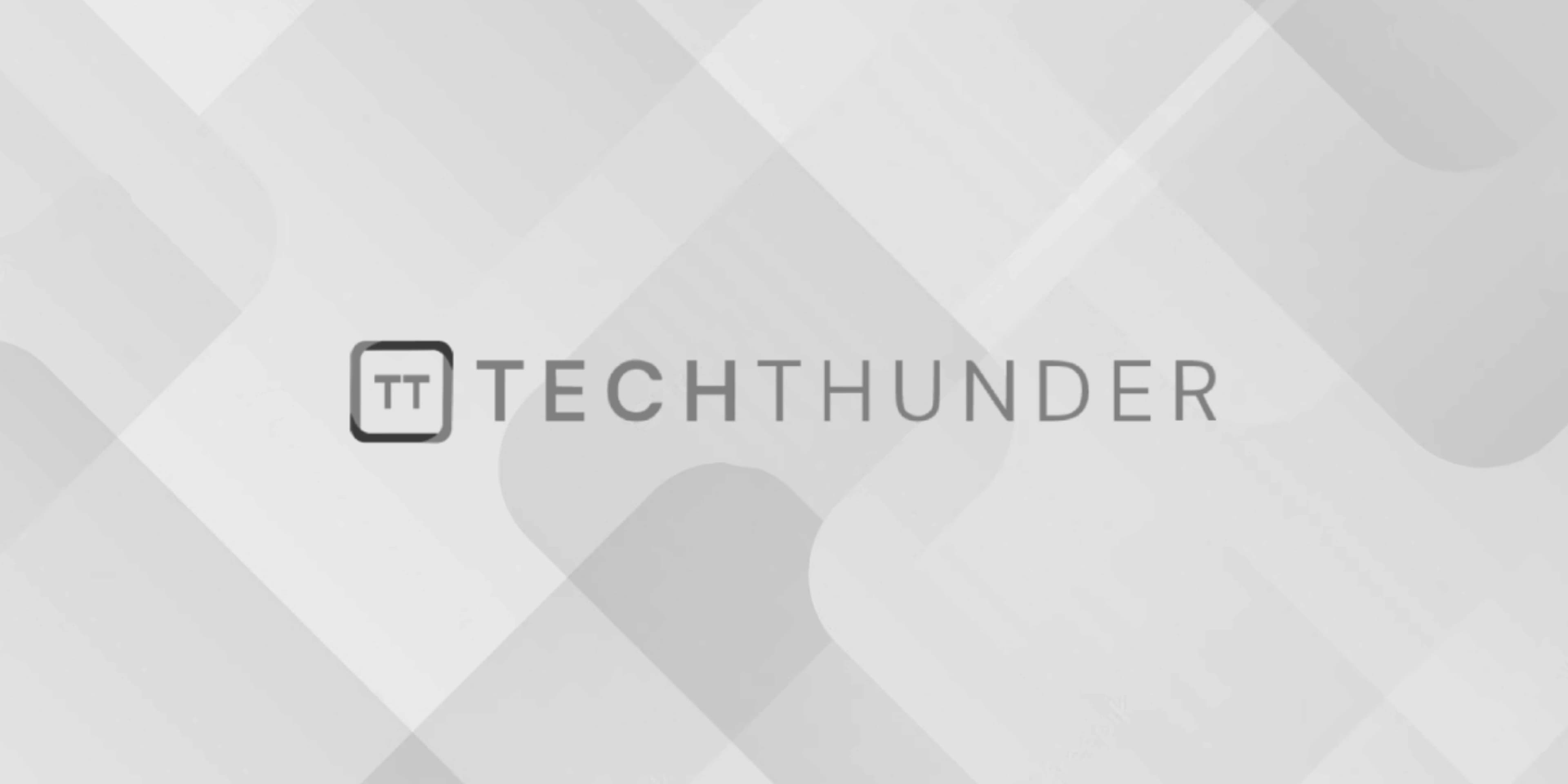
306 views
Data Types in C
The C programming, data types define the type of data that a variable can hold. C provides a range of data types to accommodate different types of data, from integers and floating-point numbers to characters and user-defined types. Here are some commonly used data types in C:
- Integers:
int
: Used for integer values.short
: Used for short integers (typically 16 bits).long
: Used for long integers (typically 32 bits).long long
: Used for very long integers (typically 64 bits).- Example:
C
int age = 30; long long population = 7000000000LL;
// Note the LL suffix for long long literals
- Floating-Point Numbers:
float
: Used for single-precision floating-point numbers.double
: Used for double-precision floating-point numbers (default for floating-point literals).long double
: Used for extended-precision floating-point numbers.- Example:
C
float pi = 3.14159f; // Note the f suffix for float literals
double e = 2.71828; // Double is the default for floating-point literal
- Characters:
char
: Used for single characters.- Example:
C
char grade = 'A';
- Boolean:
_Bool
(orbool
after including<stdbool.h>
): Used for Boolean values (true or false).- Example (with
stdbool.h
):
C
#include <stdbool.h>
bool isStudent = true;
- Enumerations (Enums):
enum
: Used to define a user-defined data type that represents a set of named integer constants.- Example:
C
enum Color {
RED, GREEN, BLUE
};
enum Color selectedColor = GREEN;
- Void:
void
: Typically used as a return type for functions that do not return a value.- Example:
C
void printMessage() {
printf("Hello, world!\n");
}
- Pointers:
int*
: Pointer to an integer.float*
: Pointer to a float.char*
: Pointer to a character (often used for strings).- Example:
C
c int num = 42;
int* ptr = # // Pointer to an integer
- Arrays:
int[]
: Array of integers.char[]
: Array of characters (strings are represented as character arrays).- Example:
C
int numbers[5] = {1, 2, 3, 4, 5};
char name[] = "John";
- User-Defined Types:
struct
: Used to define user-defined composite data types (structures).union
: Used to define unions, which can hold one value from a set of possible types.- Example:
C
struct Person {
char name[50]; int age;
};
union Data {
int intValue;
float floatValue;
};
These are some of the fundamental data types in C. C also allows for type modifiers, such as signed
, unsigned
, const
, and others, to further refine the behavior of these basic data types. Understanding data types is essential for declaring variables and specifying the type of data they will hold.