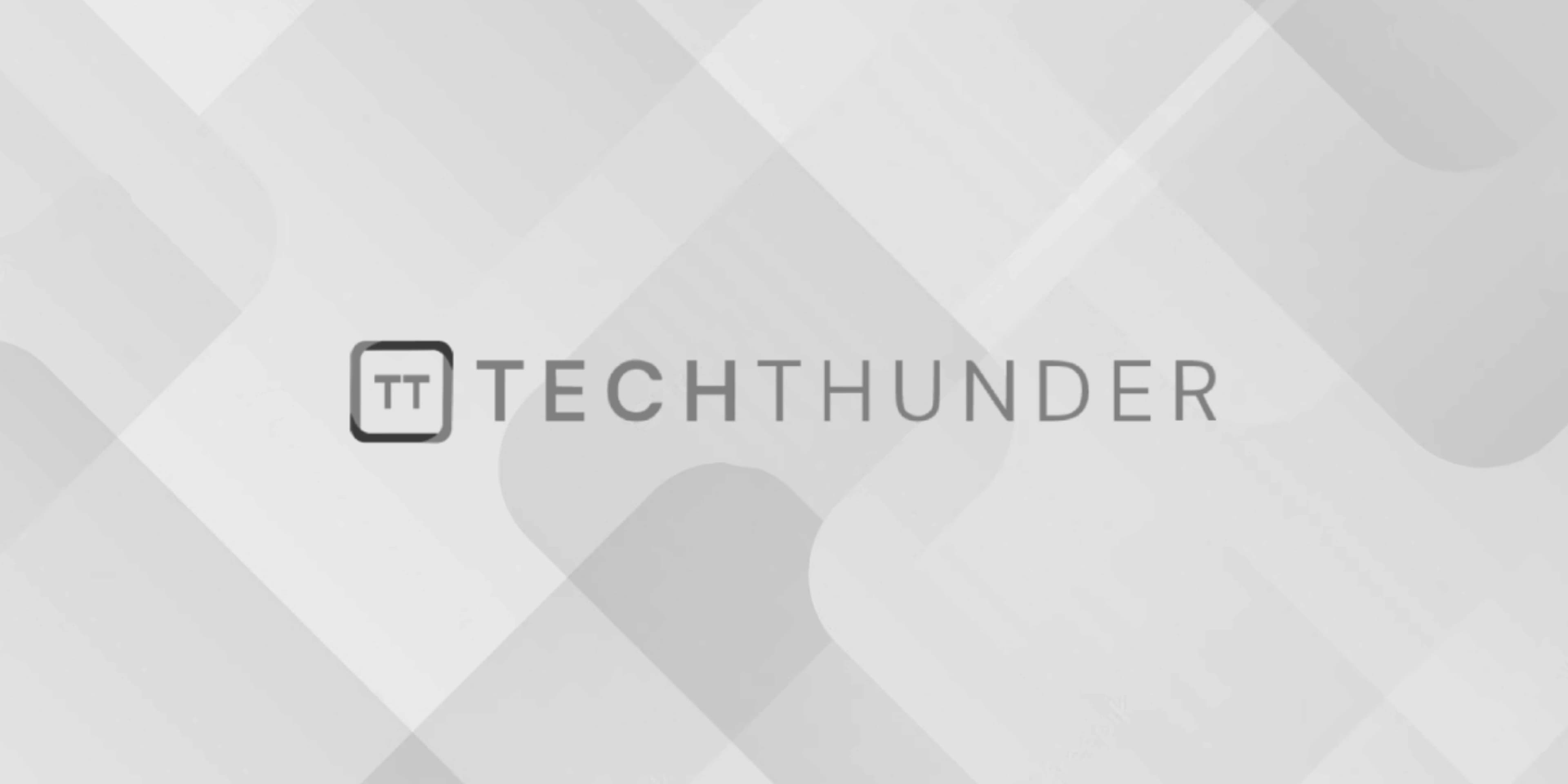
280 views
Twin Prime Numbers in C
Twin prime numbers are pairs of prime numbers that have a difference of 2. For example, (3, 5), (5, 7), and (11, 13) are twin prime pairs because the difference between each pair is 2.
To find twin prime numbers in C, you can write a program that checks if two consecutive numbers are prime and have a difference of 2. Here’s an example program to find twin prime numbers within a specified range:
C
#include <stdio.h>
#include <stdbool.h>
// Function to check if a number is prime
bool isPrime(int n) {
if (n <= 1) {
return false;
}
if (n <= 3) {
return true;
}
if (n % 2 == 0 || n % 3 == 0) {
return false;
}
for (int i = 5; i * i <= n; i += 6) {
if (n % i == 0 || n % (i + 2) == 0) {
return false;
}
}
return true;
}
int main() {
int lowerLimit, upperLimit;
printf("Enter the lower limit: ");
scanf("%d", &lowerLimit);
printf("Enter the upper limit: ");
scanf("%d", &upperLimit);
printf("Twin prime numbers between %d and %d are:\n", lowerLimit, upperLimit);
for (int i = lowerLimit; i <= upperLimit - 2; i++) {
if (isPrime(i) && isPrime(i + 2)) {
printf("(%d, %d)\n", i, i + 2);
}
}
return 0;
}
In this program:
- We define a
isPrime
function to check if a given number is prime. This function is used to determine whether two consecutive numbers are prime. - In the
main
function, we take input for the lower and upper limits of the range within which we want to find twin prime numbers. - We then loop through the range from
lowerLimit
toupperLimit - 2
, checking for twin prime pairs (i, i + 2) and printing them if both numbers are prime. - The
isPrime
function uses a primality test to determine if a number is prime. It’s more efficient than checking divisibility by all numbers up to the square root of n.
When you run this program and input the lower and upper limits, it will display the twin prime pairs within the specified range.