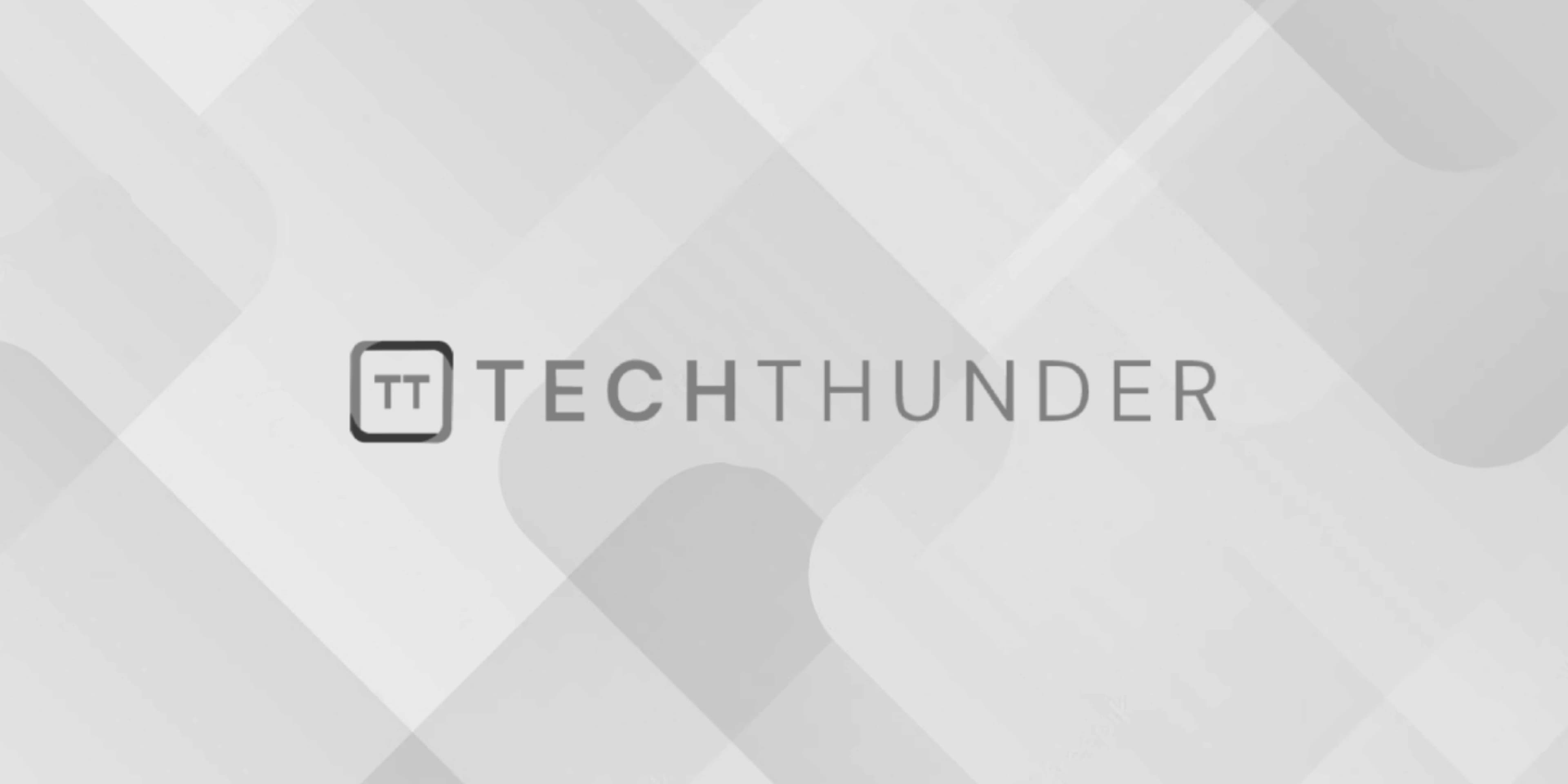
The exit() function in C
The exit()
function in C is used to terminate a program and return an exit status to the operating system or the calling process. When a program calls exit()
, it will immediately terminate, and control returns to the operating system. The exit status code provided to exit()
is a way for the program to communicate its termination status to the calling environment.
Here’s the function prototype for exit()
:
void exit(int status);
status
: An integer value that represents the exit status code. This status code is returned to the operating system or the parent process. Conventionally, a status code of 0 indicates success, while non-zero values are used to indicate various error conditions or other exit statuses.
Here’s an example of how to use the exit()
function in a C program:
#include <stdio.h>
#include <stdlib.h>
int main() {
int result = performSomeOperation();
if (result == 0) {
printf("Operation succeeded.\n");
exit(0); // Exit with a status code of 0 (success)
} else {
printf("Operation failed.\n");
exit(1); // Exit with a status code of 1 (failure)
}
}
In this example, the program performs some operation (represented by the performSomeOperation()
function) and checks the result. Depending on the result, it calls exit()
with an appropriate status code. In this case, a status code of 0 indicates success, and a status code of 1 indicates failure.
When the program exits, the status code is returned to the calling environment. This status code can be used by shell scripts or other programs to determine the outcome of the executed program.
It’s important to note that after calling exit()
, no further code in the program will be executed, and the program will terminate immediately. Therefore, it’s typically used when a program needs to exit gracefully or when it encounters a specific condition that requires termination.