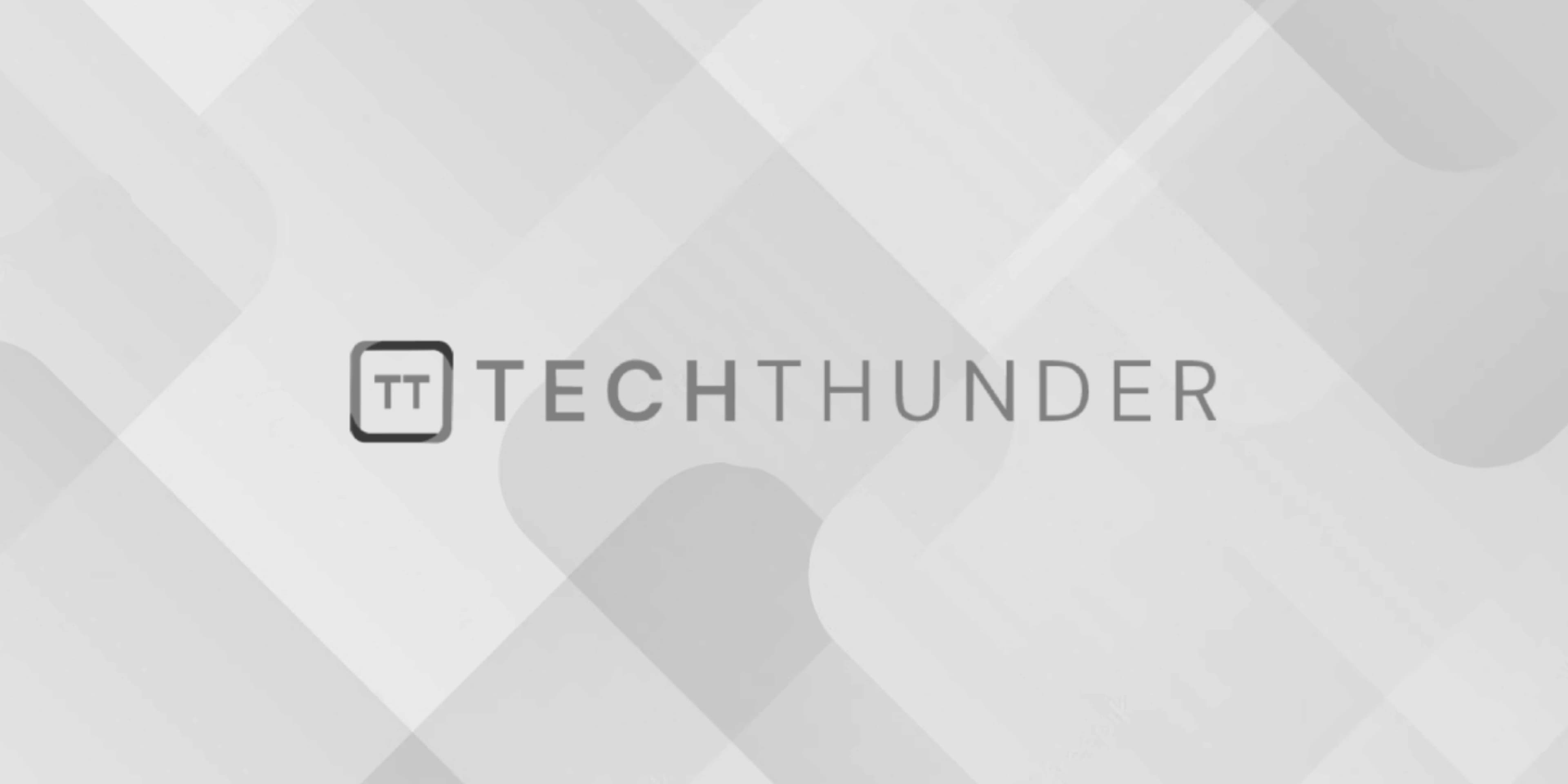
272 views
C File Handling
File handling in C involves reading and writing data to and from files. The standard library in C provides functions and data types to work with files. Here’s an overview of file handling in C.
Opening a File:
- To work with a file, you first need to open it using the
fopen()
function. This function takes two arguments: the file name (including the path) and the mode in which you want to open the file. - Common modes include
"r"
for reading,"w"
for writing (creates a new file or overwrites an existing one),"a"
for appending (adds data to the end of an existing file),"rb"
and"wb"
for binary reading and writing, and more. - Example:
C
#include <stdio.h>
int main() {
FILE *file = fopen("example.txt", "w");
if (file == NULL) {
perror("Error opening the file");
return 1;
}
// Your code here
fclose(file);
return 0;
}
Reading from a File:
- You can read data from a file using functions like
fread()
,fgets()
, orfscanf()
. - Example using
fgets()
:
C
#include <stdio.h>
// Assuming 'file' is declared and opened somewhere in the code
char buffer[100];
if (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("Data read from the file: %s\n", buffer);
}
Writing to a File:
- You can write data to a file using functions like
fwrite()
,fputs()
, orfprintf()
. - Example using
fprintf()
:
C
c fprintf(file, "This is a line of text.\n");
Closing a File:
- It’s essential to close the file when you’re done using it to free up system resources and ensure that data is written to the file.
- Use the
fclose()
function to close the file. - Example:
C
c fclose(file);
Checking for Successful Operations:
- After opening, reading, or writing to a file, it’s a good practice to check if the operation was successful.
- Most file-related functions return
NULL
or a specific value on failure. - Use
feof()
to check for the end of the file. - Example:
C
c if (feof(file)) {
printf("End of file reached.\n");
}
Error Handling:
- Handle errors that may occur during file operations using functions like
perror()
or by checking the return values of file-related functions. - Example:
C
c if (file == NULL) {
perror("Error opening the file");
return 1;
}
Binary File Handling:
- For binary file handling, use
"rb"
and"wb"
modes and functions likefread()
andfwrite()
to read and write binary data.
Here’s a simple example that demonstrates file handling by writing and reading a line of text to/from a file:
C
#include <stdio.h>
int main() {
FILE *file = fopen("example.txt", "w");
if (file == NULL) {
perror("Error opening the file");
return 1;
}
fprintf(file, "This is a line of text.\n");
fclose(file);
file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening the file");
return 1;
}
char buffer[100];
if (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("Data read from the file: %s", buffer);
}
fclose(file);
return 0;
}
This program writes a line of text to “example.txt,” closes the file, and then reads and prints the same line of text from the file.