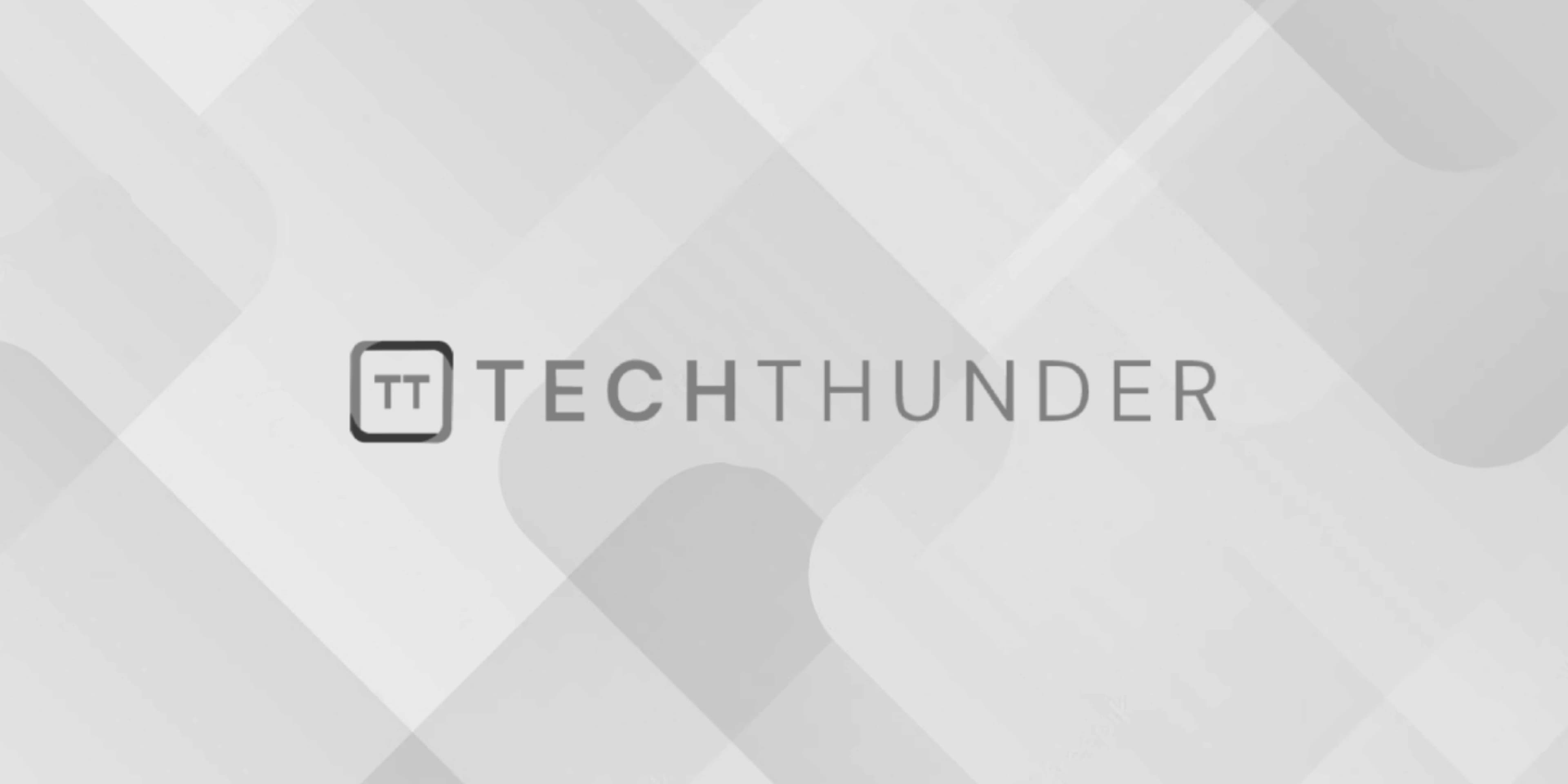
Static in C
The static
keyword has multiple uses and meanings, depending on where and how it is applied. It can be used in different contexts to specify storage duration, scope, and linkage of variables and functions. Here are some common uses of the static
keyword in C:
- Static Variables:
- When
static
is used with a variable declared within a function (local variable), it changes the storage duration of the variable. - A
static
local variable retains its value between function calls. It is initialized only once when the program starts, and its value persists across multiple calls to the function.
#include <stdio.h>
void foo() {
static int count = 0; // Static local variable
count++;
printf("Count: %d\n", count);
}
int main() {
foo(); // Count: 1
foo(); // Count: 2
foo(); // Count: 3
return 0;
}
- Static Functions:
- When
static
is used to declare a function, it specifies that the function has internal linkage, meaning it is only visible and accessible within the current source file. Other source files cannot access the static function.
// File1.c
static void static_function() {
// Function implementation
}
In this example, static_function
is only accessible within File1.c
and cannot be used in other source files.
- Static Global Variables:
- When
static
is used with a global variable, it specifies that the variable has internal linkage, similar to static functions. The variable is only accessible within the current source file.
// File1.c
static int static_global_var = 42; // Static global variable
In this case, static_global_var
is limited to File1.c
and cannot be accessed from other source files.
- Static in Header Files:
- When
static
is used in a function prototype or variable declaration within a header file, it limits the visibility of that function or variable to the source file that includes the header. This is a way to achieve encapsulation and avoid naming conflicts in larger projects.
// Header.h
static void static_function_prototype();
The static_function_prototype
can only be used in source files that include Header.h
.
- Static Labels (Goto Labels):
- The
static
keyword can be used to declare labels forgoto
statements. This makes the label visible only within the function or block where it is declared.
void foo() {
static label1: // Static label
// Code
goto label1; // Jump to the static label
}
- Static Members in Structs and Unions:
- When
static
is used with a member of astruct
orunion
, it indicates that the member is shared among all instances of the struct or union, rather than having a separate instance for each.
struct Point {
int x;
int y;
static int instance_count; // Static member shared among all Point instances
};
The instance_count
is shared by all Point
instances.
The static
keyword has various uses in C, and its meaning can change based on context. It is a powerful tool for controlling the scope, storage duration, and linkage of variables and functions in C programs.