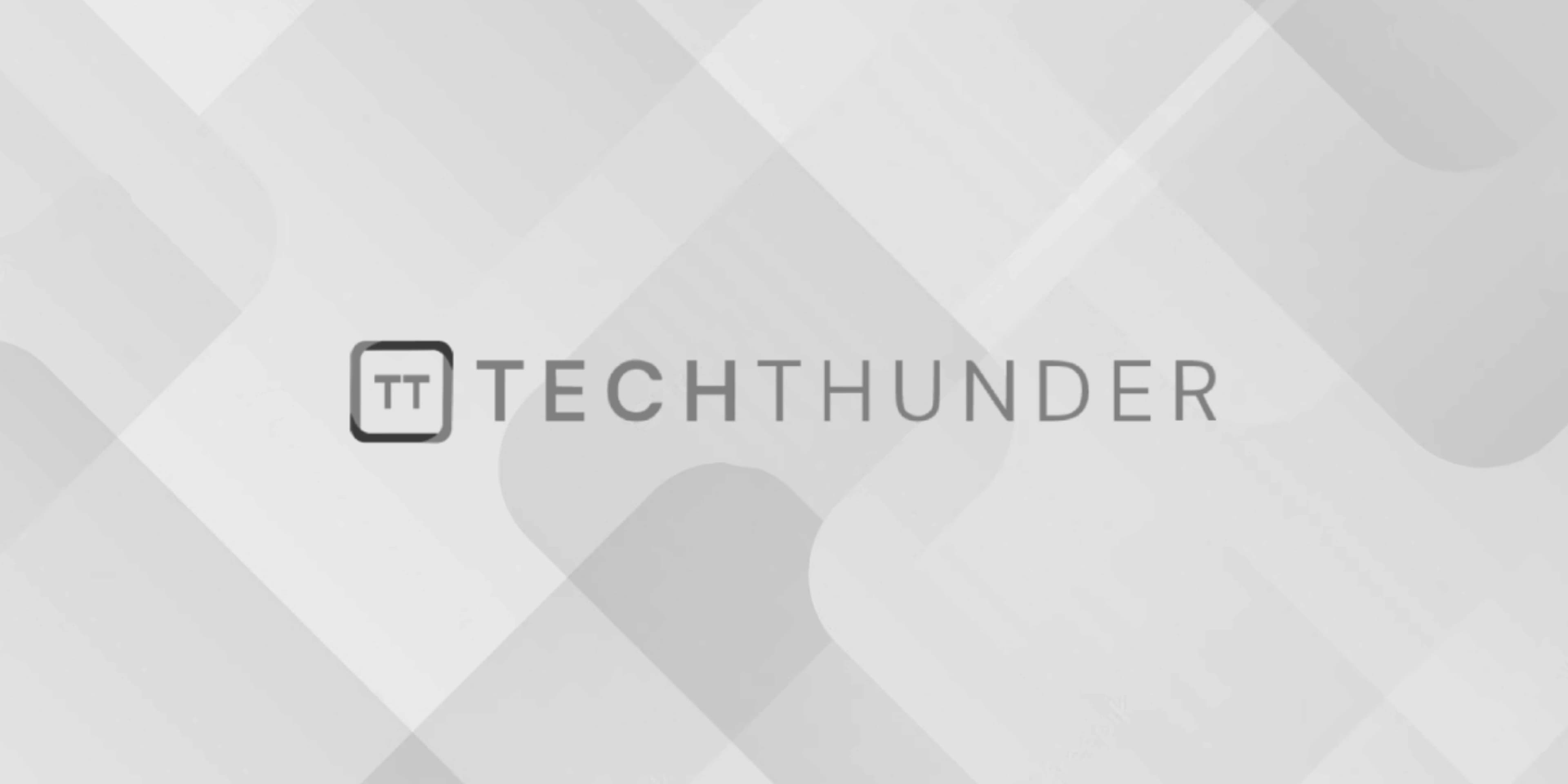
249 views
while loop vs do-while loop in C
The both while
loops and do-while
loops are used for repetitive tasks where you want to execute a block of code multiple times as long as a certain condition is met. However, they have different behaviors and use cases:
while
Loop:
- In a
while
loop, the condition is checked at the beginning (before entering the loop). If the condition is false initially, the loop may not execute at all. - It’s often referred to as an “entry-controlled” loop because the condition is evaluated before the loop body is executed.
- If the condition is false, the loop body is skipped, and the program continues with the code after the loop.
- Here’s the basic syntax of a
while
loop:while (condition) { // Code to be executed while the condition is true }
- Example:
int i = 1; while (i <= 5) { printf("%d ", i); i++; }
Thiswhile
loop will print numbers from 1 to 5.
do-while
Loop:
- In a
do-while
loop, the condition is checked at the end (after executing the loop body at least once). This guarantees that the loop body will execute at least once, even if the condition is initially false. - It’s often referred to as an “exit-controlled” loop because the condition is evaluated after the loop body is executed.
- The
do-while
loop has the following syntax:do { // Code to be executed at least once } while (condition);
- Example:
int i = 1; do { printf("%d ", i); i++; } while (i <= 5);
Thisdo-while
loop will also print numbers from 1 to 5.
Here are some considerations to help you decide when to use which loop:
- Use a
while
loop when you want to execute a block of code zero or more times based on a condition. If the condition is false initially, the loop will not execute. - Use a
do-while
loop when you want to execute a block of code one or more times, and you want to guarantee that the loop body is executed at least once, regardless of the initial condition. - If you’re unsure which loop to use, consider whether you need the loop body to run at least once. If yes, use
do-while
. Otherwise, use awhile
loop.
Both types of loops have their own use cases, and the choice between them depends on the specific requirements of your program.