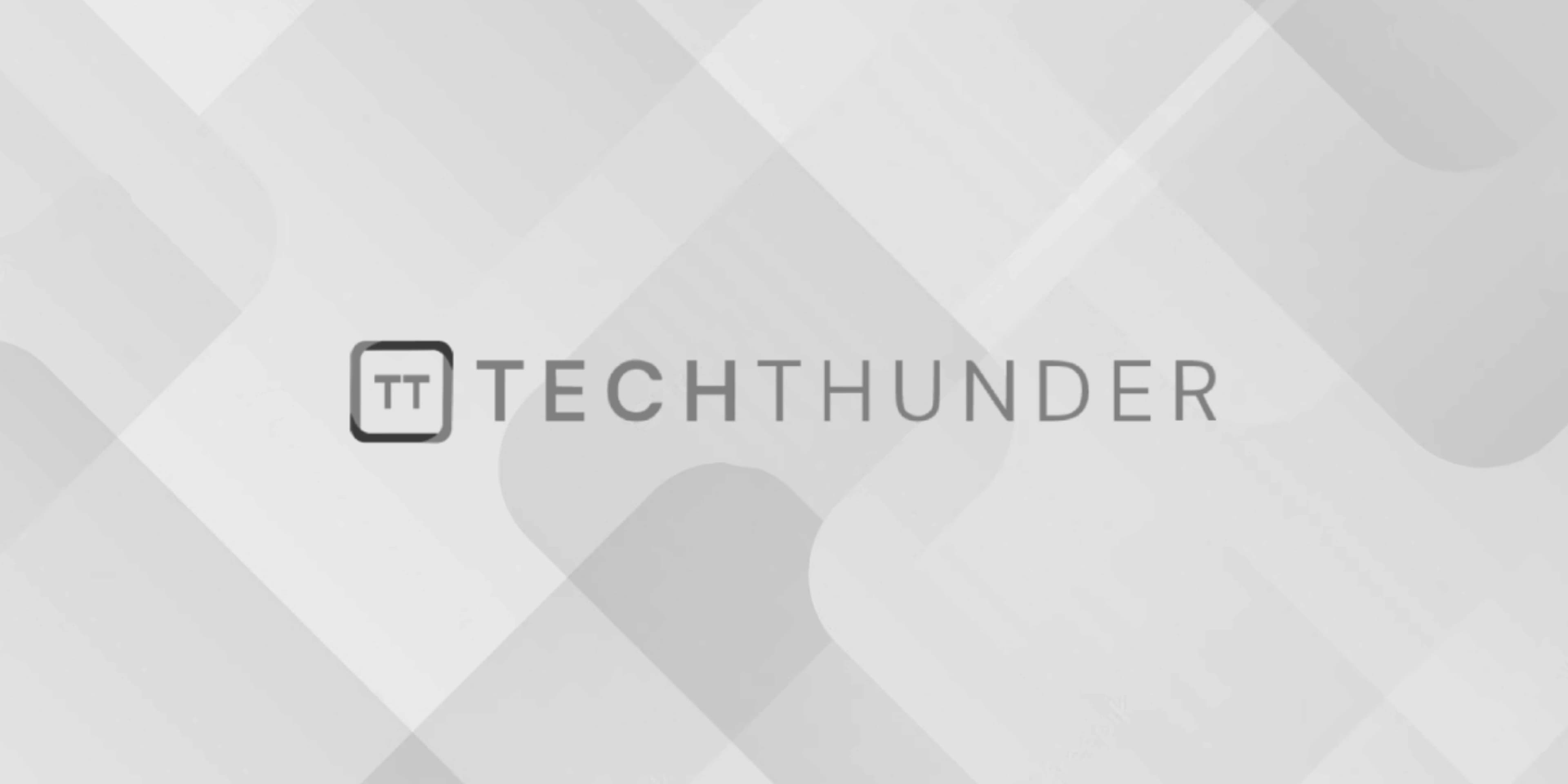
C strstr()
The strstr()
is a standard library function that is used to find the first occurrence of a substring (a sequence of characters) within a given string. It’s part of the <string.h>
header and is commonly used for searching and manipulating strings in C programs.
Here is the syntax of strstr()
:
char *strstr(const char *haystack, const char *needle);
haystack
is the string in which you want to search for the substring.needle
is the substring you want to find within thehaystack
.
The strstr()
function returns a pointer to the first occurrence of the needle
substring within the haystack
string. If the needle
is not found within the haystack
, it returns a null pointer (NULL
).
Here’s an example of using strstr()
:
#include <stdio.h>
#include <string.h>
int main() {
const char *haystack = "The quick brown fox jumps over the lazy dog.";
const char *needle = "fox";
char *result = strstr(haystack, needle);
if (result != NULL) {
printf("Substring found at position: %ld\n", result - haystack);
} else {
printf("Substring not found.\n");
}
return 0;
}
In this example, we have a haystack
string containing a sentence and a needle
string containing “fox.” We use strstr()
to search for the “fox” substring within the haystack
. If the substring is found, strstr()
returns a pointer to the first occurrence, and we calculate its position by subtracting the haystack
pointer from the result pointer.
It’s important to note that strstr()
searches for the first occurrence of the substring and does not find subsequent occurrences. If you need to find all occurrences of a substring, you may need to use a loop and repeatedly call strstr()
.
Here’s an example that finds all occurrences of a substring:
#include <stdio.h>
#include <string.h>
int main() {
const char *haystack = "The quick brown fox jumps over the lazy fox.";
const char *needle = "fox";
char *result = strstr(haystack, needle);
while (result != NULL) {
printf("Substring found at position: %ld\n", result - haystack);
result = strstr(result + 1, needle); // Move to the next character and search again
}
return 0;
}
This modified example uses a loop to find all occurrences of the “fox” substring within the haystack
string. It repeatedly calls strstr()
while incrementing the search position to find subsequent occurrences.