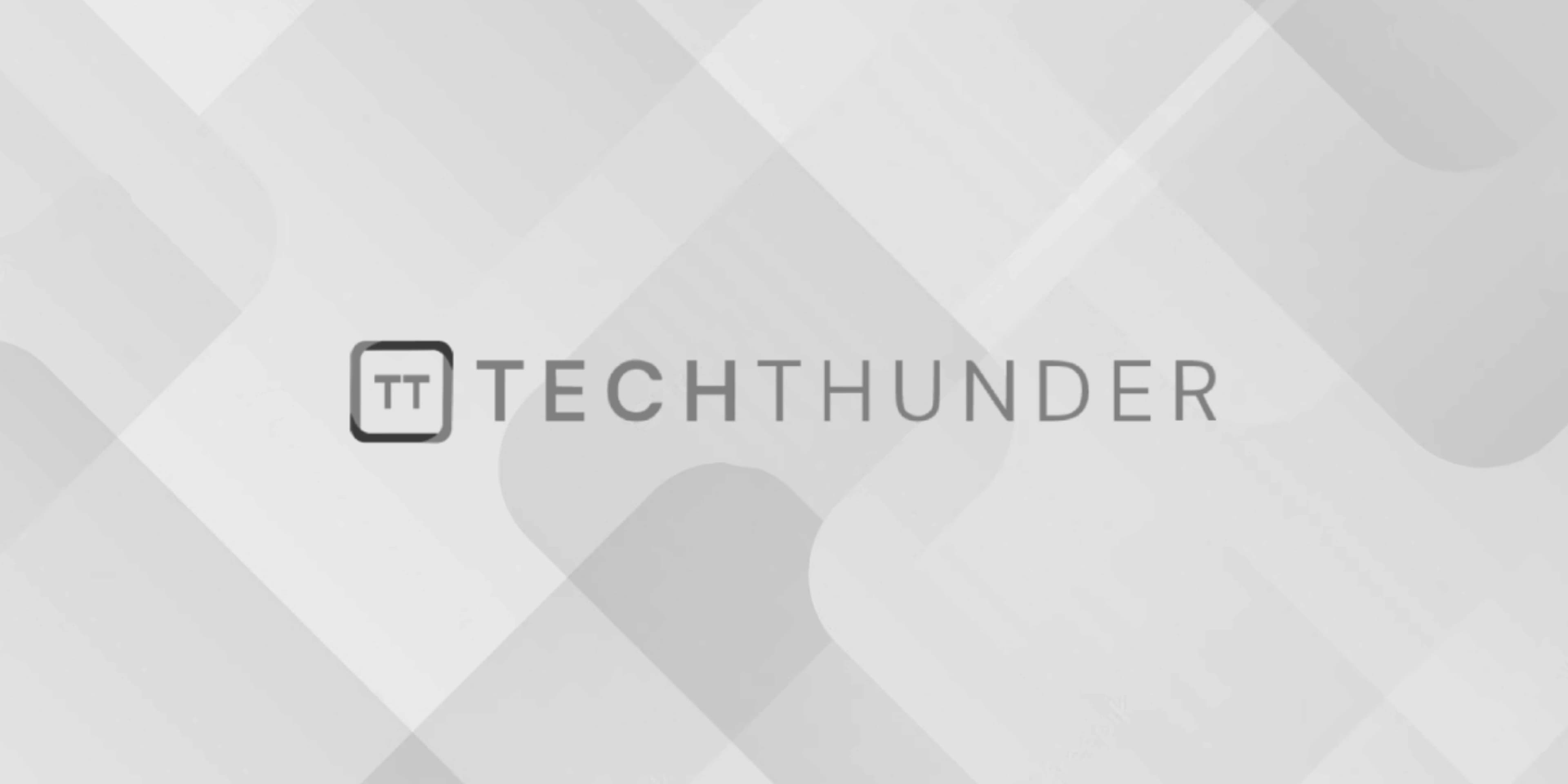
291 views
Fibonacci Series in C
The C program to generate the Fibonacci series up to a specified number of terms:
C
#include <stdio.h>
int main() {
int n, term1 = 0, term2 = 1, nextTerm;
printf("Enter the number of terms: ");
scanf("%d", &n);
printf("Fibonacci Series: ");
for (int i = 1; i <= n; i++) {
// Print the current term
printf("%d, ", term1);
// Calculate the next term
nextTerm = term1 + term2;
// Update terms for the next iteration
term1 = term2;
term2 = nextTerm;
}
printf("\n");
return 0;
}
In this program:
- We first prompt the user to enter the number of terms (
n
) they want in the Fibonacci series. - We initialize
term1
andterm2
to 0 and 1, respectively, as these are the first two terms of the Fibonacci sequence. - Inside the loop, we print
term1
, which is the current term of the series. - We calculate the next term (
nextTerm
) by addingterm1
andterm2
. - We then update
term1
andterm2
for the next iteration by assigningterm2
toterm1
andnextTerm
toterm2
. - The loop continues until we have printed the specified number of terms.
When you run this program and enter the number of terms you want, it will generate and display the Fibonacci series up to the specified number of terms.