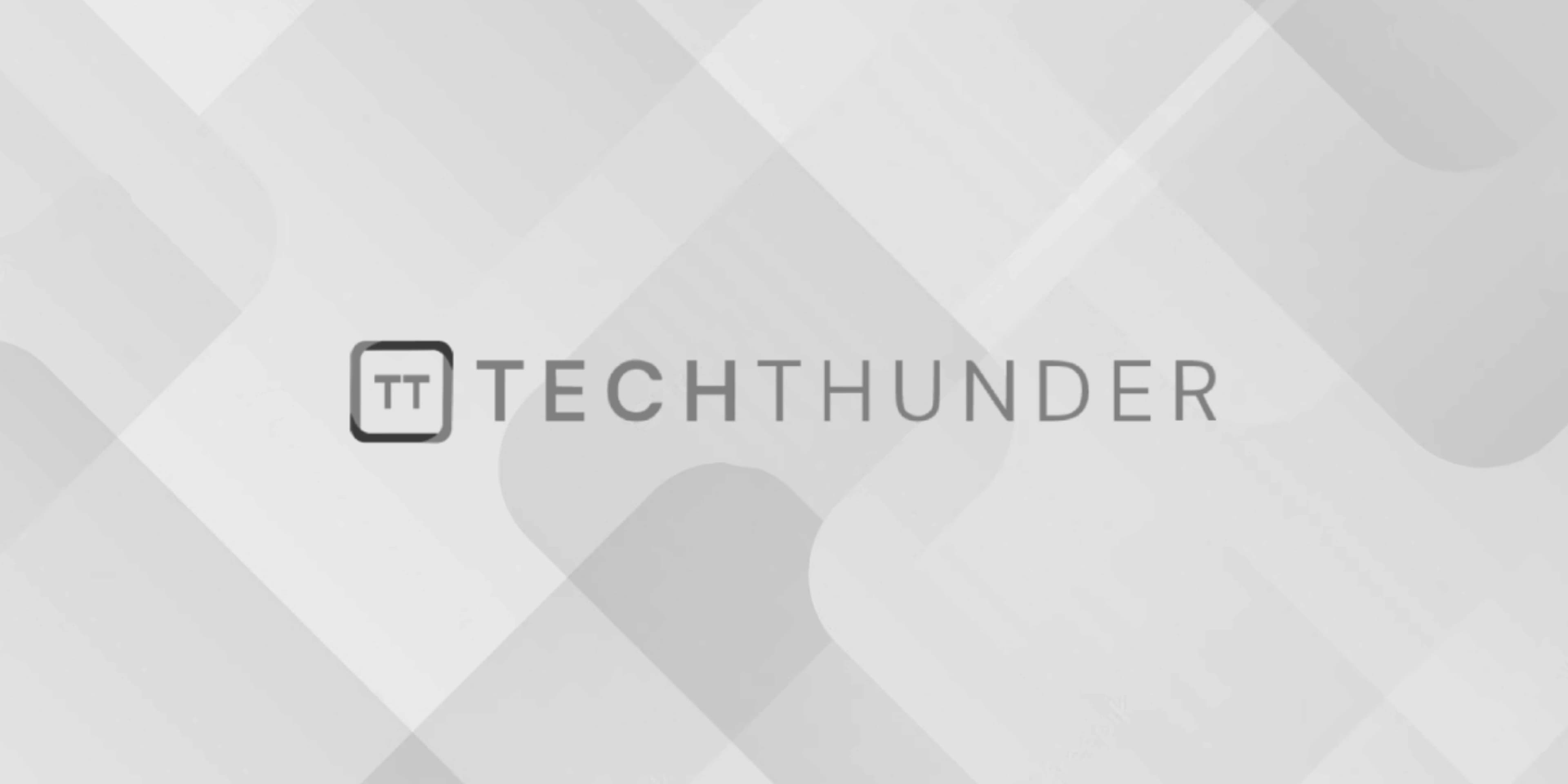
Factorial in C
To calculate the factorial of a number in C, you can use a simple loop or recursion. Here, I’ll provide you with examples of both methods:
Using a Loop (Iterative approach):
#include <stdio.h>
int main() {
int n;
unsigned long long factorial = 1; // Use unsigned long long to handle larger factorials
printf("Enter a non-negative integer: ");
scanf("%d", &n);
// Check for negative input
if (n < 0) {
printf("Factorial is not defined for negative numbers.\n");
} else {
// Calculate factorial
for (int i = 1; i <= n; i++) {
factorial *= i;
}
printf("Factorial of %d = %llu\n", n, factorial);
}
return 0;
}
In this program, we use a loop to calculate the factorial of the input number n
. We start with factorial
set to 1 and multiply it by numbers from 1 to n
to compute the factorial.
Using Recursion:
#include <stdio.h>
unsigned long long factorial(int n);
int main() {
int n;
unsigned long long fact;
printf("Enter a non-negative integer: ");
scanf("%d", &n);
if (n < 0) {
printf("Factorial is not defined for negative numbers.\n");
} else {
fact = factorial(n);
printf("Factorial of %d = %llu\n", n, fact);
}
return 0;
}
unsigned long long factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
In the recursive approach, we define a separate function factorial
that calls itself to compute the factorial of the input number n
. The base case is when n
is 0 or 1, in which case the factorial is 1. Otherwise, we recursively call the factorial
function with n-1
and multiply the result by n
to get the factorial.
Choose the method that suits your needs, and both approaches will calculate the factorial of a non-negative integer.