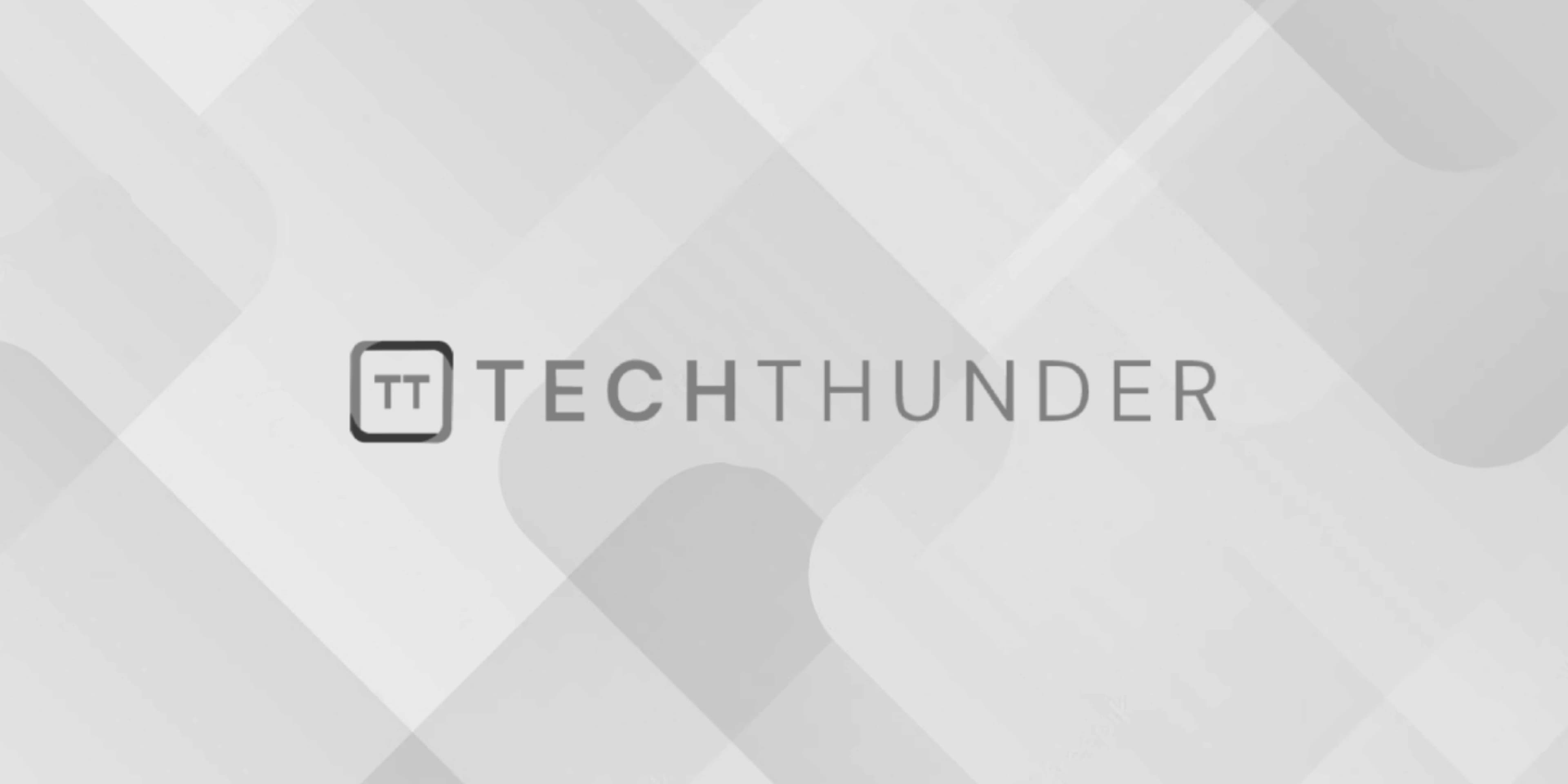
C Preprocessor Test
The C preprocessor is a part of the C compiler that performs text manipulations on your source code before actual compilation. It handles tasks like including header files, macro expansion, conditional compilation, and more.
To demonstrate the C preprocessor, let’s go through some common preprocessor directives and how they work:
- Include Files (
#include
):
The#include
directive is used to include the content of header files into your source code. For example:
#include <stdio.h>
This line includes the standard input/output library’s header file, which provides functions like printf
and scanf
.
- Define Macros (
#define
):
You can define macros using the#define
directive. Macros are used for text substitution. For example:
#define PI 3.14159265359
This defines a macro PI
that can be used throughout the code. Whenever the preprocessor encounters PI
, it replaces it with 3.14159265359
.
- Conditional Compilation (
#ifdef
,#ifndef
,#else
,#endif
):
Conditional compilation allows you to include or exclude parts of the code based on conditions. For example:
#ifdef DEBUG
// Code for debugging
#else
// Code for release
#endif
If the DEBUG
macro is defined, the debugging code will be included; otherwise, the release code will be included.
- Pragma Directives (
#pragma
):
The#pragma
directive provides special instructions to the compiler. For example:
#pragma pack(push, 1)
struct MyStruct {
int x;
char c;
};
#pragma pack(pop)
This directive controls the alignment of struct members in memory.
To see how the preprocessor modifies your code, you can use the -E
option with your C compiler to generate the preprocessed code. For example, with gcc
, you can run:
gcc -E your_program.c -o preprocessed_output.c
This will produce a file (preprocessed_output.c
) with the preprocessed code. You can then examine this file to see how the preprocessor has processed your code, including macro expansions and conditional code inclusion.
Testing and exploring the preprocessor’s behavior can help you understand how it works and how it can be used to manage code complexity and configuration in C programs.