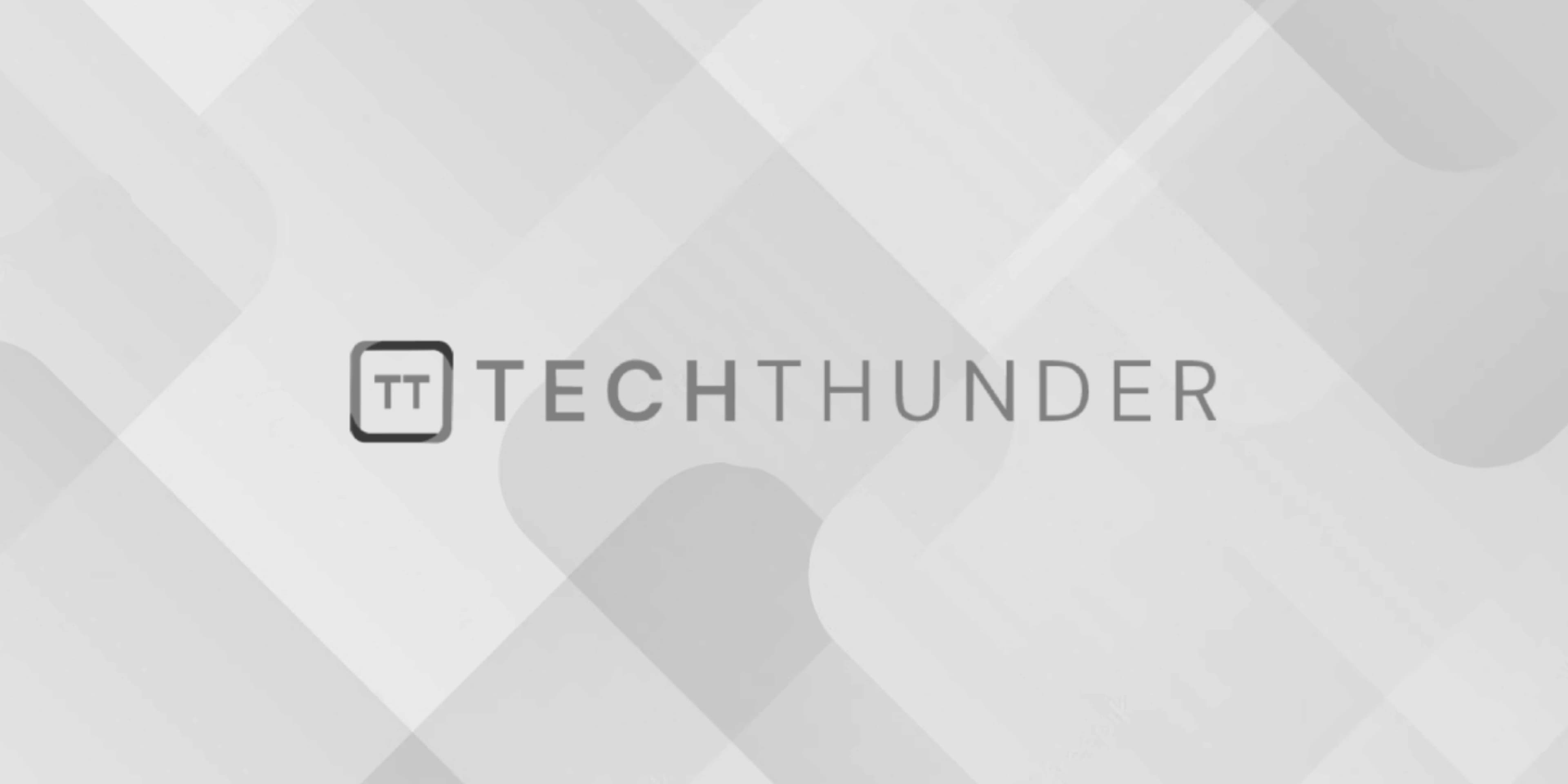
C #include
The #include
directive is used to include the contents of another file into your source code. This is commonly used for including header files that contain function prototypes, macro definitions, and other declarations. The #include
directive is processed by the C preprocessor, which combines the content of the specified file with your source code before compilation.
Here’s the basic syntax of the #include
directive:
#include <header_file.h>
<header_file.h>
is the name of the header file you want to include. It is typically enclosed in angle brackets (<
and>
) to indicate that it is a system header file or library header.
Alternatively, you can include header files using double quotes "
if they are located in the same directory as your source file or in a directory specified in your compiler’s search path:
#include "my_header.h"
Here’s a common example of using the #include
directive to include the standard input/output library’s header file:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
In this example:
#include <stdio.h>
includes the standard I/O header file, which provides theprintf
function prototype among other things.- The
printf
function is used to print “Hello, World!” to the standard output.
Including header files is essential for using functions, types, and macros defined in those headers. It allows you to reuse code, use library functions, and declare your own function prototypes to maintain code modularity and readability.
In addition to system and library header files, you can create your own header files to organize your code and declare custom functions and data structures. These header files are typically paired with implementation files (e.g., .c
files) to separate declarations from the actual code.