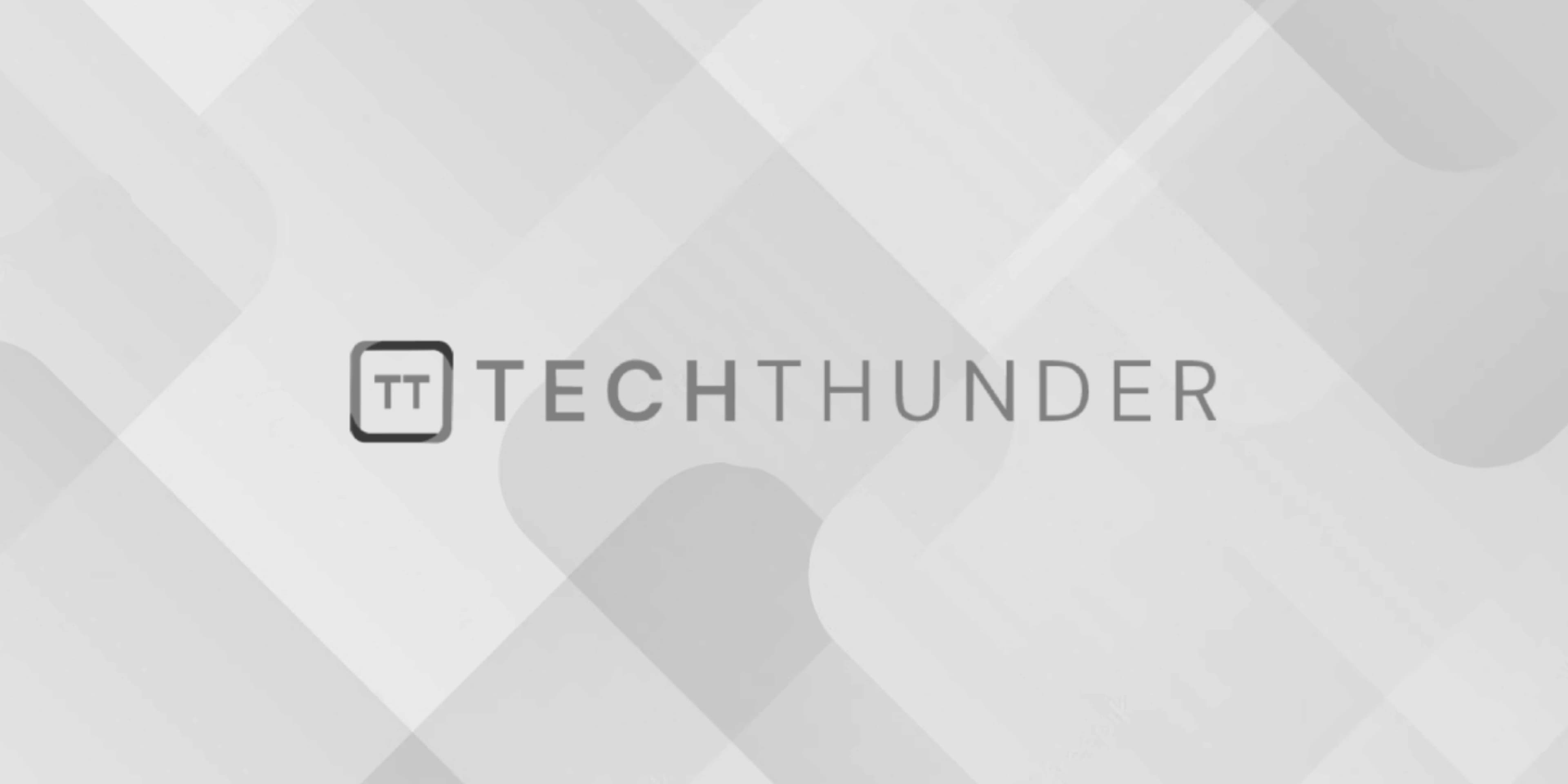
145 views
C Structure Test
The C can define and use structures to group related data elements together into a single composite data type. Here’s an example of how to define, create, and use structures in C:
C
#include <stdio.h>
#include <string.h>
// Define a structure named "Person"
struct Person {
char name[50];
int age;
};
int main() {
// Create an instance of the "Person" structure
struct Person person1;
// Initialize the structure fields
strcpy(person1.name, "John");
person1.age = 30;
// Access and print the structure fields
printf("Name: %s\n", person1.name);
printf("Age: %d\n", person1.age);
// You can also create an instance and initialize it directly
struct Person person2 = {"Alice", 25};
// Access and print the fields of the second structure
printf("Name: %s\n", person2.name);
printf("Age: %d\n", person2.age);
return 0;
}
In this example:
- We define a structure named “Person” using the
struct
keyword. It has two fields:name
(a character array) andage
(an integer). - In the
main()
function, we create two instances of the “Person” structure,person1
andperson2
. - We initialize the fields of
person1
andperson2
using thestrcpy()
function for the name field and direct assignment for the age field. - We access and print the fields of both
person1
andperson2
.
Structures are a fundamental way to organize and manage data in C, especially when you need to group multiple related pieces of information together into a single unit. You can define more complex structures with nested structures, arrays, and pointers as members, making them versatile for various data storage needs.