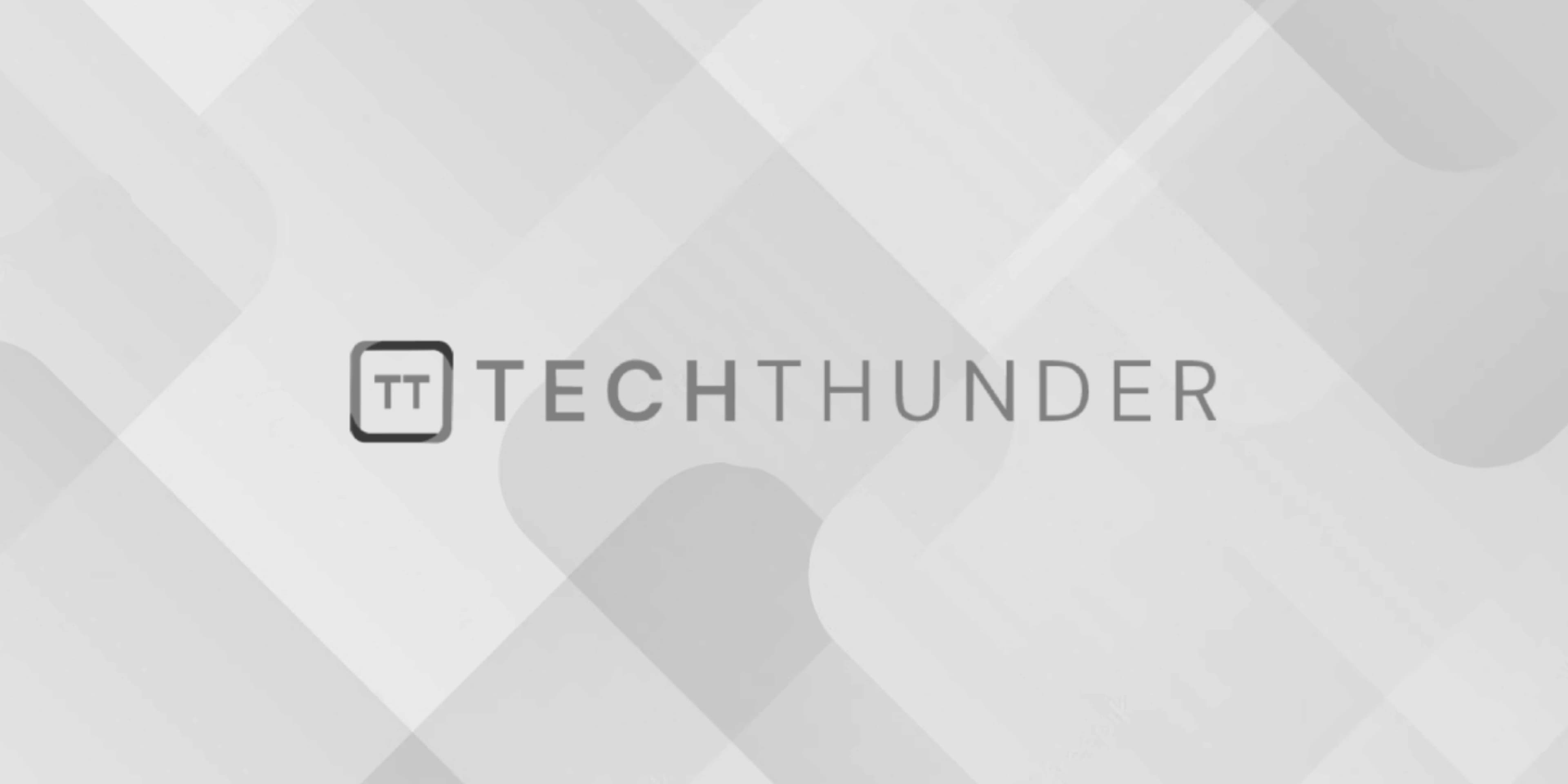
C #if
The #if
directive is used for conditional compilation. It allows you to include or exclude portions of code from compilation based on specific conditions that you specify. The #if
directive checks whether a given expression evaluates to true or false, and if it evaluates to true, the code within the #if
block is included in the compilation.
Here’s the basic syntax of the #if
directive:
#if expression
// Code to compile if the expression is true
#endif
expression
is a C expression that is evaluated by the preprocessor. If the expression is nonzero (true), the code within the#if
block is compiled. If the expression is zero (false), the code within the#if
block is excluded from compilation.
Here’s an example of how the #if
directive can be used:
#include <stdio.h>
#define DEBUG 1 // Define DEBUG macro
int main() {
#if DEBUG
printf("Debug mode is enabled.\n");
#else
printf("Debug mode is disabled.\n");
#endif
return 0;
}
In this example:
- The
DEBUG
macro is defined with a value of1
using#define
. This is often used to enable debug code when needed. - Inside the program, the
#if
directive checks whether theDEBUG
macro evaluates to true (nonzero). Since it is defined as1
, the expression is true, and the code within the#if
block is included in compilation. - As a result, “Debug mode is enabled.” is printed when the program is executed.
You can use #if
to conditionally compile code based on various conditions, including macro definitions, expressions, or predefined macros that the preprocessor recognizes.
Here’s an example that uses predefined macros to check the target platform:
#include <stdio.h>
int main() {
#if defined(_WIN32)
printf("Running on Windows.\n");
#else
printf("Running on a non-Windows platform.\n");
#endif
return 0;
}
In this case, the defined(_WIN32)
expression checks if the _WIN32
predefined macro is defined, which is typically the case when compiling for Windows platforms. Depending on the platform, it prints a corresponding message.