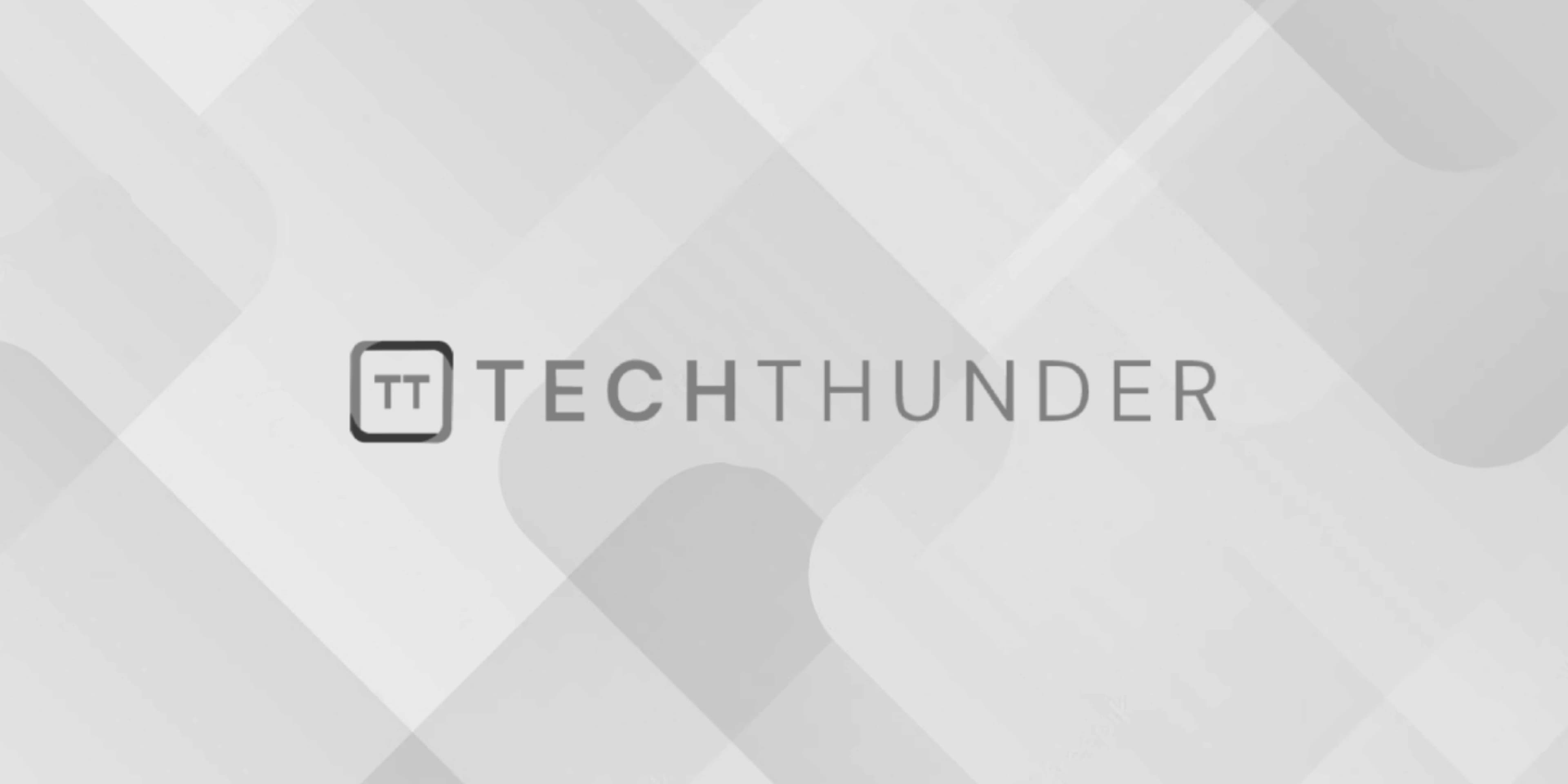
98 views
Palindrome Number in C
A palindrome number is a number that reads the same forwards and backwards. To check if a number is a palindrome in C, you can reverse the number and compare it with the original number. If they are the same, then the number is a palindrome. Here’s a C program to check for palindromic numbers:
C
#include <stdio.h>
int main() {
int num, reversedNum = 0, originalNum, remainder;
printf("Enter an integer: ");
scanf("%d", &num);
originalNum = num; // Store the original number
// Reverse the number
while (num > 0) {
remainder = num % 10; // Extract the last digit
reversedNum = reversedNum * 10 + remainder; // Append the digit to the reversed number
num /= 10; // Remove the last digit
}
// Check if it's a palindrome
if (originalNum == reversedNum) {
printf("%d is a palindrome number.\n", originalNum);
} else {
printf("%d is not a palindrome number.\n", originalNum);
}
return 0;
}
In this program:
- We input an integer from the user.
- We store the original number in the
originalNum
variable for comparison later. - We reverse the number and store it in the
reversedNum
variable using awhile
loop. We extract the last digit of the number, append it toreversedNum
, and remove the last digit from the original number in each iteration. - Finally, we compare the
originalNum
with thereversedNum
to determine whether it’s a palindrome or not and print the result accordingly.
Compile and run the program, and it will check if the entered integer is a palindrome or not.