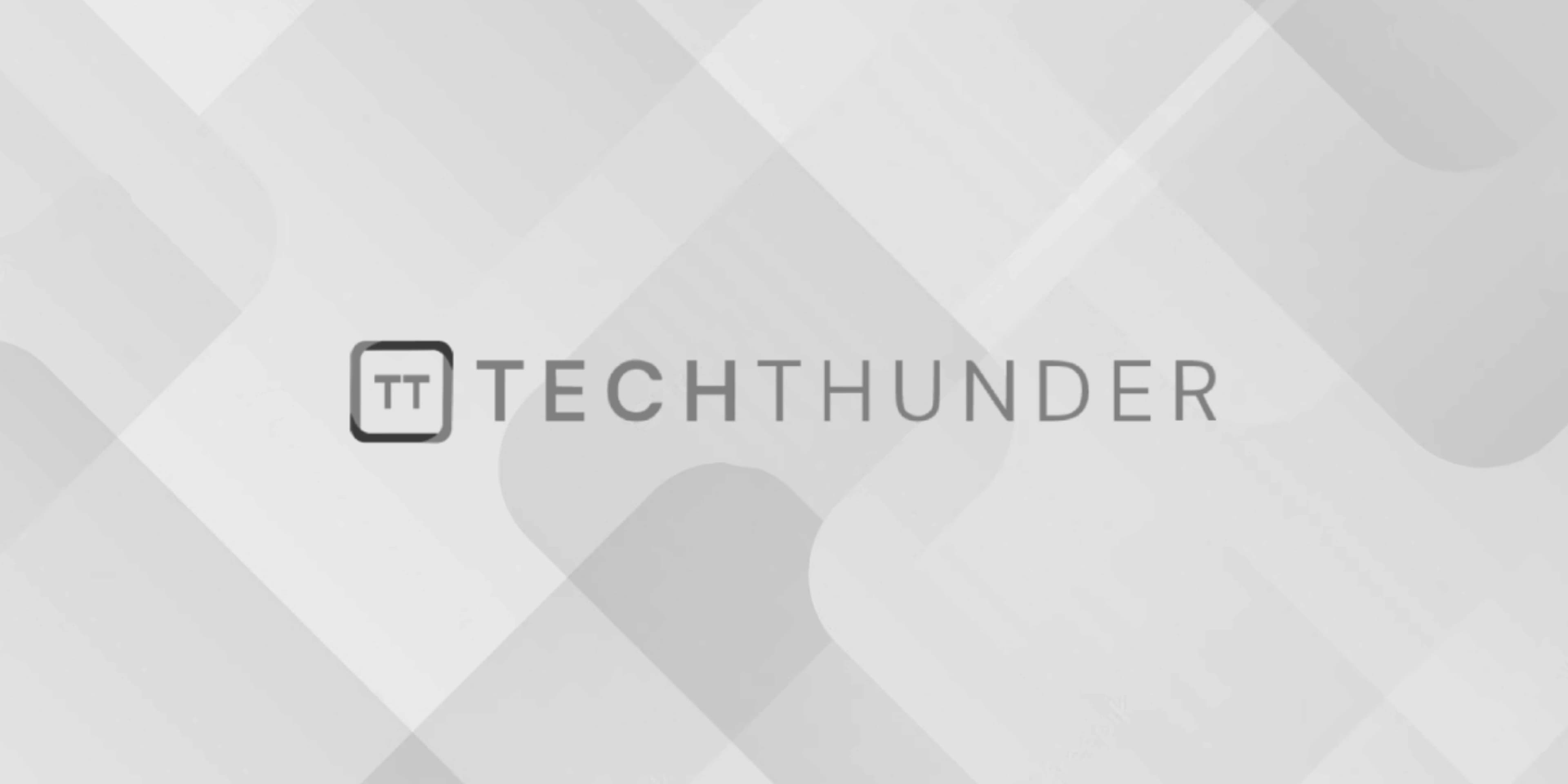
Memset C
The memset()
function is used to fill a block of memory with a specific byte value. This function is particularly useful for initializing arrays or setting a range of memory locations to a constant value. The memset()
function is part of the C Standard Library and is declared in the <string.h>
header.
Here’s the syntax of the memset()
function:
void *memset(void *ptr, int value, size_t num);
ptr
: A pointer to the memory block that you want to fill.value
: The byte value to be set. This should be an integer, usually in the range 0-255, which will be interpreted as an unsigned char and used to fill the memory.num
: The number of bytes to fill starting from the given pointer.
The memset()
function returns a pointer to the ptr
argument.
Here’s an example of how to use memset()
to initialize an array of integers to a specific value:
#include <stdio.h>
#include <string.h>
int main() {
int numbers[5];
// Initialize the array to 0 using memset
memset(numbers, 0, sizeof(numbers));
// Print the initialized array
for (int i = 0; i < 5; i++) {
printf("numbers[%d] = %d\n", i, numbers[i]);
}
return 0;
}
In this example:
- We include the
<string.h>
header to use thememset()
function. - We declare an integer array
numbers
with 5 elements. - We use
memset(numbers, 0, sizeof(numbers))
to initialize all elements of thenumbers
array to 0. Thesizeof(numbers)
calculates the total number of bytes to initialize based on the size of the array. - We print the initialized array to verify that all elements are set to 0.
Keep in mind that memset()
works with bytes, so the value
argument should be an integer value in the range 0-255. If you need to initialize a memory block with values other than 0, you should convert the desired byte value to the appropriate integer value.
Additionally, when using memset()
, be cautious to ensure that you don’t inadvertently overwrite memory beyond the intended block, as it can lead to undefined behavior.