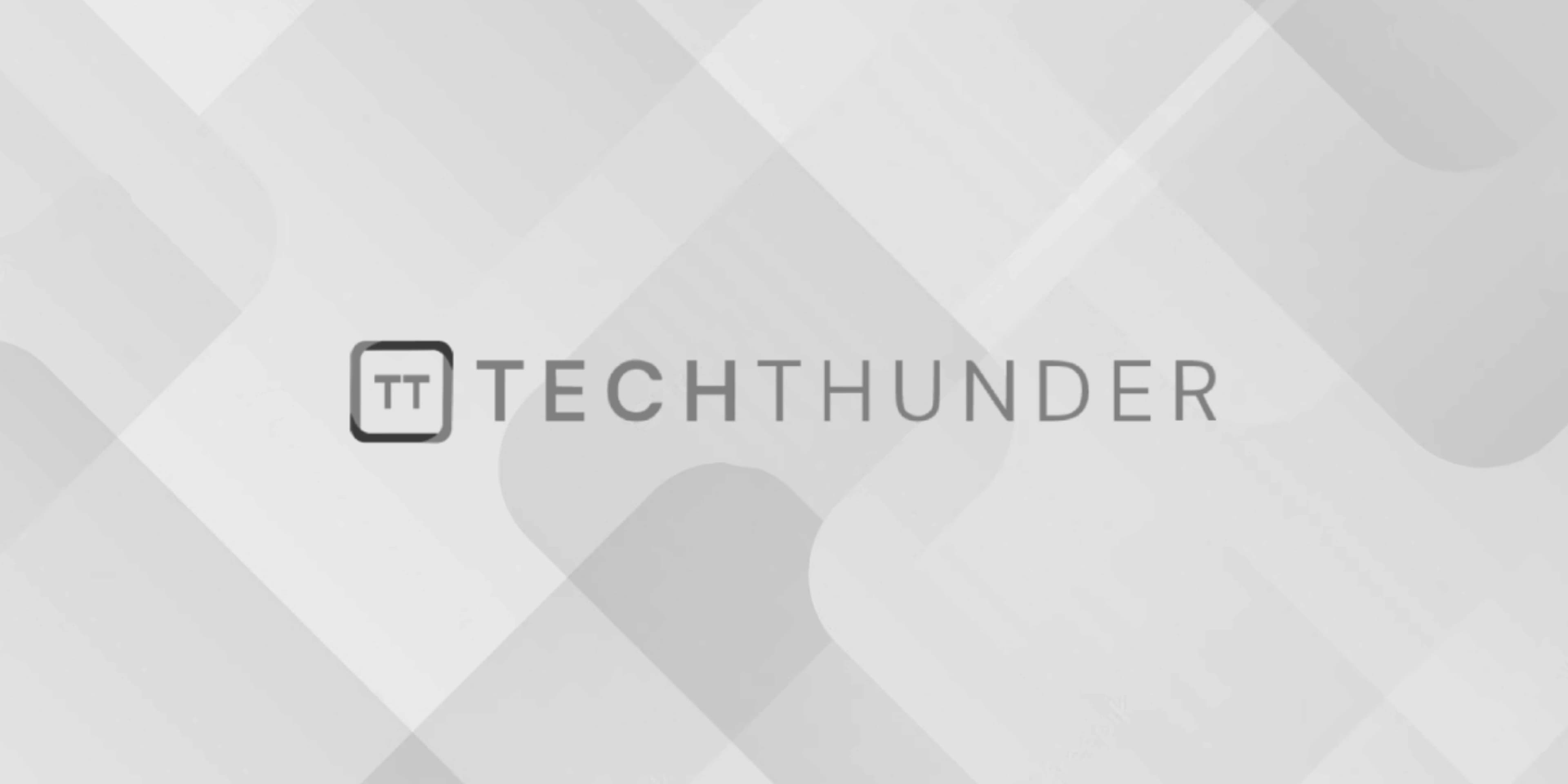
269 views
3 way merge sort in C
Three-way merge sort is an extension of the traditional merge sort algorithm, where instead of dividing the array into two subarrays, it divides the array into three subarrays and recursively sorts them. Once the subarrays are sorted, they are merged together to produce the final sorted array. Here’s a C implementation of the three-way merge sort algorithm:
C
#include <stdio.h>
#include <stdlib.h>
// Merge three sorted subarrays into one sorted array
void merge(int arr[], int left[], int middle[], int right[], int n1, int n2, int n3) {
int i = 0, j = 0, k = 0, l = 0;
while (i < n1 && j < n2 && k < n3) {
if (left[i] <= middle[j] && left[i] <= right[k]) {
arr[l++] = left[i++];
} else if (middle[j] <= left[i] && middle[j] <= right[k]) {
arr[l++] = middle[j++];
} else {
arr[l++] = right[k++];
}
}
while (i < n1) {
arr[l++] = left[i++];
}
while (j < n2) {
arr[l++] = middle[j++];
}
while (k < n3) {
arr[l++] = right[k++];
}
}
// Recursive three-way merge sort function
void mergeSort(int arr[], int n) {
if (n > 1) {
int n1 = n / 3;
int n2 = (2 * n) / 3;
int n3 = n - n1 - n2;
int left[n1];
int middle[n2];
int right[n3];
for (int i = 0; i < n1; i++) {
left[i] = arr[i];
}
for (int i = 0; i < n2; i++) {
middle[i] = arr[i + n1];
}
for (int i = 0; i < n3; i++) {
right[i] = arr[i + n1 + n2];
}
mergeSort(left, n1);
mergeSort(middle, n2);
mergeSort(right, n3);
merge(arr, left, middle, right, n1, n2, n3);
}
}
int main() {
int arr[] = {12, 11, 13, 5, 6, 7, 3, 8, 10, 9};
int n = sizeof(arr) / sizeof(arr[0]);
printf("Original array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
mergeSort(arr, n);
printf("Sorted array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
In this program:
- The
merge
function takes three sorted subarrays (left
,middle
, andright
) and their sizes (n1
,n2
, andn3
) and merges them into a single sorted array (arr
). - The
mergeSort
function is a recursive function that divides the input array into three subarrays, sorts them recursively, and then merges them using themerge
function. - In the
main
function, we demonstrate the three-way merge sort by initializing an array, callingmergeSort
, and then printing the sorted array.
This code implements a basic three-way merge sort algorithm for educational purposes. In practice, you may want to consider optimizations and error handling for different input scenarios.