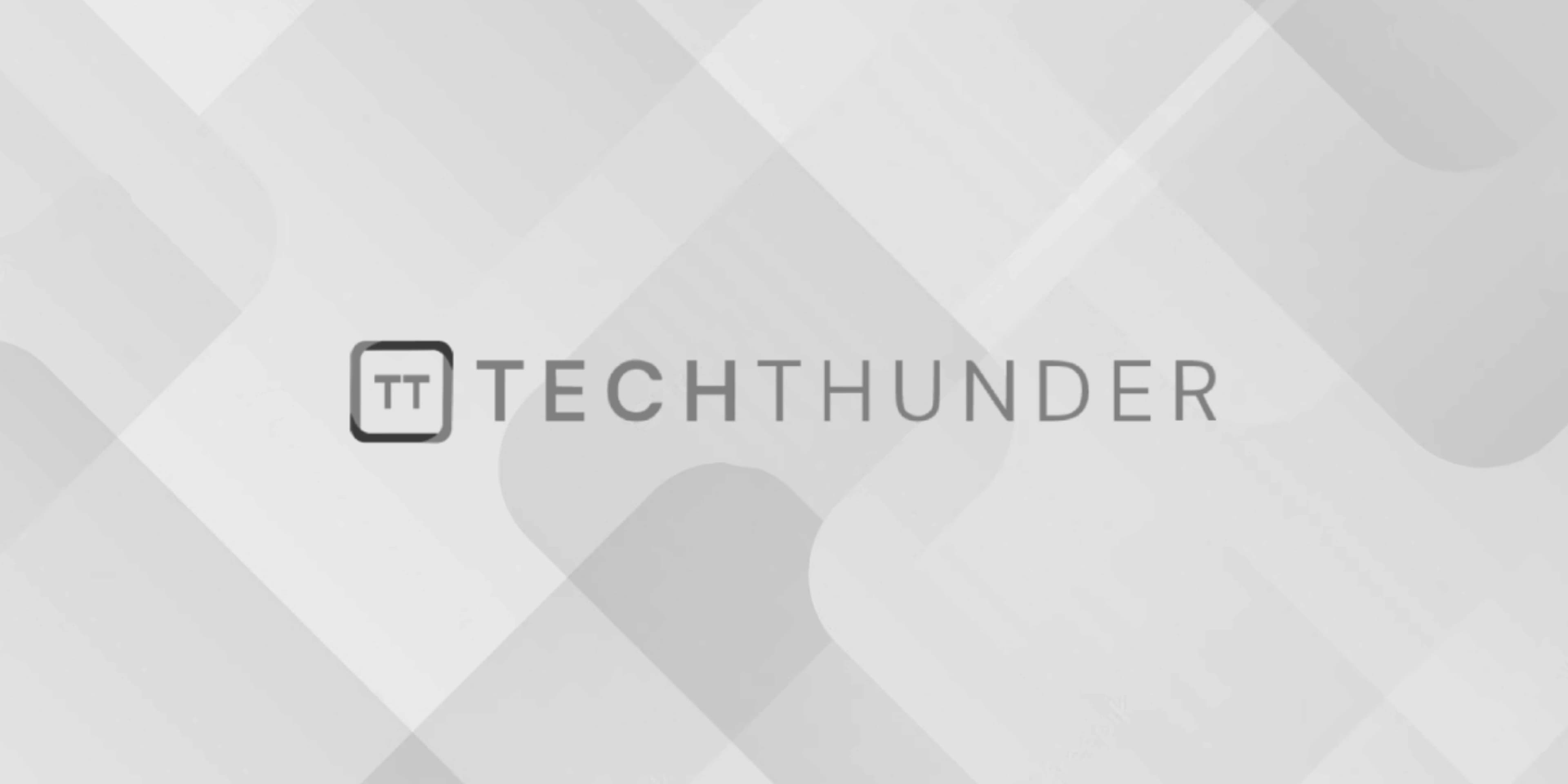
309 views
Sleeping Barber Problem Solution in C
The Sleeping Barber Problem is a classic synchronization problem that involves a barber, a waiting room with a limited number of chairs, and a number of customers. The problem is to coordinate the interactions between the barber and customers so that the barber serves customers in the waiting room while avoiding conflicts like racing conditions and deadlock. Below is a solution to the Sleeping Barber Problem in C using POSIX threads (pthread library).
C
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#define NUM_CHAIRS 5 // Number of chairs in the waiting room
pthread_mutex_t mutex;
pthread_cond_t barberReady, customerReady;
int numCustomers = 0;
int barberAvailable = 0;
void* barber(void* arg) {
while (1) {
pthread_mutex_lock(&mutex);
if (numCustomers == 0) {
printf("Barber is sleeping.\n");
barberAvailable = 1;
pthread_cond_wait(&customerReady, &mutex);
}
numCustomers--;
printf("Barber is cutting hair. Customers waiting: %d\n", numCustomers);
sleep(2); // Simulate hair-cutting
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&barberReady);
}
}
void* customer(void* arg) {
while (1) {
pthread_mutex_lock(&mutex);
if (numCustomers < NUM_CHAIRS) {
if (barberAvailable) {
barberAvailable = 0;
pthread_cond_signal(&customerReady);
}
numCustomers++;
printf("Customer arrived. Customers waiting: %d\n", numCustomers);
pthread_mutex_unlock(&mutex);
pthread_cond_wait(&barberReady, &mutex);
} else {
printf("Customer leaving, no chairs available.\n");
pthread_mutex_unlock(&mutex);
}
sleep(1); // Simulate time between customer arrivals
}
}
int main() {
pthread_t barberThread, customerThread;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&barberReady, NULL);
pthread_cond_init(&customerReady, NULL);
pthread_create(&barberThread, NULL, barber, NULL);
pthread_create(&customerThread, NULL, customer, NULL);
pthread_join(barberThread, NULL);
pthread_join(customerThread, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&barberReady);
pthread_cond_destroy(&customerReady);
return 0;
}
In this program:
- The barber and customer threads are created using
pthread_create
. pthread_mutex
is used to protect the shared variables and prevent race conditions.pthread_cond
variables (barberReady
andcustomerReady
) are used for signaling between the barber and customers.- The
barber
function simulates the barber’s behavior of cutting hair when a customer arrives. If no customers are present, the barber sleeps until a customer arrives. - The
customer
function simulates customers arriving at the barber shop. If there are empty chairs, the customer takes a seat. If all chairs are occupied, the customer leaves. - The program runs indefinitely, simulating barber and customer interactions. The barber serves one customer at a time, and customers arrive at random intervals.
Compile and run this program to observe the Sleeping Barber Problem in action.