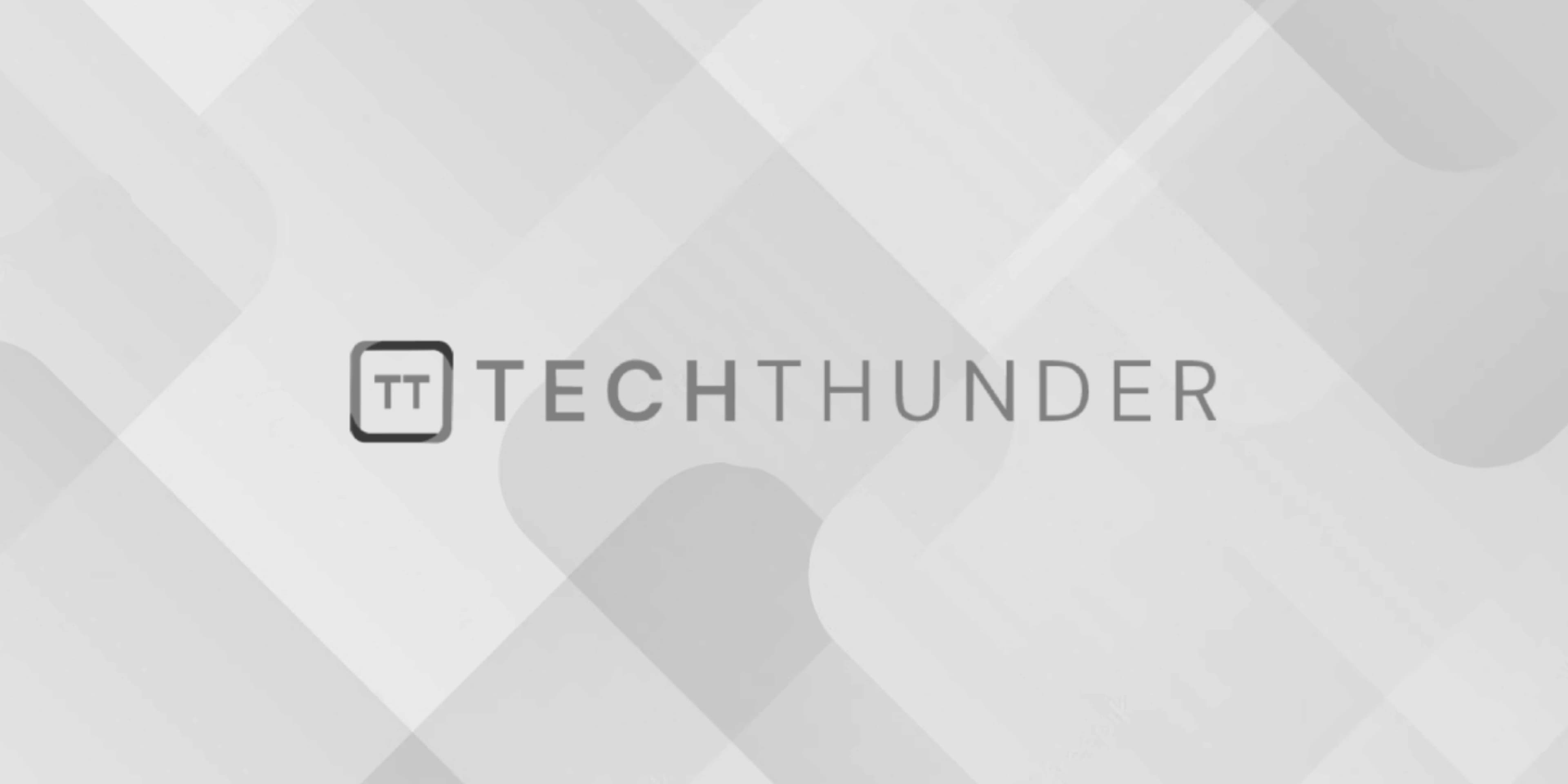
229 views
Composite Numbers List in C
The simple C program that generates a list of composite numbers within a specified range:
C
#include <stdio.h>
// Function to check if a number is composite
int isComposite(int num) {
if (num <= 1) {
return 0; // 0 and 1 are not composite
}
for (int i = 2; i * i <= num; ++i) {
if (num % i == 0) {
return 1; // Found a divisor other than 1 and itself
}
}
return 0; // No divisors found other than 1 and itself
}
int main() {
int lower, upper;
printf("Enter the lower and upper bounds: ");
scanf("%d %d", &lower, &upper);
printf("Composite numbers between %d and %d:\n", lower, upper);
for (int num = lower; num <= upper; ++num) {
if (isComposite(num)) {
printf("%d ", num);
}
}
printf("\n");
return 0;
}
In this program, the isComposite
function checks if a given number is composite by iterating from 2 up to the square root of the number. If the number is divisible by any smaller number, it’s considered composite. The main program takes the lower and upper bounds from the user and generates a list of composite numbers within that range.
Keep in mind that this is a basic implementation and might not be the most efficient for generating composite numbers for very large ranges. Also, ensure that you validate user input to ensure it’s within an appropriate range.