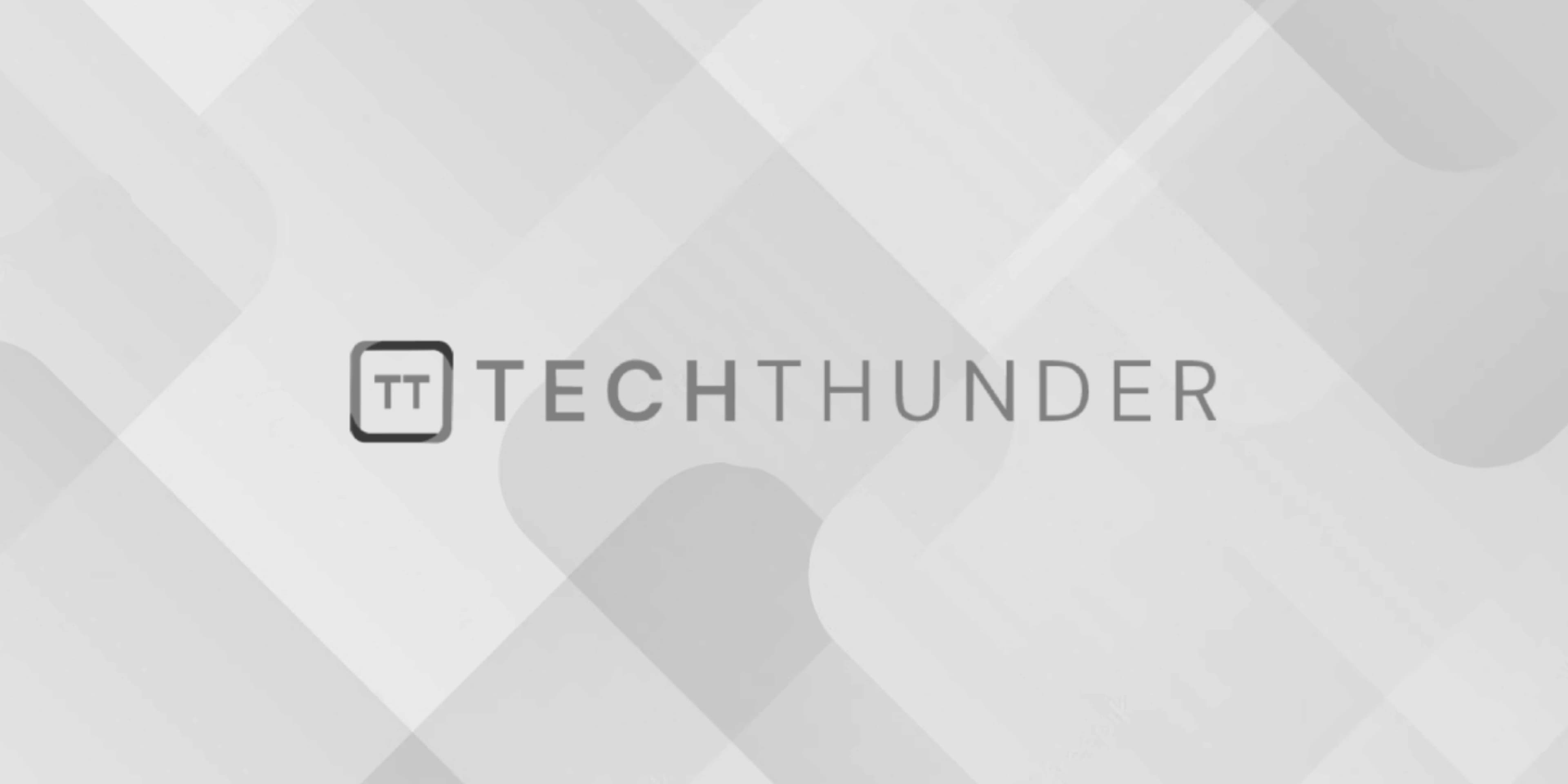
120 views
Assert in C
The assert()
macro is a debugging aid used for ensuring that certain conditions are met during program execution. It’s part of the assert.h
header and is typically used as a debugging and error-checking tool during development.
The assert()
macro has the following syntax:
C
#include <assert.h>
void assert(int expression);
expression
: An expression that represents a condition to be checked. If the expression evaluates to false (i.e., zero), theassert()
macro triggers an assertion failure, which typically leads to the program terminating.
Here’s how assert()
works:
- When the
assert()
macro is encountered in the code, it evaluates the given expression. - If the expression is true (non-zero), the program continues executing as usual, and there is no effect.
- If the expression is false (zero), an assertion failure occurs. This typically triggers a message that includes the file name, line number, and the failed expression. The program then terminates or can be configured to abort with a specific error code.
Here’s an example of how to use assert()
:
C
#include <stdio.h>
#include <assert.h>
int main() {
int x = 10;
// Check if x is greater than 20
assert(x > 20);
printf("x is greater than 20.\n");
return 0;
}
In this example, the assertion x > 20
is false since x
is equal to 10. When you run this program, it will trigger an assertion failure, and by default, it will terminate with an error message indicating the failed assertion.
It’s important to note the following about assert()
:
assert()
is typically used during development and debugging to catch logical errors or unexpected conditions. In production code, assertions are often disabled to improve performance. This is usually achieved by defining theNDEBUG
macro before includingassert.h
in your production code.- Assertions should not be used for handling expected runtime errors or user inputs. Use proper error handling mechanisms (e.g., if statements, error codes, exceptions) for those cases.
- Assertions are a powerful tool for debugging, but they should not be relied upon as the sole method of error checking and validation in your programs.
- When using assertions, it’s essential to ensure that the conditions you assert are valid and represent logical expectations in your code.