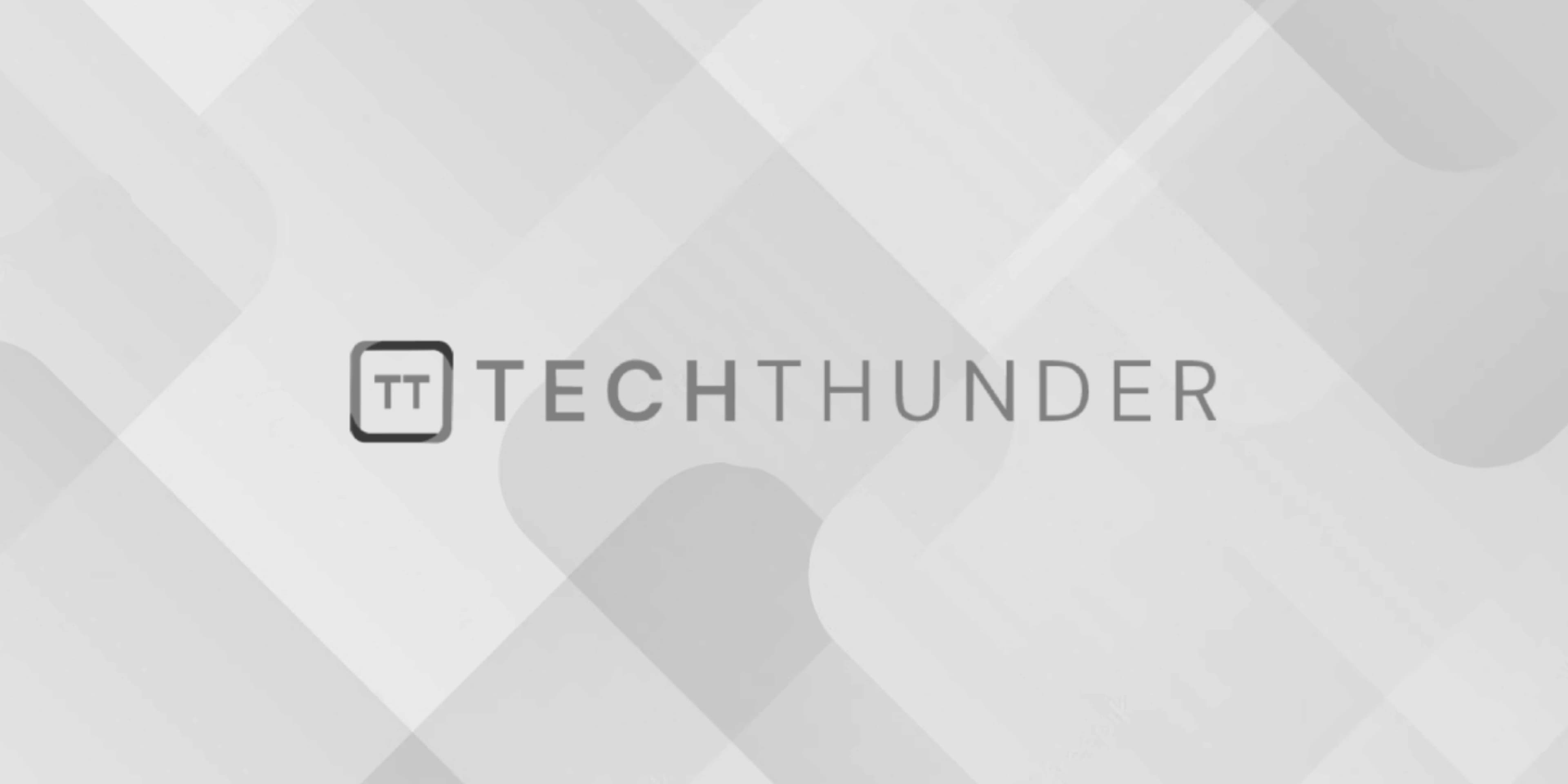
Modulus Operator in C
The modulus operator is represented by the %
symbol. It is used to find the remainder when one integer is divided by another. The modulus operator is particularly useful when you want to work with the remainder of a division operation.
Here’s the basic syntax of the modulus operator:
result = dividend % divisor;
dividend
: The number you want to find the remainder of (the numerator).divisor
: The number you are dividing by (the denominator).result
: The remainder after dividingdividend
bydivisor
.
Here are a few examples of how the modulus operator is used:
#include <stdio.h>
int main() {
int dividend = 10;
int divisor = 3;
int result;
// Calculate the remainder
result = dividend % divisor;
printf("The remainder of %d divided by %d is %d\n", dividend, divisor, result);
return 0;
}
In this example, dividend
is 10, divisor
is 3, and result
will be assigned the value 1 because 10 divided by 3 is 3 with a remainder of 1.
Here’s another example:
#include <stdio.h>
int main() {
int dividend = 17;
int divisor = 5;
int result;
// Calculate the remainder
result = dividend % divisor;
printf("The remainder of %d divided by %d is %d\n", dividend, divisor, result);
return 0;
}
In this case, dividend
is 17, divisor
is 5, and result
will be assigned the value 2 because 17 divided by 5 is 3 with a remainder of 2.
The modulus operator is frequently used in programming for various purposes, including checking for even or odd numbers, performing cyclic calculations, and finding patterns in data.