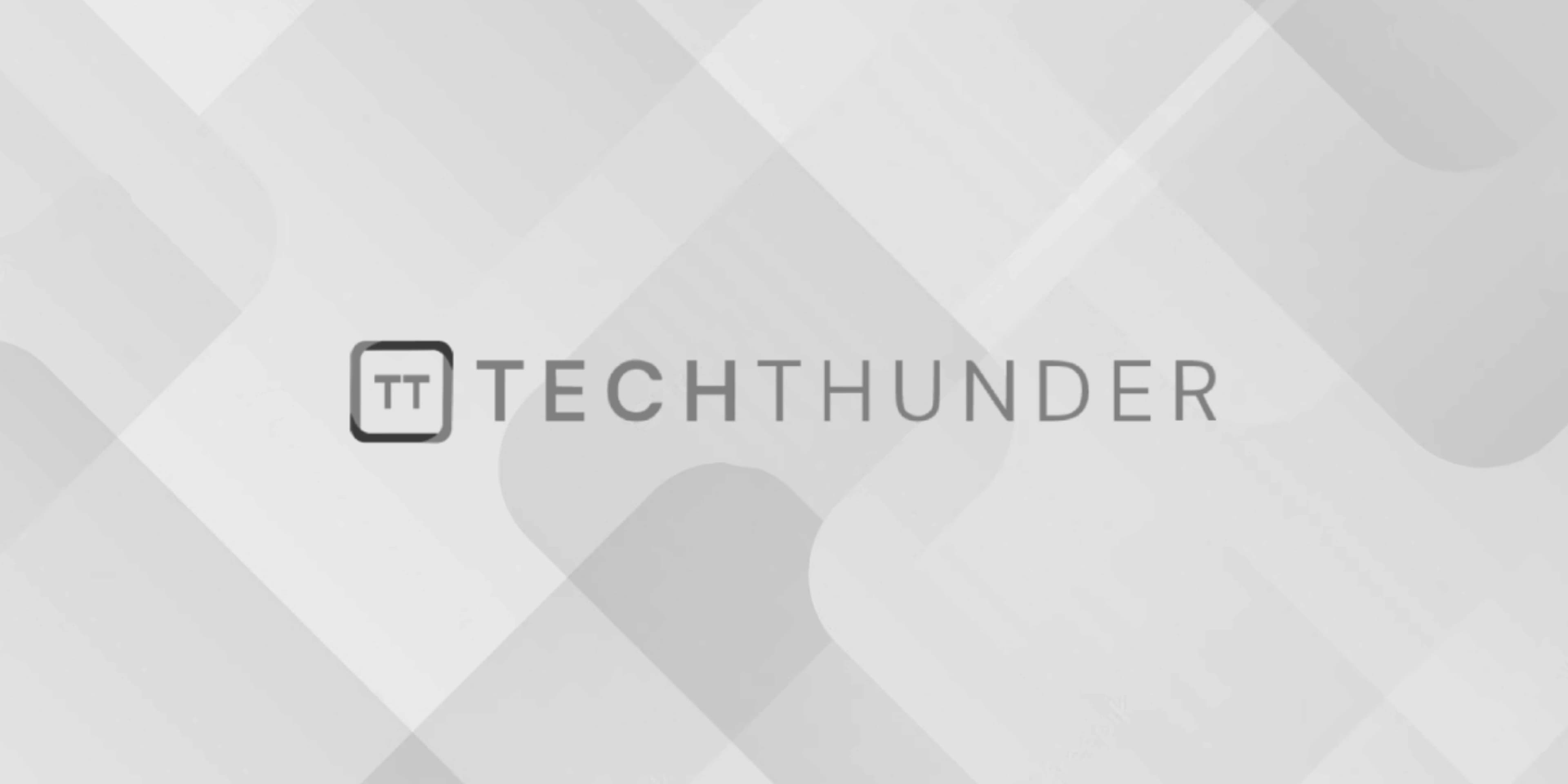
Shift Operators in C
Shift operators in C are binary operators used to perform bit-level manipulation of integers. There are two shift operators in C:
- Left Shift Operator (
<<
): This operator shifts the bits of a number to the left by a specified number of positions. It effectively multiplies the number by 2 raised to the power of the shift count. The syntax is:
result = operand << shift_count;
Example:
int x = 5; // binary: 0000 0101
int y = x << 2; // binary: 0001 0100 (20 in decimal)
- Right Shift Operator (
>>
): This operator shifts the bits of a number to the right by a specified number of positions. For signed integers, it is an arithmetic shift (the sign bit is extended), while for unsigned integers, it is a logical shift (0s are filled on the left). The syntax is:
result = operand >> shift_count;
Example:
int x = 20; // binary: 0001 0100
int y = x >> 2; // binary: 0000 0101 (5 in decimal)
Here’s a summary of how these operators work:
- Left shifting a number by
n
positions is equivalent to multiplying it by 2^n. - Right shifting a number by
n
positions is equivalent to dividing it by 2^n.
It’s important to be cautious when using right shifts with signed integers. The behavior of right-shifting a negative number is implementation-defined in C, which means it can vary between compilers. In practice, most compilers use arithmetic right shifts for signed integers, meaning the sign bit is extended when shifting right.
Here’s an example illustrating the potential difference with signed integers:
int x = -8; // binary: 1111 1000 (assuming 8-bit two's complement)
int y = x >> 1; // Implementation-defined result
The result of y
could be either -4
(if it uses an arithmetic shift) or 124
(if it uses a logical shift), depending on the compiler and platform. To avoid such ambiguity, it’s recommended to use unsigned integers when you want a strictly logical right shift.