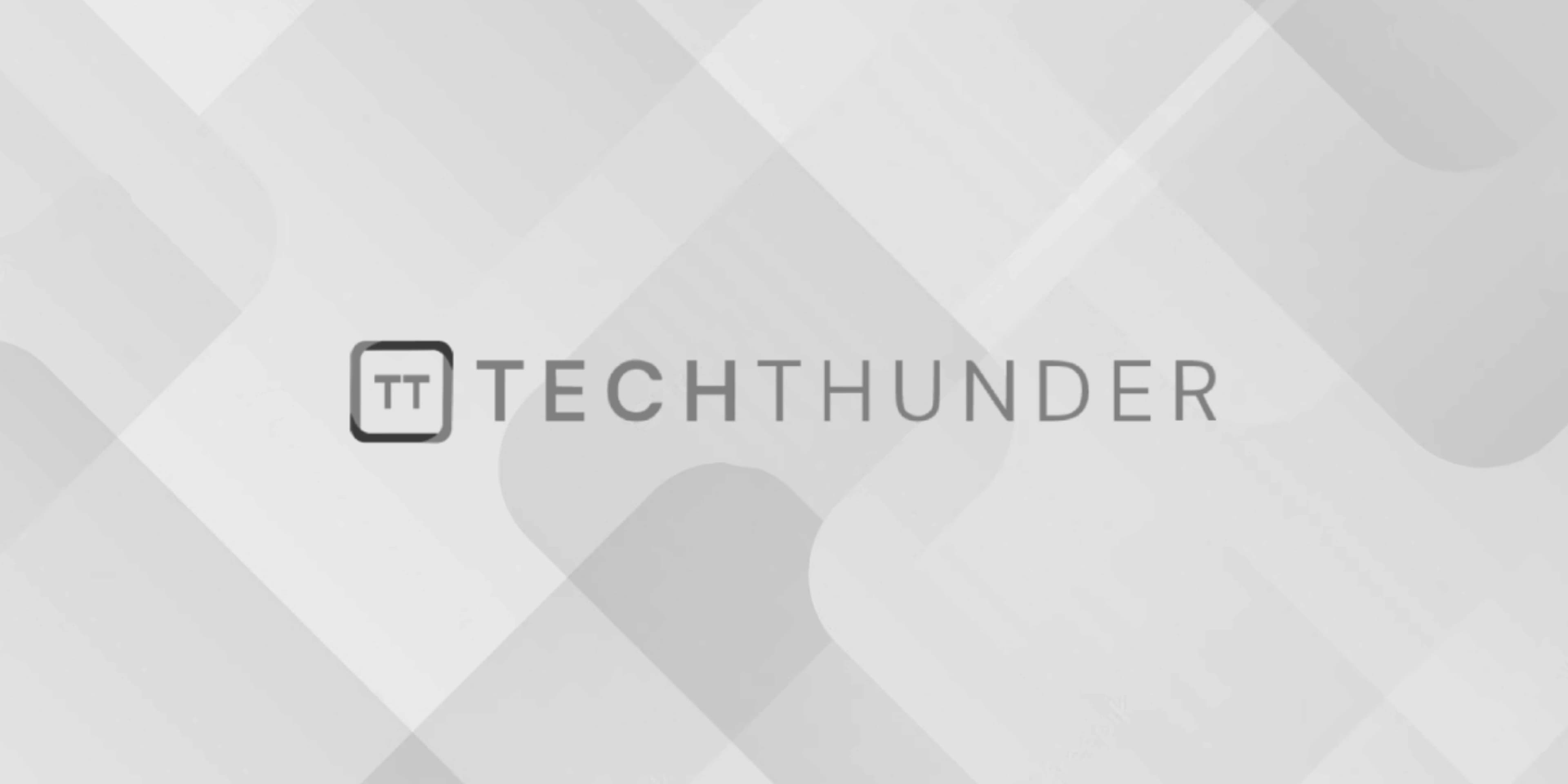
244 views
Relational Operator in C
The relational operators are used to compare two values and determine the relationship between them. These operators are often used in conditional statements and expressions to make decisions based on the comparison results. C provides the following relational operators:
- Equal to (
==
): Checks if two values are equal.
- Example:
x == y
returns true ifx
is equal toy
.
- Not equal to (
!=
): Checks if two values are not equal.
- Example:
x != y
returns true ifx
is not equal toy
.
- Greater than (
>
): Checks if the left operand is greater than the right operand.
- Example:
x > y
returns true ifx
is greater thany
.
- Less than (
<
): Checks if the left operand is less than the right operand.
- Example:
x < y
returns true ifx
is less thany
.
- Greater than or equal to (
>=
): Checks if the left operand is greater than or equal to the right operand.
- Example:
x >= y
returns true ifx
is greater than or equal toy
.
- Less than or equal to (
<=
): Checks if the left operand is less than or equal to the right operand.
- Example:
x <= y
returns true ifx
is less than or equal toy
.
These operators return a result of either true (1) or false (0) based on the comparison of the operands. Relational operators are commonly used in conditional statements, such as if
and while
statements, to control the flow of a program based on conditions.
Example of using relational operators in C:
C
#include <stdio.h>
int main() {
int a = 5;
int b = 10;
if (a == b) {
printf("a is equal to b.\n");
} else {
printf("a is not equal to b.\n");
}
if (a < b) {
printf("a is less than b.\n");
}
if (a <= b) {
printf("a is less than or equal to b.\n");
}
if (a != b) {
printf("a is not equal to b.\n");
}
return 0;
}
In this example, relational operators are used to compare the values of a
and b
, and appropriate messages are printed based on the comparison results.