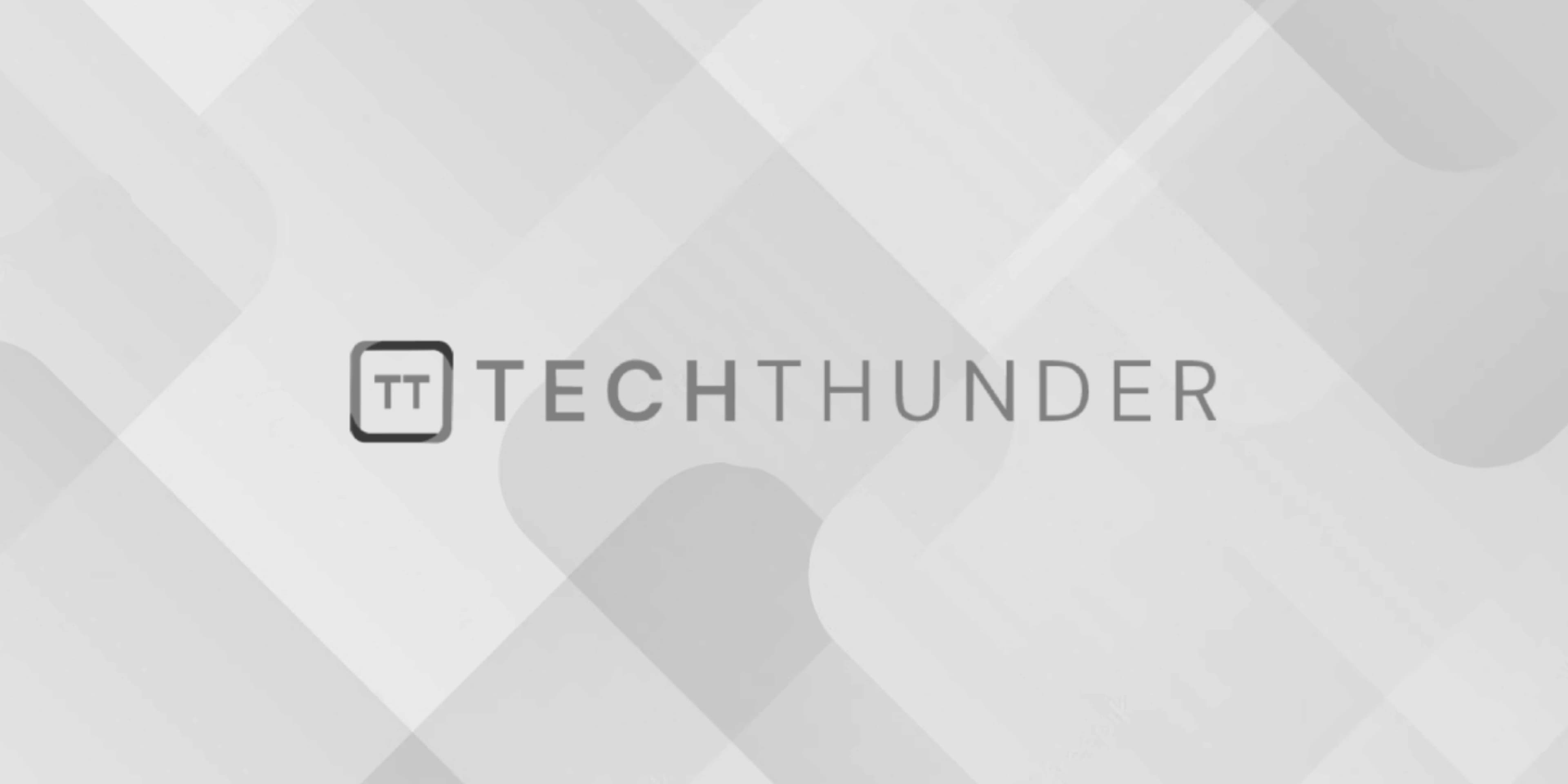
C strcmp()
The strcmp()
is a standard library function that is used to compare two strings. It’s part of the <string.h>
header and is commonly used for string comparison in C programs. strcmp()
compares two strings character by character and returns an integer value to indicate the result of the comparison. The possible return values are:
- 0: Indicates that the two strings are identical (i.e., they have the same content).
- Negative value: Indicates that the first string is lexicographically less than the second string.
- Positive value: Indicates that the first string is lexicographically greater than the second string.
Here is the syntax of strcmp()
:
int strcmp(const char *str1, const char *str2);
str1
andstr2
are pointers to the null-terminated strings you want to compare.
Here’s an example of using strcmp()
:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "apple";
char str2[] = "banana";
int result = strcmp(str1, str2);
if (result == 0) {
printf("The two strings are identical.\n");
} else if (result < 0) {
printf("str1 is lexicographically less than str2.\n");
} else {
printf("str1 is lexicographically greater than str2.\n");
}
return 0;
}
In this example, strcmp()
is used to compare two strings, “apple” and “banana.” The result is checked, and the appropriate message is printed based on the comparison result.
It’s important to note that strcmp()
compares strings lexicographically, which means it performs a character-by-character comparison based on the ASCII values of the characters. It stops comparing as soon as it encounters a difference or reaches the end of either string.
When using strcmp()
, be cautious about buffer overflows and ensure that the strings you are comparing are null-terminated. If you are comparing strings without null-terminators, you should use other functions like strncmp()
to specify the maximum number of characters to compare.