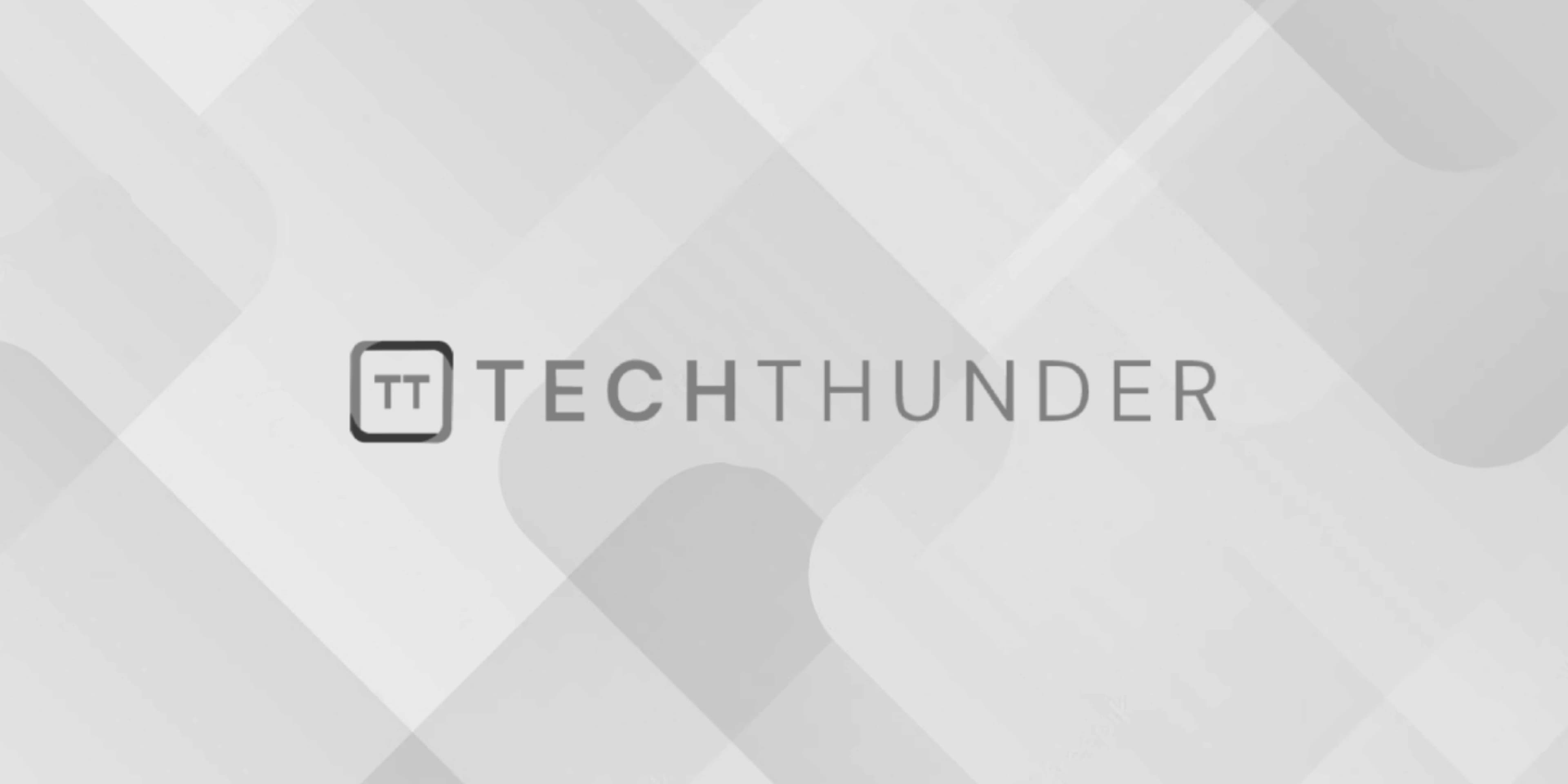
isalpha() function in C
The isalpha()
function is a library function declared in the <ctype.h>
header. It is used to determine whether a given character is an alphabetic character, which means it is a letter of the alphabet (either uppercase or lowercase).
Here’s the function signature:
int isalpha(int c);
c
: The integer representation of the character to be tested.
The function returns a non-zero (true) value if the character is an alphabetic character (a letter), and it returns 0 (false) if it’s not.
Here’s an example of how to use isalpha()
:
#include <stdio.h>
#include <ctype.h>
int main() {
char ch1 = 'A';
char ch2 = '7';
char ch3 = '$';
if (isalpha(ch1)) {
printf("%c is an alphabetic character.\n", ch1);
} else {
printf("%c is not an alphabetic character.\n", ch1);
}
if (isalpha(ch2)) {
printf("%c is an alphabetic character.\n", ch2);
} else {
printf("%c is not an alphabetic character.\n", ch2);
}
if (isalpha(ch3)) {
printf("%c is an alphabetic character.\n", ch3);
} else {
printf("%c is not an alphabetic character.\n", ch3);
}
return 0;
}
In this example, isalpha()
is used to check whether three characters (ch1
, ch2
, and ch3
) are alphabetic characters. The function returns true for 'A'
since it’s an alphabetic character, and it returns false for '7'
and '$'
since they are not alphabetic characters.
Output:
A is an alphabetic character.
7 is not an alphabetic character.
$ is not an alphabetic character.
You can use isalpha()
to validate and process input where you expect alphabetic characters or to filter out characters that don’t represent letters.