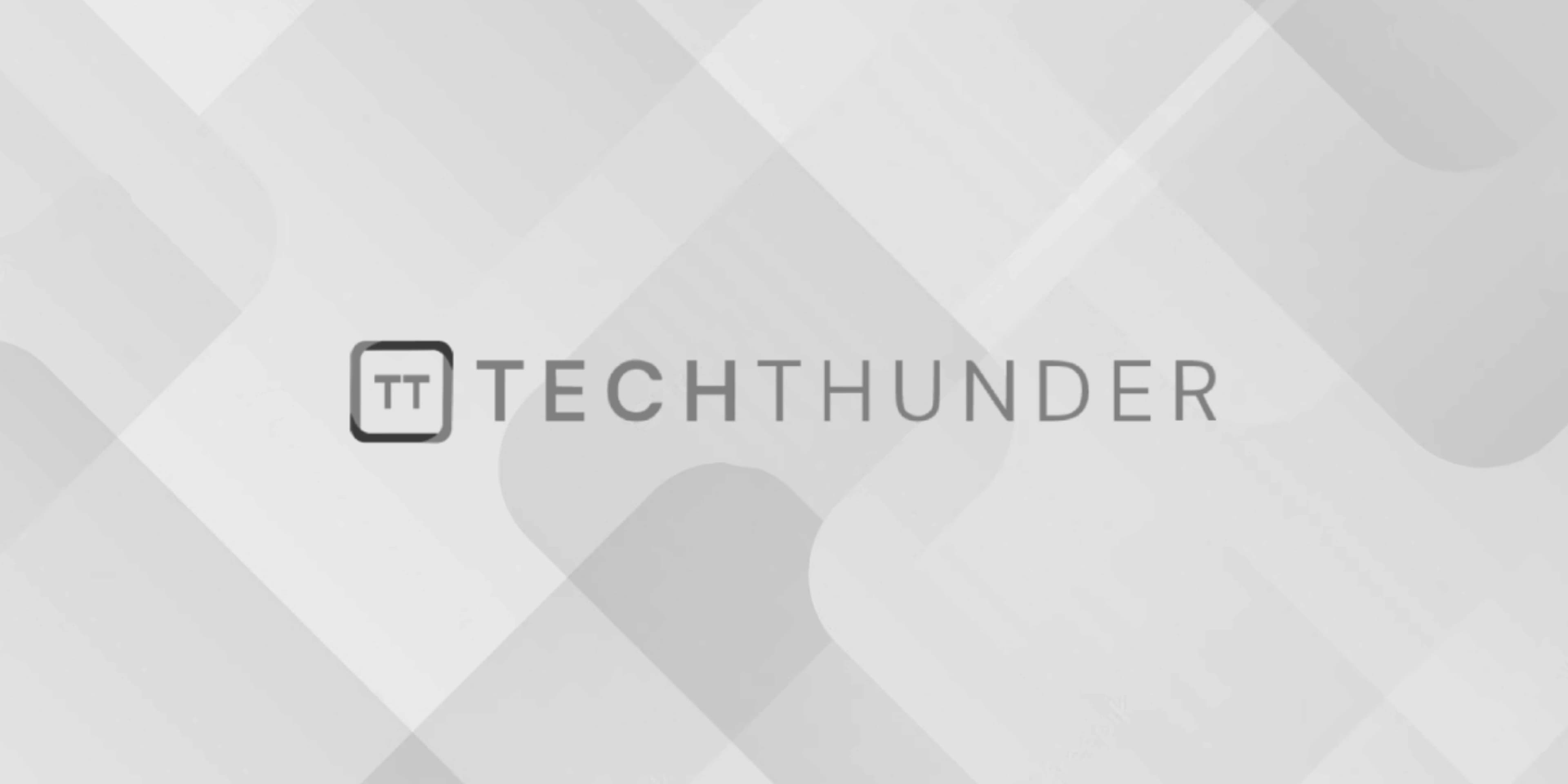
typedef in C
The typedef
is a keyword used to create an alias (alternate name) for a data type. It allows you to define custom type names, which can make your code more readable, maintainable, and portable. typedef
is often used with structures, unions, enums, and primitive data types.
The basic syntax of typedef
is as follows:
typedef existing_data_type new_data_type_name;
Here’s how you can use typedef
with different types of data:
- Primitive Data Types:
typedef int myInteger; // Create an alias for int
typedef double myDouble; // Create an alias for double
myInteger x = 42;
myDouble y = 3.14;
- Structures:
typedef struct {
int age;
char name[50];
} Person;
Person person1;
person1.age = 30;
strcpy(person1.name, "John Doe");
- Enums:
typedef enum {
RED,
GREEN,
BLUE
} Color;
Color favoriteColor = GREEN;
- Pointers to Functions:
typedef int (*MathFunction)(int, int);
int add(int a, int b) {
return a + b;
}
MathFunction operation = add;
int result = operation(5, 3); // Calls the 'add' function
Using typedef
can make your code more self-explanatory and can help abstract away complex data types. For example, instead of declaring variables with types like struct MyStruct
, you can use a typedef
to create a more meaningful and concise alias like MyStruct
.
Here’s an example demonstrating the use of typedef
with structures:
#include <stdio.h>
typedef struct {
int x;
int y;
} Point;
int main() {
Point p1 = {10, 20};
Point p2 = {5, 15};
printf("Point 1: x = %d, y = %d\n", p1.x, p1.y);
printf("Point 2: x = %d, y = %d\n", p2.x, p2.y);
return 0;
}
In this example, Point
is created as an alias for the struct
with two integer members, x
and y
. This makes the code more readable and allows you to work with Point
objects instead of the more verbose struct
declarations.