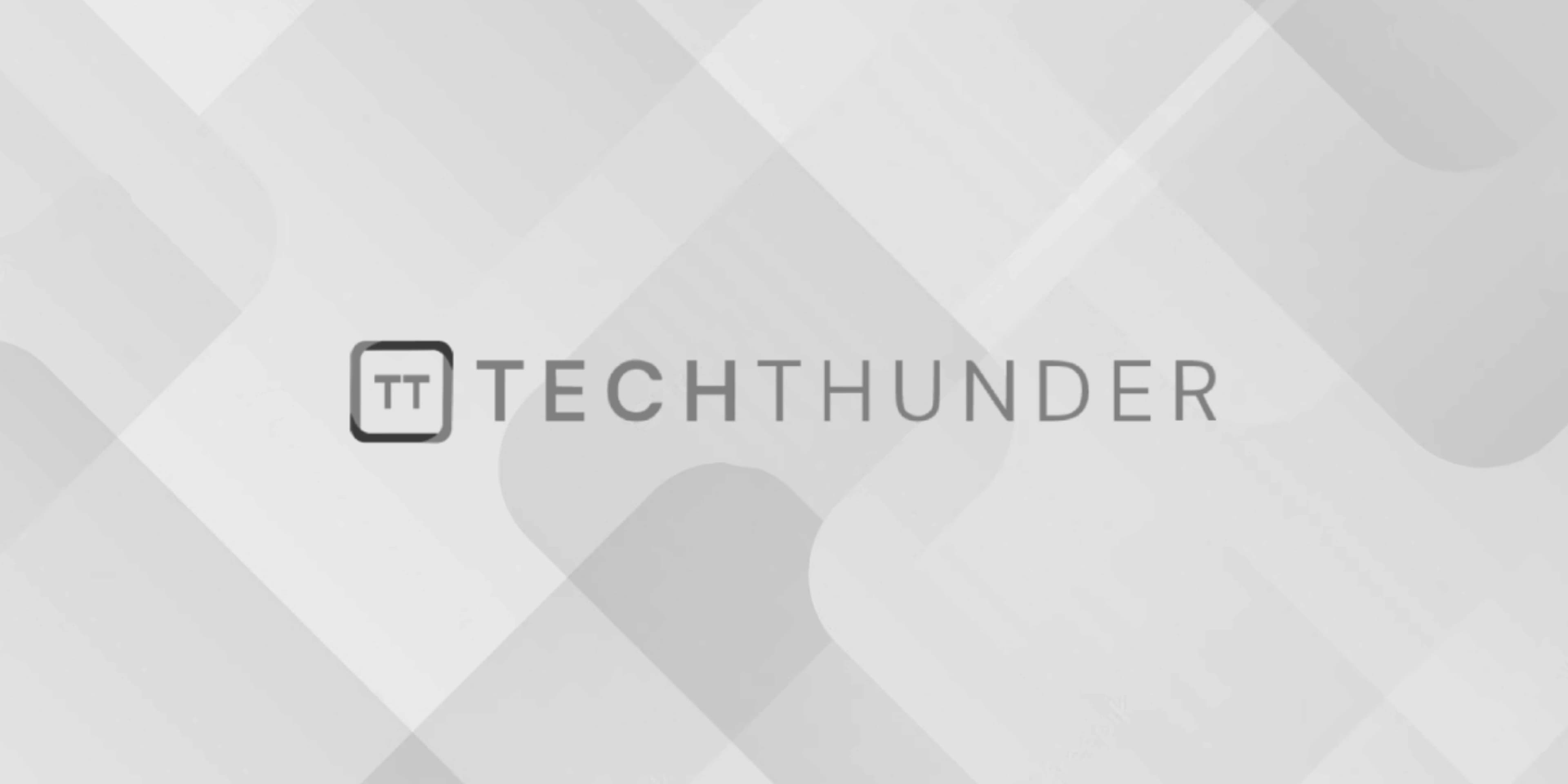
Structure Padding in C
Structure padding in C is a concept related to memory alignment. It refers to the practice of adding extra bytes (padding) between structure members to ensure that each member is properly aligned in memory, according to the hardware architecture’s requirements. Proper alignment can improve the efficiency of memory access and data transfer, especially on architectures where unaligned memory access is less efficient or even unsupported.
Here are some key points about structure padding in C:
- Data Alignment: Many computer architectures require that certain data types (e.g., integers, floats, pointers) be stored at memory addresses that are multiples of their size. For example, a 4-byte integer might need to be stored at a memory address that is a multiple of 4.
- Structure Members: When you define a C structure (struct), its members are laid out in memory in the order they are declared. The size and alignment of each member affect the overall size and alignment of the entire structure.
- Padding: To ensure proper alignment of structure members, the compiler may insert padding bytes between members. Padding bytes are added to make sure that each member starts at an appropriate memory address.
- Compiler-Dependent: The amount and placement of padding are compiler-dependent and can vary between different C compilers and even between different compiler settings. Therefore, you should not rely on a specific padding scheme if portability is a concern.
- #pragma pack: Some C compilers provide compiler-specific pragmas or directives to control structure packing. For example,
#pragma pack
can be used to control the alignment and padding of structure members. However, these directives may not be portable across different compilers. - Memory Efficiency: Structure padding can lead to memory inefficiency, especially when dealing with large structures. To minimize padding, you can manually reorder structure members or use compiler-specific directives, but this may compromise code readability and portability.
Here’s an example illustrating structure padding:
#include <stdio.h>
struct Example {
char a; // 1 byte
int b; // 4 bytes (padded to ensure proper alignment)
double c; // 8 bytes
};
int main() {
printf("Size of struct Example: %lu bytes\n", sizeof(struct Example));
return 0;
}
In this example, the struct Example
contains a char, an int, and a double. Due to alignment requirements, the compiler inserts padding between a
and b
to ensure that b
is properly aligned as a 4-byte integer. As a result, the size of the structure is likely to be larger than the sum of the sizes of its members due to the padding.
To minimize structure padding and memory overhead, you can rearrange the members manually or use compiler-specific pragmas or options to control the packing of structures. However, keep in mind that these approaches may not be portable across different compilers and architectures.