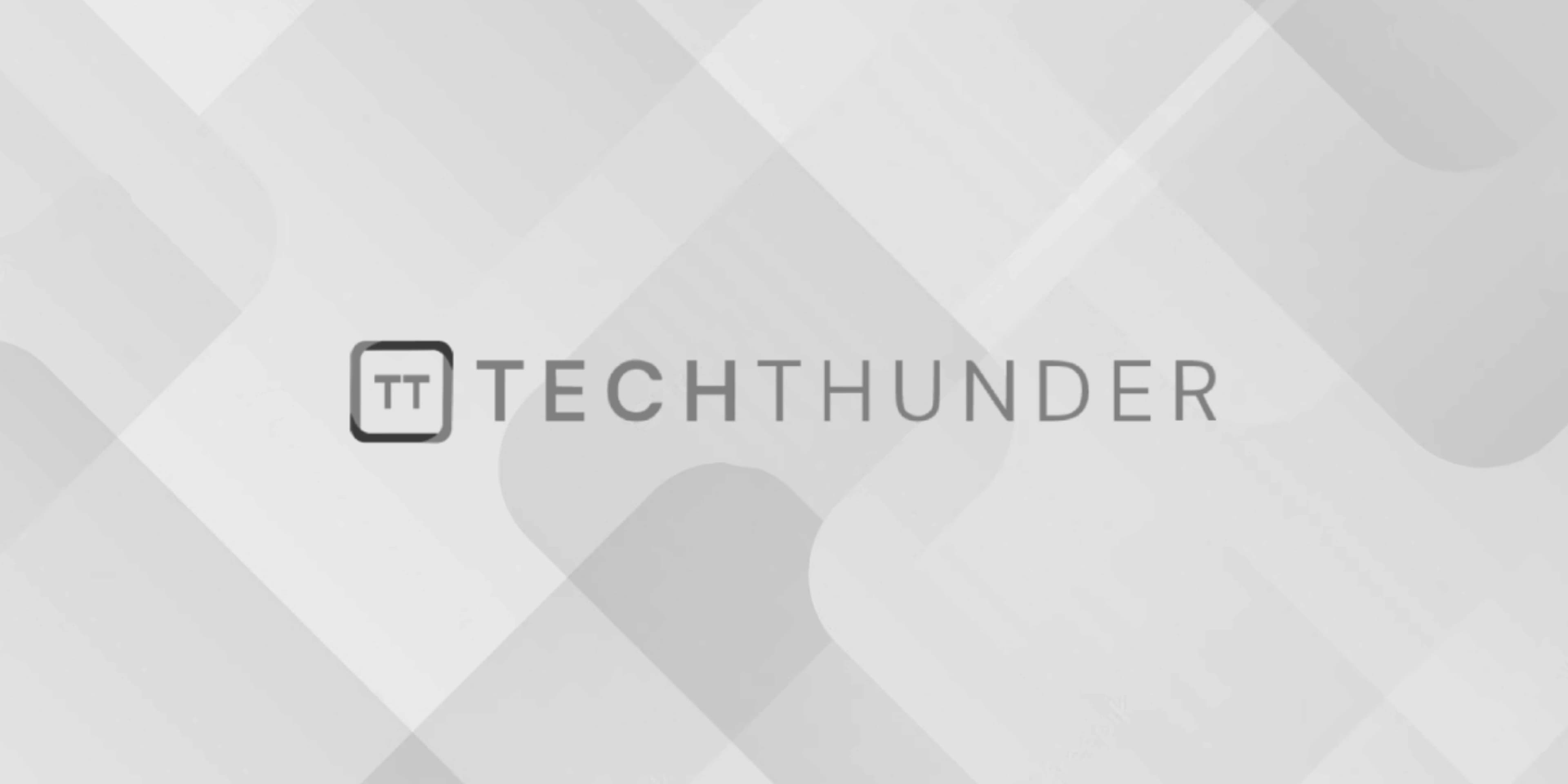
309 views
Pre-increment and Post-increment Operator in C
The both pre-increment and post-increment operators are used to increase the value of a variable by 1. However, they differ in how they modify the variable and when they return the value of the variable.
- Post-increment (
variable++
):
- The post-increment operator increases the value of the variable after it’s used in the current expression. It returns the current value and then increments the variable by 1.
- It’s also known as the “use and then increment” operator. Example:
C
int a = 5;
int b = a++`; // Use the current value of 'a' and then increment it
In this example, the value of b
will be 5 because a
is incremented after its current value is assigned to b
.
- Pre-increment (
++variable
):
- The pre-increment operator increases the value of the variable before it’s used in the current expression. It increments the variable by 1 and then returns the updated value.
- It’s also known as the “increment and then use” operator. Example:
C
int a = 5;
int b = ++a; // Increment 'a' first, then use its updated value
In this example, the value of b
will be 6 because a
is incremented before its value is assigned to b
.
Here’s a side-by-side comparison of pre-increment and post-increment:
C
int x = 5;
int y = 5;
int postResult = x++; // Use the current value of 'x' (5) and then increment it (x becomes 6)
int preResult = ++y; // Increment 'y' first (y becomes 6), then use its updated value
printf("Post-increment result: %d\n", postResult); // Post-increment result: 5
printf("Pre-increment result: %d\n", preResult); // Pre-increment result: 6
It’s important to understand the difference between pre-increment and post-increment, especially when they are used in expressions. Depending on whether you want to use the current value before or after incrementing, you should choose the appropriate operator.