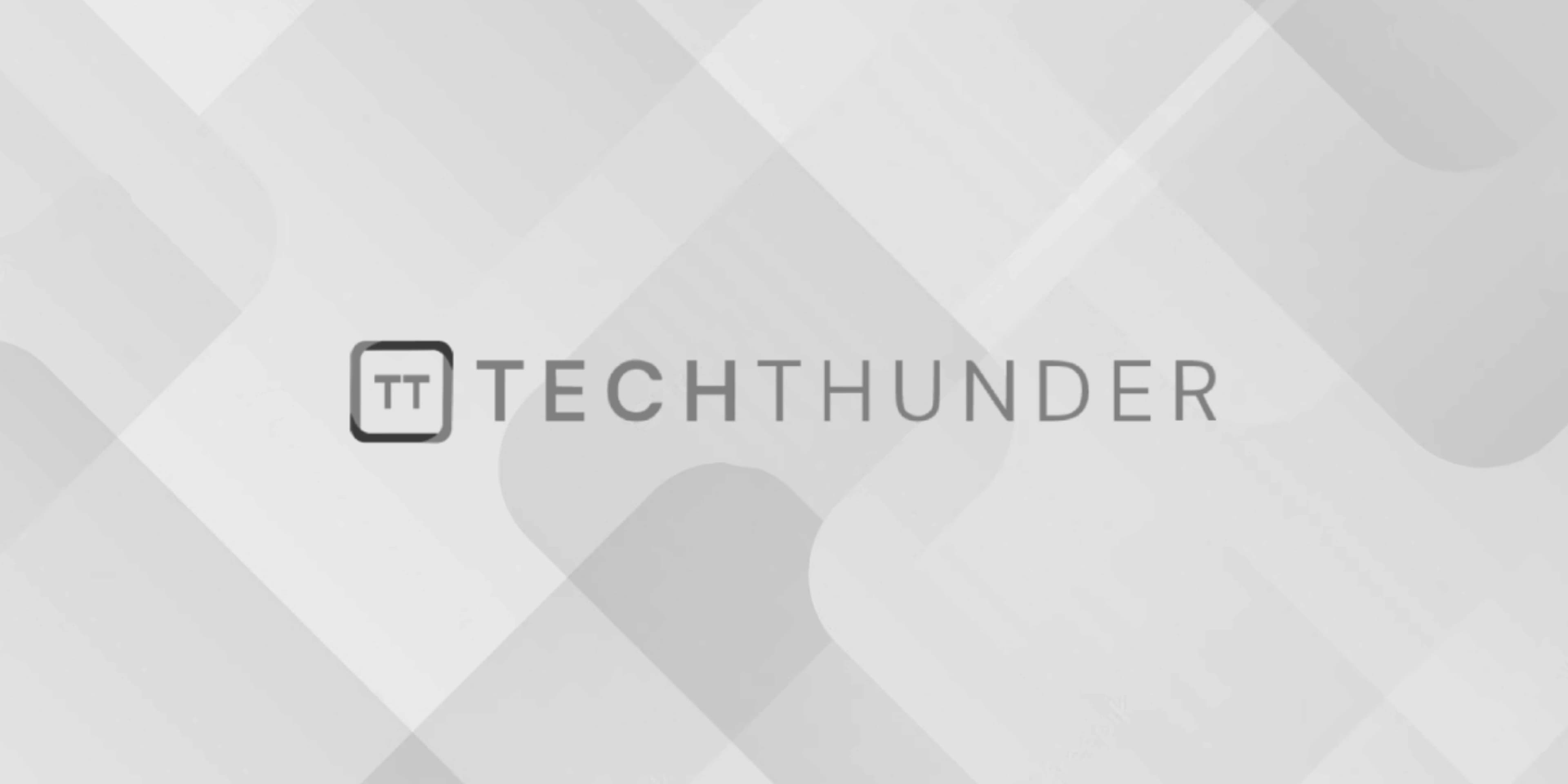
sprintf() in C
The sprintf()
function is used to format and store a series of characters as a string in a character array. It is part of the C Standard Library and is declared in the stdio.h
header file. sprintf()
is similar to printf()
, but instead of printing the formatted string to the standard output (typically the console), it stores the formatted string in a character array.
The syntax of sprintf()
is as follows:
int sprintf(char *str, const char *format, ...);
str
: A pointer to a character array where the formatted string will be stored.format
: A format string that specifies how the data should be formatted....
: Additional arguments that correspond to the values to be formatted and inserted into the string.
Here’s an example of how to use sprintf()
to format and store a string:
#include <stdio.h>
int main() {
char buffer[50]; // Character array to store the formatted string
int num = 42;
double pi = 3.14159;
char name[] = "John";
// Using sprintf to format and store a string in the buffer
sprintf(buffer, "Integer: %d, Double: %.2lf, String: %s", num, pi, name);
// Printing the formatted string stored in the buffer
printf("Formatted String: %s\n", buffer);
return 0;
}
In this example:
- We declare a character array
buffer
to store the formatted string. - We have three variables: an integer
num
, a doublepi
, and a stringname
. - We use
sprintf()
to format these variables into a string and store it in thebuffer
using the specified format. - Finally, we print the formatted string stored in the
buffer
usingprintf()
.
The output of this program will be:
Formatted String: Integer: 42, Double: 3.14, String: John
It’s important to ensure that the character array (str
in sprintf()
) is large enough to accommodate the formatted string, including the null-terminator ('\0'
). Failure to do so can result in buffer overflow, which is a security vulnerability. Always make sure that the destination buffer is large enough to hold the formatted string, or consider using safer alternatives like snprintf()
that allow you to specify the maximum buffer size.