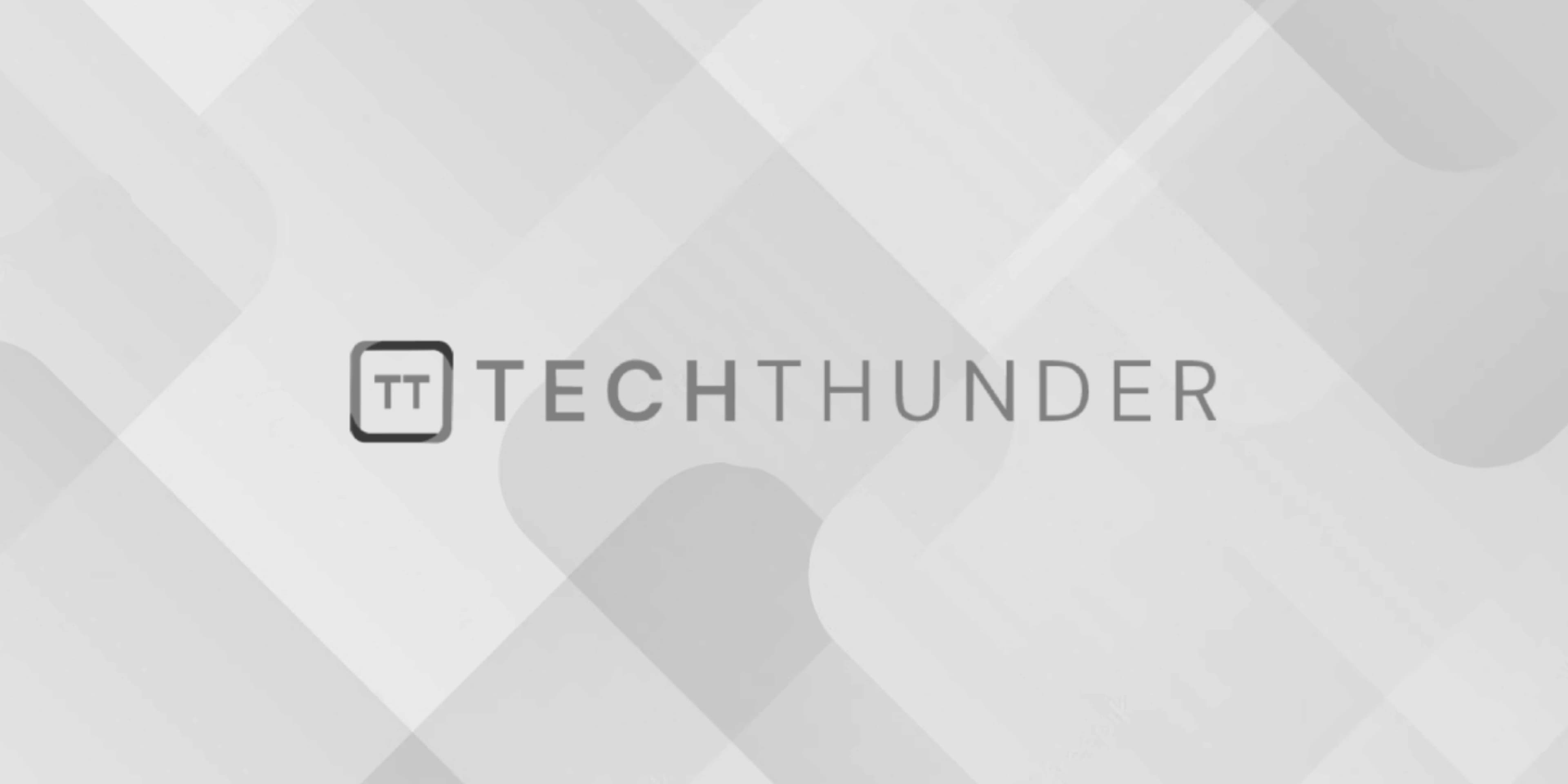
89 views
Find Median of 1D Array Using Functions in C
To find the median of a one-dimensional (1D) array in C using functions, you can follow these steps:
- Create a function to calculate the median.
- Sort the array in ascending order.
- If the array has an odd number of elements, return the middle element as the median.
- If the array has an even number of elements, return the average of the two middle elements.
Here’s an example of how to do this:
C
#include <stdio.h>
#include <stdlib.h>
// Function to compare two integers for sorting
int compareIntegers(const void *a, const void *b) {
return (*(int *)a - *(int *)b);
}
// Function to calculate the median of an array
double findMedian(int arr[], int size) {
// Sort the array in ascending order
qsort(arr, size, sizeof(int), compareIntegers);
// If the array has an odd number of elements, return the middle element
if (size % 2 != 0) {
return (double)arr[size / 2];
}
// If the array has an even number of elements, return the average of the two middle elements
else {
int mid1 = arr[(size - 1) / 2];
int mid2 = arr[size / 2];
return ((double)mid1 + mid2) / 2.0;
}
}
int main() {
int size;
printf("Enter the size of the array: ");
scanf("%d", &size);
if (size <= 0) {
printf("Invalid array size.\n");
return 1;
}
int arr[size];
printf("Enter %d elements:\n", size);
for (int i = 0; i < size; i++) {
scanf("%d", &arr[i]);
}
double median = findMedian(arr, size);
printf("Median of the array: %.2f\n", median);
return 0;
}
In this program:
- We use the
qsort()
function from thestdlib.h
library to sort the array in ascending order. To useqsort()
, we provide a comparison functioncompareIntegers
to specify how the elements should be compared during sorting. - The
findMedian
function calculates the median based on the sorted array. If the array has an odd number of elements, it returns the middle element. If the array has an even number of elements, it returns the average of the two middle elements. - In the
main
function, we input the size and elements of the array, callfindMedian
to calculate the median, and then print the result.
This program allows you to find the median of a 1D array of integers using functions.