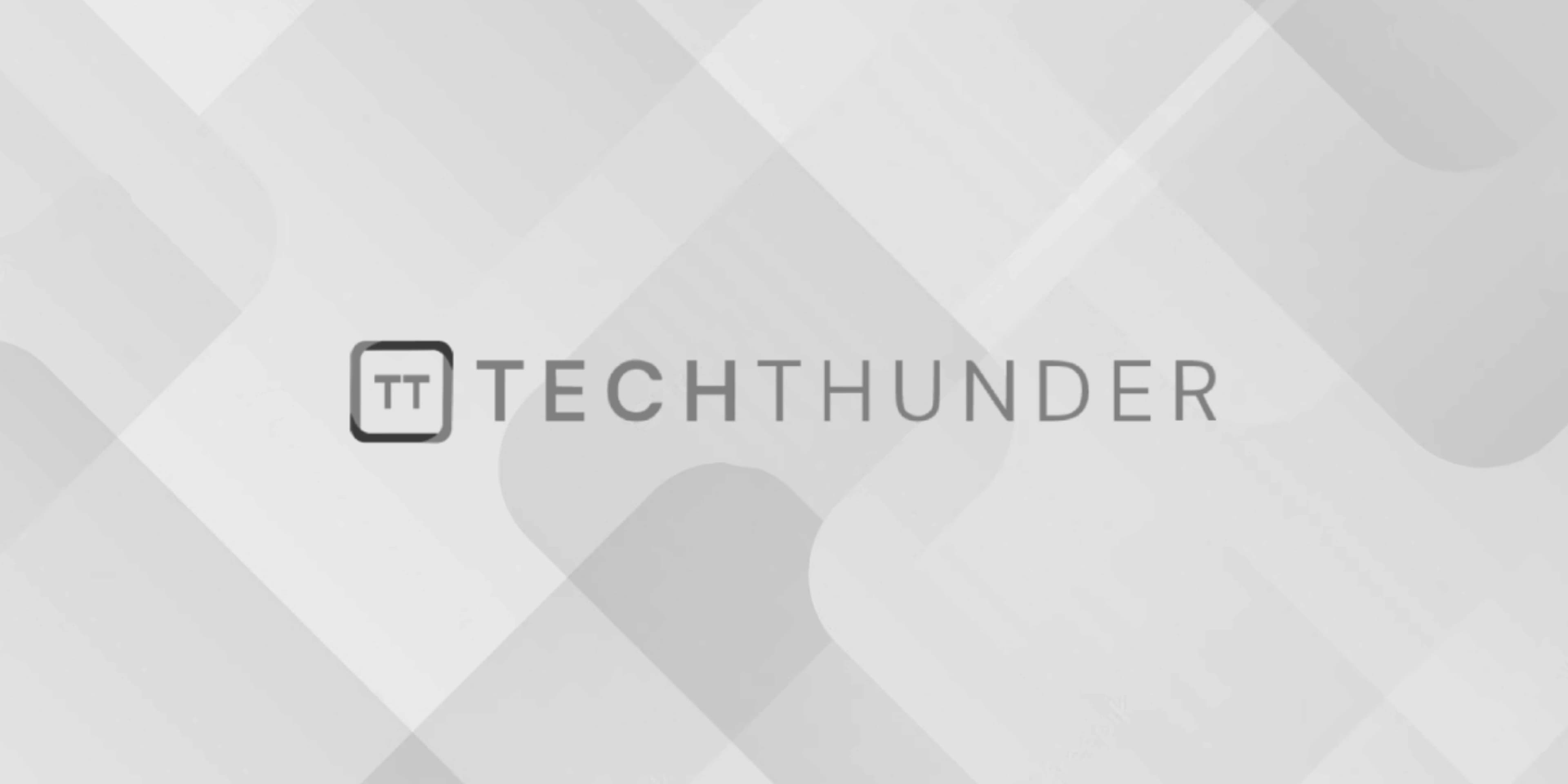
void pointer in C
The void
pointer, often denoted as void *
, is a special type of pointer that can point to objects of any data type. It is a generic or untyped pointer because it does not have a specific data type associated with it. void *
is commonly used in situations where you need to work with data of unknown type or when you want to create more flexible and generic functions.
Here are some key points about void
pointers in C:
- Declaration: You declare a
void
pointer like this:
void *ptr;
This declares a ptr
that can point to objects of any data type.
- Pointer Assignment: You can assign a
void
pointer to the address of any data object, regardless of its type:
int x = 42;
float y = 3.14;
void *ptr1 = &x; // Pointing to an integer
void *ptr2 = &y; // Pointing to a float
Here, ptr1
and ptr2
are void
pointers pointing to variables of different types.
- Pointer Arithmetic: You cannot directly perform pointer arithmetic with
void
pointers because the compiler doesn’t know the size of the data being pointed to. To perform pointer arithmetic, you must first cast thevoid
pointer to a specific pointer type:
int *intPtr = (int *)ptr1; // Casting void pointer to int pointer
Now, intPtr
is an int
pointer and can be used for pointer arithmetic.
- Dereferencing: You cannot directly dereference a
void
pointer because the compiler doesn’t know the data type. To access the value, you must cast it to the appropriate pointer type before dereferencing:
int x = *(int *)ptr1; // Casting and dereferencing a void pointer
This casts ptr1
to an int
pointer and then dereferences it to access the integer value.
- Generic Functions:
void
pointers are often used to create generic functions that can work with data of different types. For example, you can create a generic sorting function that takes avoid
pointer to an array and sorts it without knowing the specific data type of the elements.
void genericSort(void *array, size_t size, size_t elemSize,
int (*compare)(const void *, const void *)) {
// Sort the array using the provided comparison function
// elemSize is used for element size in bytes
}
Such functions can work with arrays of any data type.
void
pointers are a powerful feature in C, but they come with the responsibility of casting and interpreting the data correctly. Incorrect use of void
pointers can lead to type-related errors and undefined behavior. When working with void
pointers, it’s essential to ensure that you cast them to the appropriate data type before dereferencing or performing pointer arithmetic to avoid such issues.