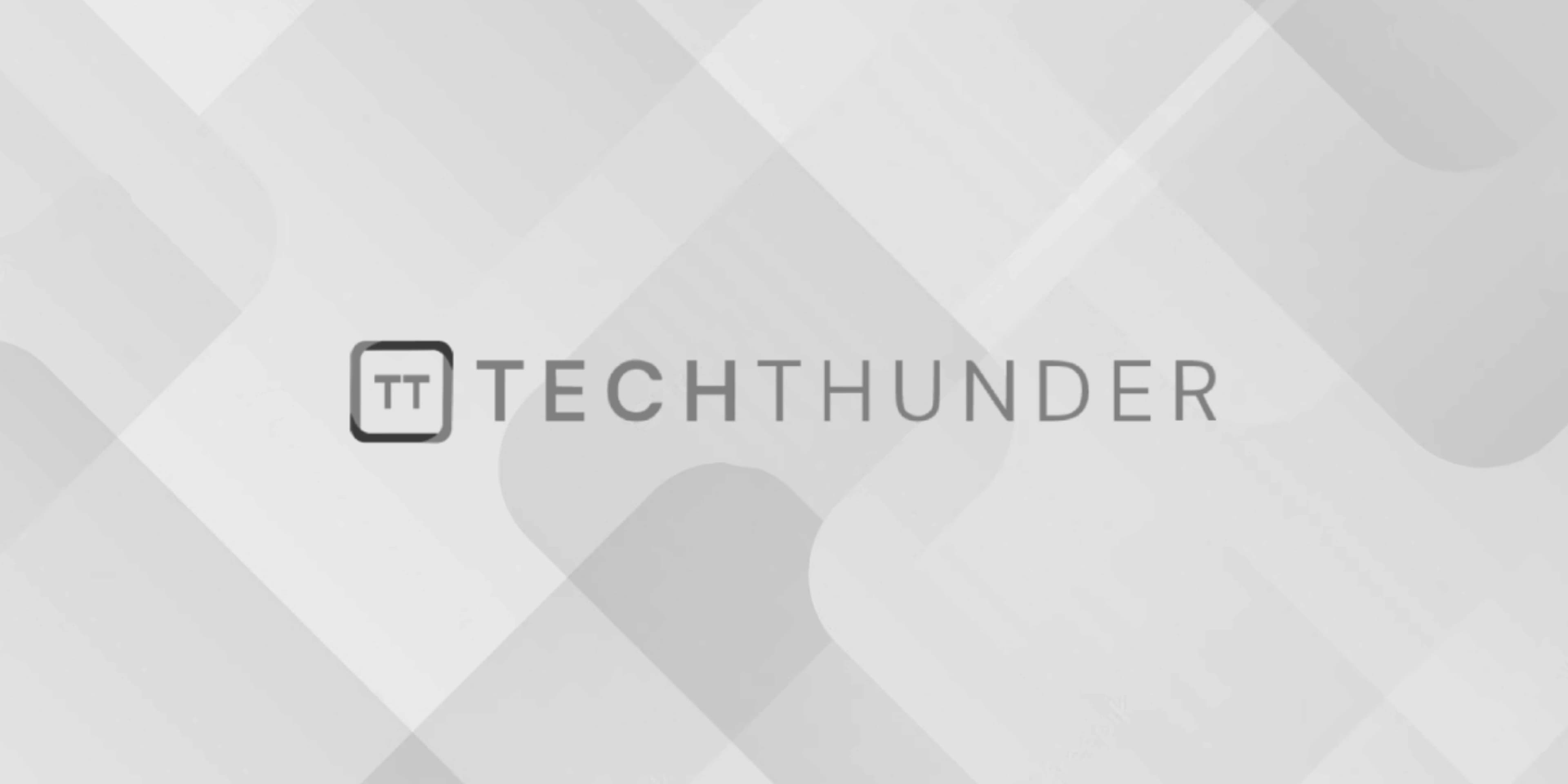
165 views
Decision Making Statements in C
Decision-making statements in C are used to control the flow of a program based on certain conditions. These statements allow you to execute different blocks of code based on whether a particular condition is true or false. The main decision-making statements in C are:
- if Statement:
Theif
statement is used to execute a block of code only if a given condition is true.
C
if (condition) {
// Code to be executed if condition is true
}
- if-else Statement:
Theif-else
statement allows you to execute one block of code if a condition is true and another block of code if the condition is false.
C
if (condition) {
// Code to be executed if condition is true
} else {
// Code to be executed if condition is false
}
- if-else if-else Statement:
Theif-else if-else
statement is used when you have multiple conditions to check and execute different blocks of code based on which condition is true.
C
if (condition1) {
// Code to be executed if condition1 is true
} else if (condition2) {
// Code to be executed if condition2 is true
} else {
// Code to be executed if none of the conditions are true
}
- switch Statement:
Theswitch
statement allows you to evaluate an expression against multiple possible case values. It’s used when you have a limited number of possible cases and want to execute different code for each case.
C
switch (expression) {
case value1:
// Code to be executed if expression == value1
break;
case value2:
// Code to be executed if expression == value2
break;
// ...
default:
// Code to be executed if none of the cases match
}
Keep in mind these important points:
- Each
if
andelse if
condition must evaluate to either true or false (1 or 0). - In the
switch
statement, each case label should be a constant value that matches the type of theexpression
. - The
break
statement is used to exit theswitch
statement after executing the relevant case. Withoutbreak
, execution will continue to the next case even if it doesn’t match.
Decision-making statements help control the program’s behavior based on specific conditions, allowing you to create more flexible and responsive programs.