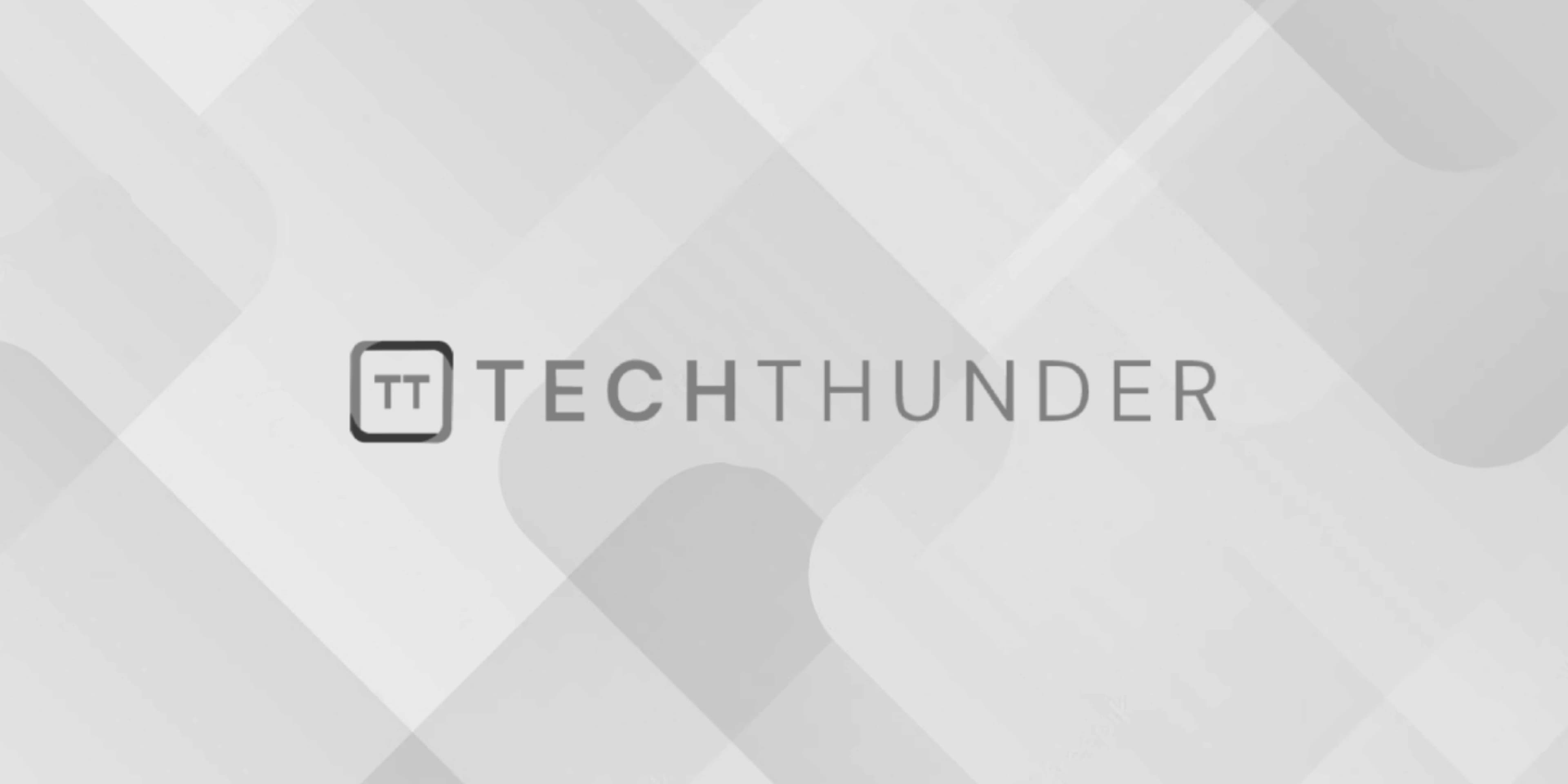
173 views
C fputc() fgetc()
The fputc()
and fgetc()
are file input/output functions used to write and read individual characters to and from a file, respectively. These functions work with character-oriented input and output, allowing you to handle text files character by character.
Here’s a brief explanation of fputc()
and fgetc()
:
fputc()
Function:
- The
fputc()
function is used to write a single character to a file. - It takes two arguments: the character to be written and a pointer to the
FILE
structure representing the file. - Example:
C
c FILE *file = fopen("example.txt", "w");
if (file != NULL) {
fputc('A', file); // Write the character 'A' to the file
fclose(file);
}
fgetc()
Function:
- The
fgetc()
function is used to read a single character from a file. - It takes a single argument: a pointer to the
FILE
structure representing the file from which you want to read. fgetc()
returns an integer representing the character read, orEOF
(End-of-File) if the end of the file is reached or an error occurs.- Example:
C
c FILE *file = fopen("example.txt", "r");
if (file != NULL) {
int ch = fgetc(file); // Read a character from the file
if (ch != EOF) {
printf("Character read from the file: %c\n", ch);
}
fclose(file);
}
Both functions are typically used within a loop to process characters one by one. Here’s a simple example that demonstrates writing and reading characters using fputc()
and fgetc()
:
C
#include <stdio.h>
int main() {
FILE *file = fopen("example.txt", "w");
if (file != NULL) {
fputc('A', file);
fputc('B', file);
fputc('C', file);
fclose(file);
}
file = fopen("example.txt", "r");
if (file != NULL) {
int ch;
printf("Characters read from the file: ");
while ((ch = fgetc(file)) != EOF) {
printf("%c ", ch);
}
fclose(file);
}
return 0;
}
In this example, the program writes the characters ‘A,’ ‘B,’ and ‘C’ to the file and then reads and prints them one by one using fgetc()
.